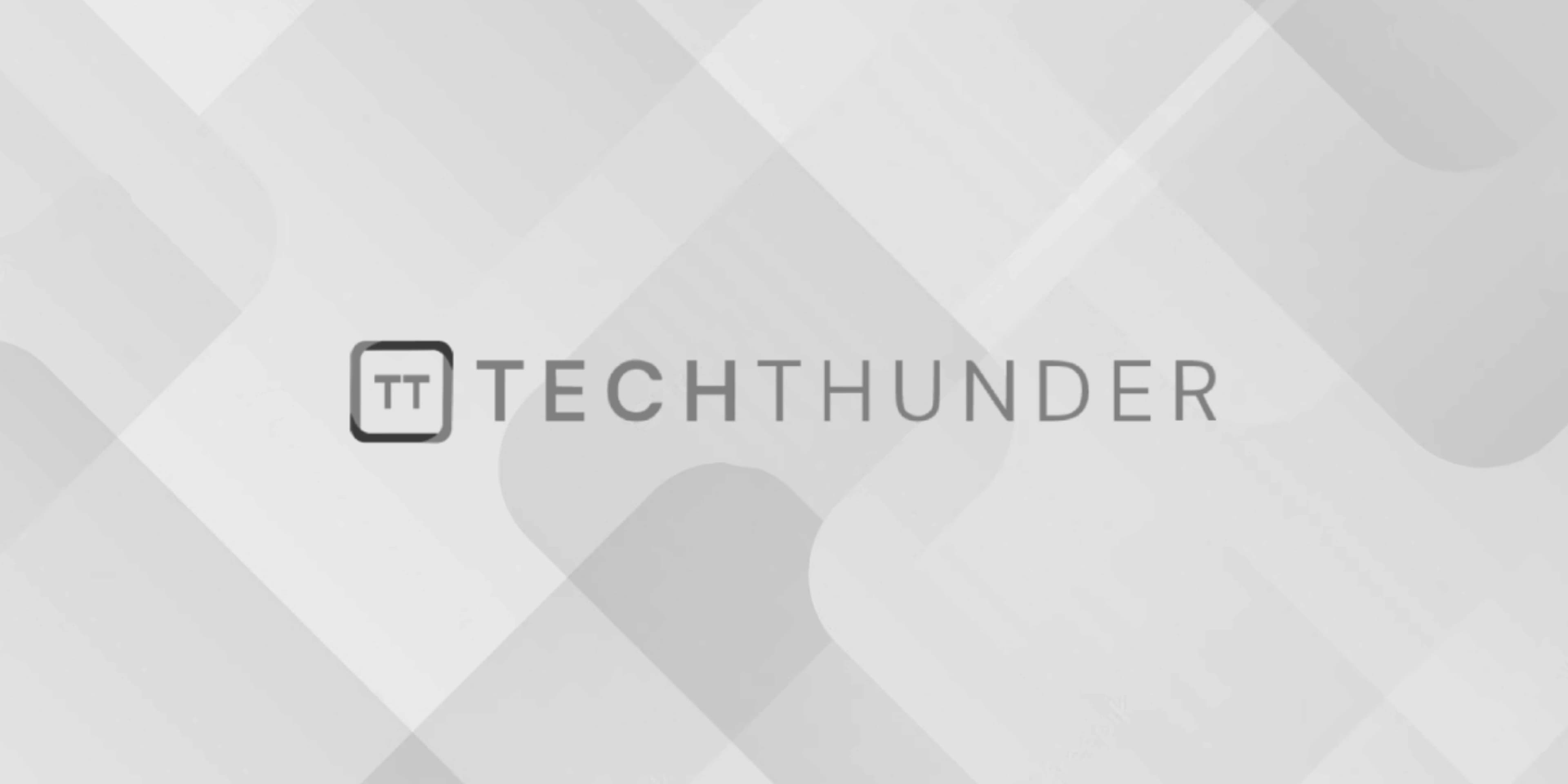
ReactJS Forms
The ReactJS, working with forms involves handling user input and managing the state of form elements. React provides a straightforward way to manage form data using component state. Here are the key steps for working with forms in React:
1. Creating a Form Component:
You can create a form component in React, which will render the form elements and manage their state.
import React, { Component } from 'react';
class MyForm extends Component {
constructor(props) {
super(props);
this.state = {
inputValue: '',
};
}
render() {
return (
<form>
{/* Form elements go here */}
</form>
);
}
}
export default MyForm;
2. Handling Input Elements:
To capture user input, you can add controlled form elements that are tied to the component’s state. For example, for a text input field:
<input
type="text"
value={this.state.inputValue}
onChange={(e) => this.setState({ inputValue: e.target.value })}
/>
Here, the value
attribute is set to the component’s state (inputValue
), and the onChange
event handler updates the state as the user types.
3. Handling Form Submission:
You can add a submit button to the form and handle its click event to submit the form data.
<form onSubmit={this.handleSubmit}>
<input
type="text"
value={this.state.inputValue}
onChange={(e) => this.setState({ inputValue: e.target.value })}
/>
<button type="submit">Submit</button>
</form>
In the form’s onSubmit
handler, you can prevent the default form submission behavior and handle the data as needed.
handleSubmit = (e) => {
e.preventDefault();
// Access form data using this.state.inputValue
console.log('Form submitted with value:', this.state.inputValue);
};
4. Validating Form Input:
You can implement form validation by adding conditional logic within the onChange
handler or the handleSubmit
method. For example, checking if the input is not empty:
handleChange = (e) => {
const inputValue = e.target.value;
if (inputValue.trim() === '') {
// Input is empty; show an error message or set an error state.
} else {
// Input is valid; update the state.
this.setState({ inputValue });
}
};
5. Handling Different Form Elements:
React can be used with various form elements such as text inputs, radio buttons, checkboxes, select elements, and more. You’ll handle each element type’s state and events based on their specific requirements.
6. Controlled vs. Uncontrolled Components:
React supports both controlled and uncontrolled form components. In controlled components, form elements are bound to React state, as shown in the examples above. In uncontrolled components, you rely on refs to access form element values directly from the DOM.
7. Libraries for Form Handling:
For complex forms and validation, you might consider using third-party libraries like Formik, Redux-Form, or React Hook Form. These libraries provide advanced features for form management and validation.
Remember that form handling in React is mainly about managing component state and using event handlers. By following these steps and principles, you can create robust and interactive forms in your React applications.