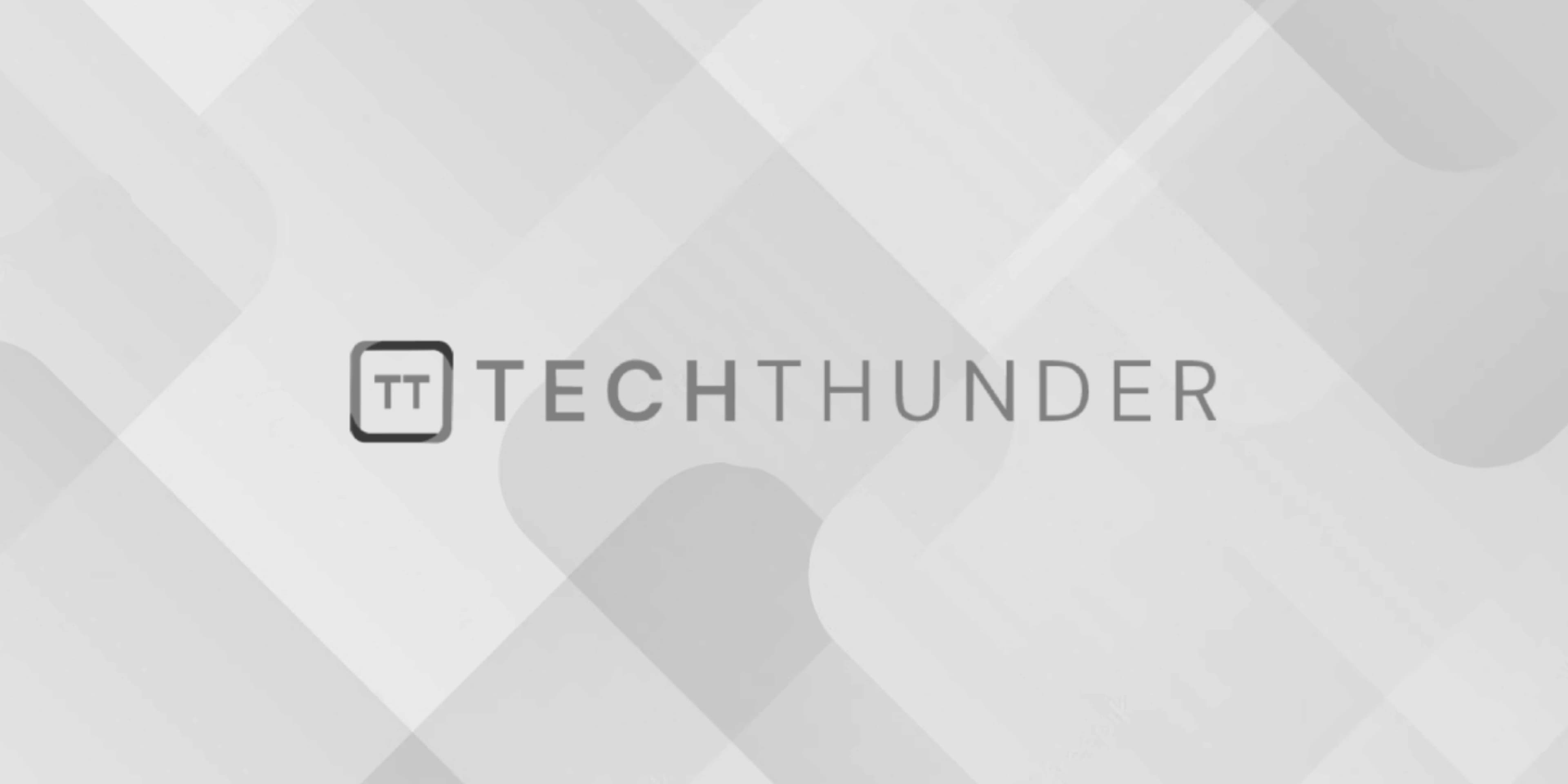
ReactJS Error Boundaries
Error Boundaries are a feature in React that help you catch and handle errors that occur during rendering, in lifecycle methods, or inside the constructors of the whole tree of React components. They are used to prevent the entire component tree from breaking due to a single error. Instead, they allow you to gracefully handle the error, display a fallback UI, and potentially recover from the error without affecting the entire application.
To use Error Boundaries in React, you need to create a special component that defines an error
state and implements the componentDidCatch
lifecycle method. Here’s how you can do it:
- Create an ErrorBoundary component:
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
componentDidCatch(error, errorInfo) {
// You can log the error or perform other error handling here
console.error('Error:', error);
console.error('Error Info:', errorInfo);
// Set the state to indicate that an error has occurred
this.setState({ hasError: true });
}
render() {
if (this.state.hasError) {
// Render a fallback UI
return <h1>Something went wrong.</h1>;
}
// Render children if no error
return this.props.children;
}
}
export default ErrorBoundary;
- Use the ErrorBoundary component:
Wrap the components that you want to be covered by the Error Boundary with the ErrorBoundary
component. This way, if an error occurs within these wrapped components, the Error Boundary will catch it.
import React from 'react';
import ErrorBoundary from './ErrorBoundary';
function MyComponent() {
// ...
}
function App() {
return (
<div className="App">
<h1>My App</h1>
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
</div>
);
}
export default App;
In this example, if an error occurs in the MyComponent
, the ErrorBoundary
will catch it and display the fallback UI (in this case, a simple “Something went wrong.” message). This prevents the entire application from crashing due to the error.
Keep in mind that Error Boundaries only catch errors within the component tree below them. They won’t catch errors in event handlers, asynchronous code, or during server-side rendering. Also, Error Boundaries are not meant to handle all types of errors; they are best suited for catching rendering errors.
Using Error Boundaries can improve the user experience by preventing crashes and showing informative messages when something goes wrong within a specific component.