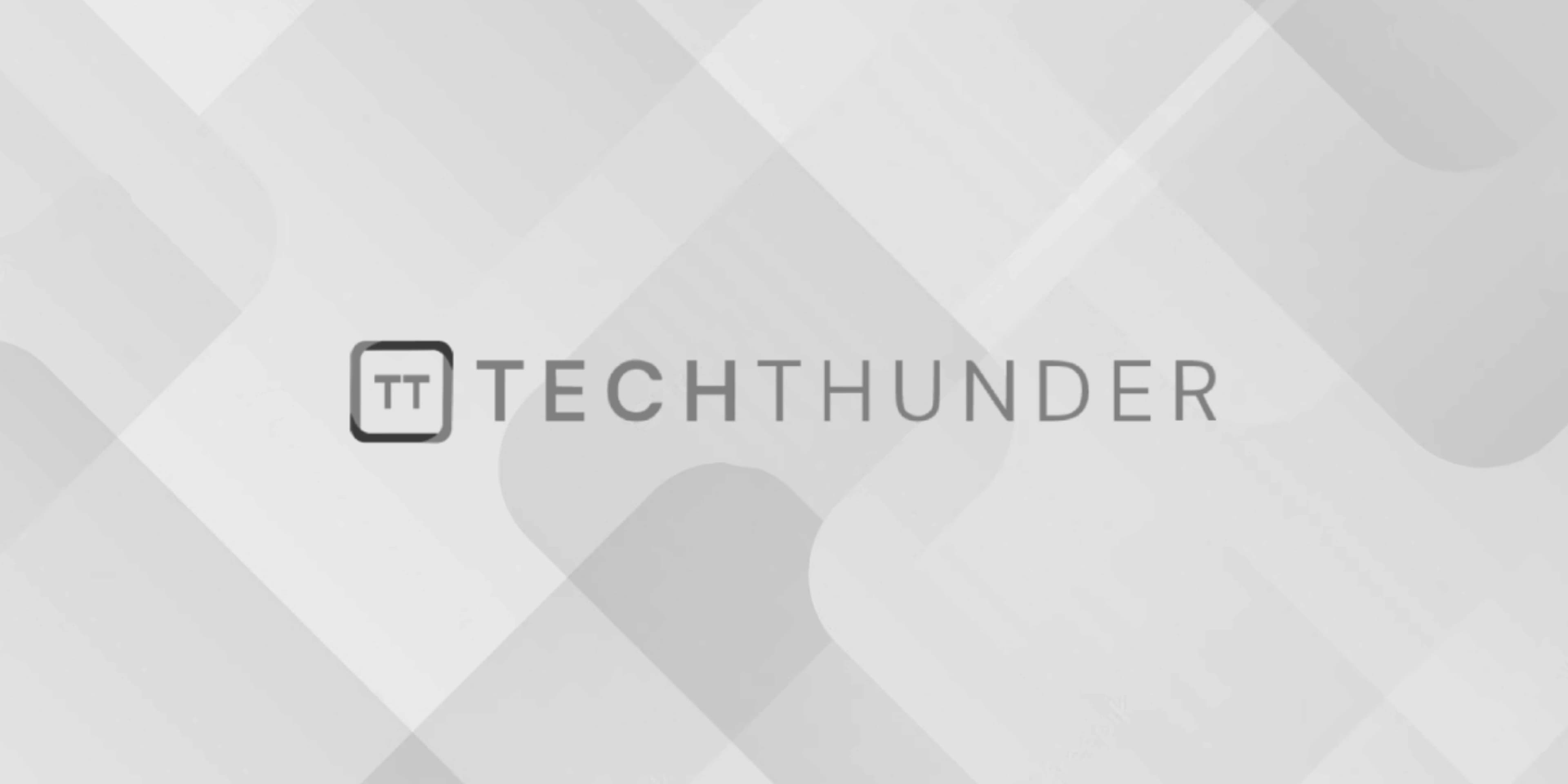
155 views
Inline Style in ReactJS
The inline styles directly to JSX elements using JavaScript objects. Inline styles are useful when you want to apply dynamic or component-specific styles. Here’s how you can use inline styles in React:
- Define the Inline Style Object: Create a JavaScript object that defines the styles you want to apply. Each CSS property should be camelCased (e.g.,
backgroundColor
instead ofbackground-color
). The property values can be strings or expressions that evaluate to valid CSS values.
TypeScript
const myStyle = {
color: 'blue',
backgroundColor: 'lightgray',
fontSize: '16px',
padding: '10px',
};
- Apply the Inline Style: Use the
style
attribute within your JSX element and assign the style object you created.
TypeScript
function MyComponent() {
const myStyle = {
color: 'blue',
backgroundColor: 'lightgray',
fontSize: '16px',
padding: '10px',
};
return (
<div style={myStyle}>
This is a component with inline styles.
</div>
);
}
You can apply inline styles to any JSX element, including divs, headings, buttons, and more.
- Dynamic Styles: Inline styles are useful for dynamically changing styles based on component state or props. You can conditionally apply styles by constructing the style object based on dynamic values.
TypeScript
function MyComponent({ isError }) {
const dynamicStyle = {
color: isError ? 'red' : 'green',
fontWeight: isError ? 'bold' : 'normal',
};
return (
<p style={dynamicStyle}>
{isError ? 'Error Message' : 'Success Message'}
</p>
);
}
In this example, the style depends on the isError
prop.
- Using Variables: You can use variables or computed values within your style object to make it more dynamic.
TypeScript
function MyComponent({ width }) {
const customWidth = width || '100px'; // Use a default width if not provided
const myStyle = {
width: customWidth,
backgroundColor: 'lightgray',
padding: '10px',
};
return (
<div style={myStyle}>
This element has a dynamic width.
</div>
);
}
Here, the customWidth
variable is used to determine the element’s width.
Remember that inline styles can become cumbersome when dealing with complex styles or animations. In such cases, it’s often more maintainable to use CSS classes or CSS-in-JS libraries like styled-components or Emotion. However, inline styles are a handy option for small, component-specific styling needs in React.