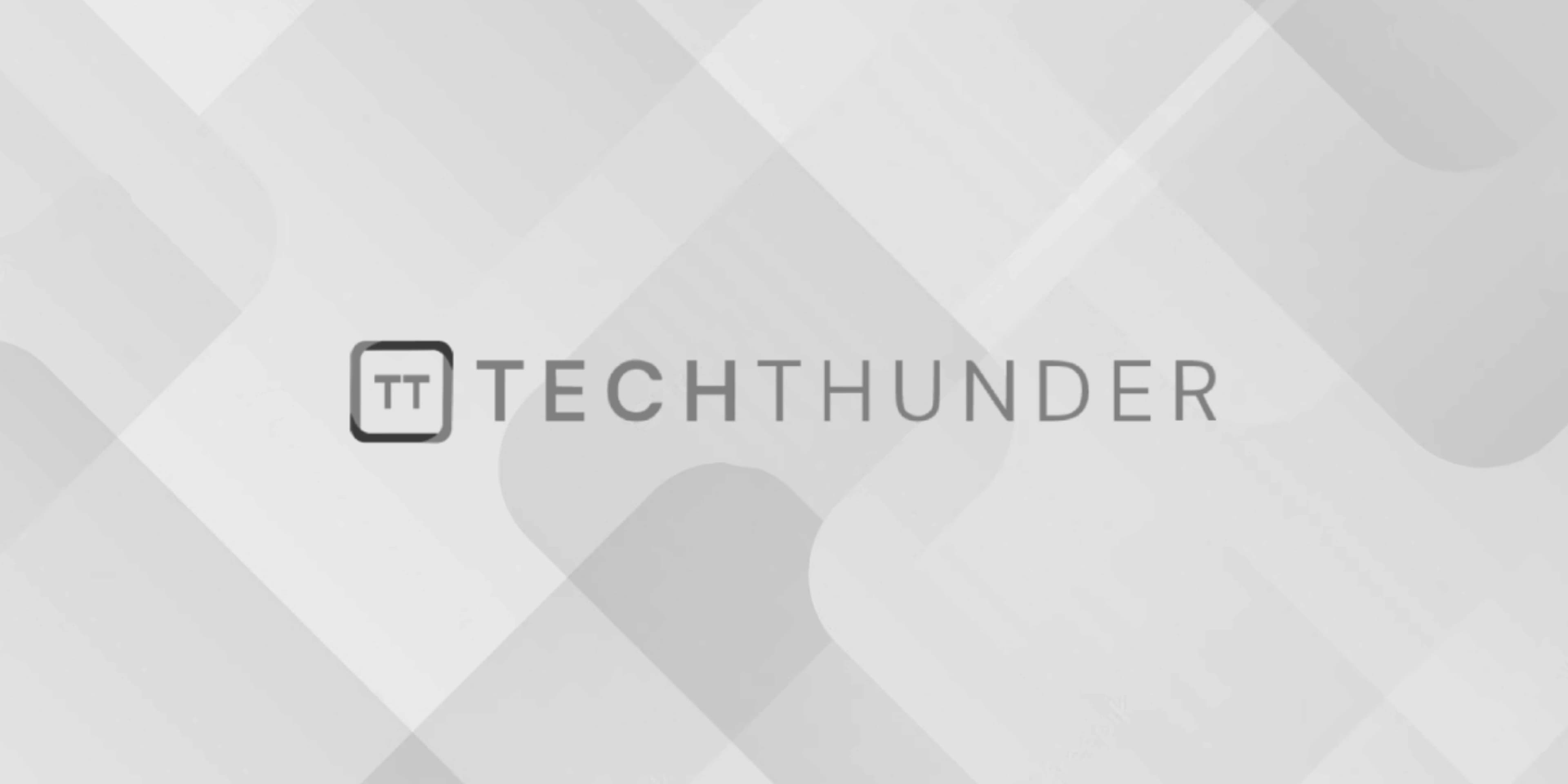
223 views
ReactJS Helmet
The react-helmet
is a library that allows you to manage the <head>
of your HTML documents, which includes elements like <title>
, <meta>
, and <link>
. This can be particularly useful in a single-page application (SPA) built with React because you may want to dynamically update the document’s head based on the current route or component data.
Here’s how you can use react-helmet
in your React application:
- Install
react-helmet
: You need to install thereact-helmet
package in your project. You can do this using npm or yarn:
Bash
npm install react-helmet
# or
yarn add react-helmet
- Import
Helmet
: In the component where you want to manage the document’s head, import theHelmet
component fromreact-helmet
.
TypeScript
import { Helmet } from 'react-helmet';
- Use
Helmet
to Manage Head Elements: You can use theHelmet
component to dynamically set the content of the<head>
section of your HTML document. For example, you can set the title, add meta tags, link to external stylesheets, and more.
TypeScript
import React from 'react';
import { Helmet } from 'react-helmet';
function MyComponent() {
return (
<div>
<Helmet>
<title>My Page Title</title>
<meta name="description" content="This is a description of my page." />
<link rel="stylesheet" href="path/to/stylesheet.css" />
{/* Add more head elements as needed */}
</Helmet>
{/* Rest of your component */}
<h1>Hello, World!</h1>
</div>
);
}
export default MyComponent;
- Dynamic Head Updates: You can also use
Helmet
to dynamically update the head based on component data or the current route. For example, if you’re using a router like React Router, you can update the title based on the current route:
TypeScript
import React from 'react';
import { Helmet } from 'react-helmet';
import { useParams } from 'react-router-dom';
function ProductDetails() {
const { productId } = useParams();
return (
<div>
<Helmet>
<title>Product Details - {productId}</title>
</Helmet>
{/* Rest of your component */}
<h1>Product Details</h1>
<p>Product ID: {productId}</p>
</div>
);
}
export default ProductDetails;
By using react-helmet
, you can keep your React components self-contained while still managing the document’s head elements in a clean and organized way. This can be especially valuable in larger SPAs or when you need to optimize SEO by dynamically setting metadata for each page.