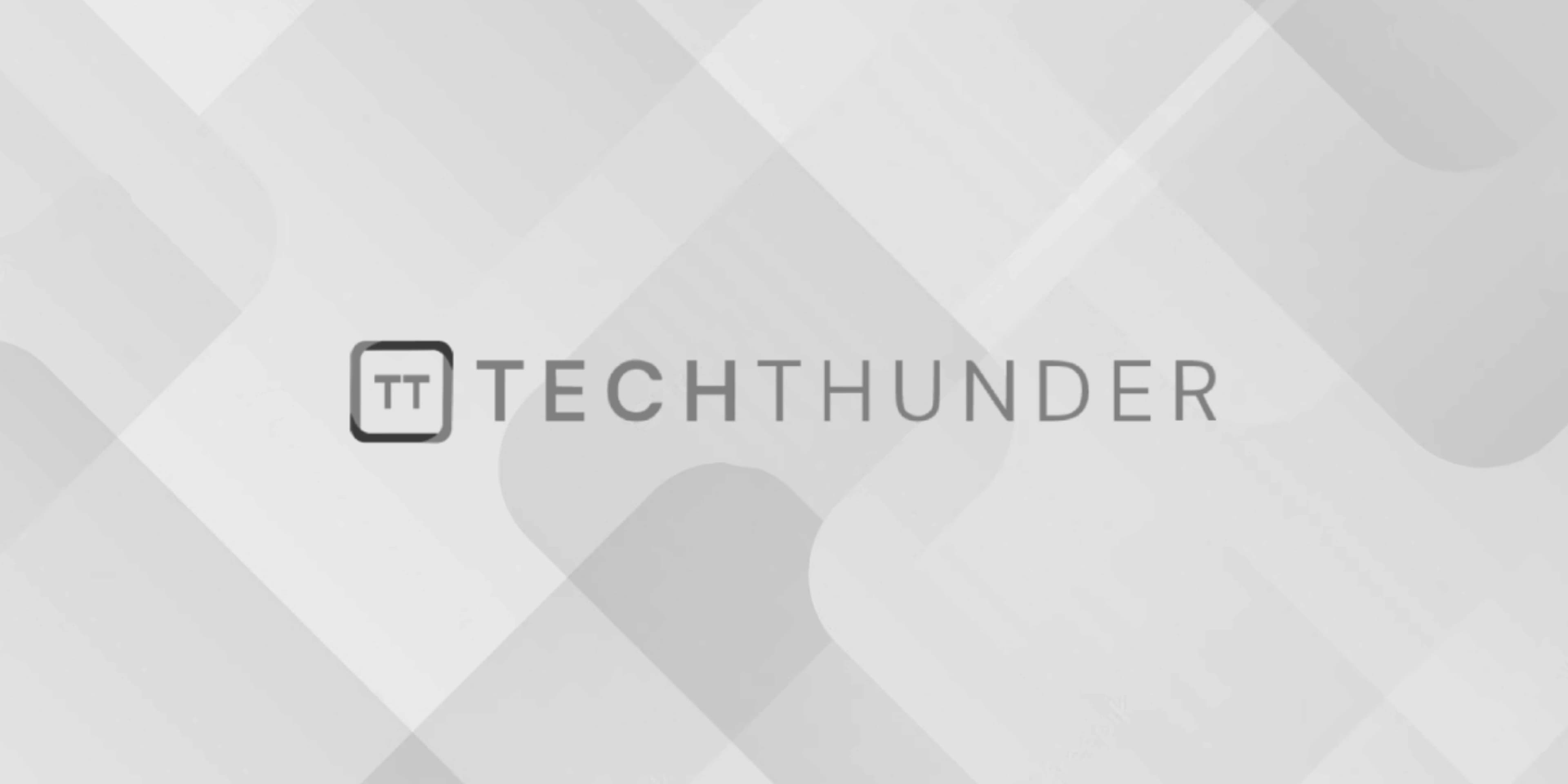
124 views
ReactJS Axios Delete Request Example
To make a DELETE request using Axios in a ReactJS application, you’ll first need to have Axios installed in your project. If you haven’t already installed it, you can do so using npm or yarn:
Bash
npm install axios
# or
yarn add axios
Once Axios is installed, you can use it to send a DELETE request to your API or server. Here’s an example of how to make a DELETE request in a React component:
TypeScript
import React, { Component } from 'react';
import axios from 'axios';
class DeleteExample extends Component {
constructor(props) {
super(props);
this.state = {
message: '', // To store the response message
};
}
handleDelete = () => {
// Replace 'your_api_endpoint_here' with the actual API endpoint you want to send the DELETE request to
axios.delete('your_api_endpoint_here')
.then((response) => {
console.log(response.data); // You can optionally log the response data
this.setState({ message: 'Delete successful' });
})
.catch((error) => {
console.error(error);
this.setState({ message: 'Delete failed' });
});
};
render() {
return (
<div>
<h1>Delete Request Example</h1>
<button onClick={this.handleDelete}>Delete Data</button>
<p>{this.state.message}</p>
</div>
);
}
}
export default DeleteExample;
In this example:
- We import React, Component, and Axios.
- We create a component called
DeleteExample
. - In the constructor, we initialize the component state with a
message
property to store the response message. - We define a
handleDelete
function that sends a DELETE request using Axios when a button is clicked. Replace'your_api_endpoint_here'
with the actual endpoint you want to use. - If the DELETE request is successful, we update the
message
state to indicate success. If it fails, we update it to indicate failure. - In the render method, we display a button to trigger the DELETE request and show the response message.
Remember to replace 'your_api_endpoint_here'
with the actual URL of the resource you want to delete. Additionally, you should handle error responses and success responses from your server API as needed for your application.