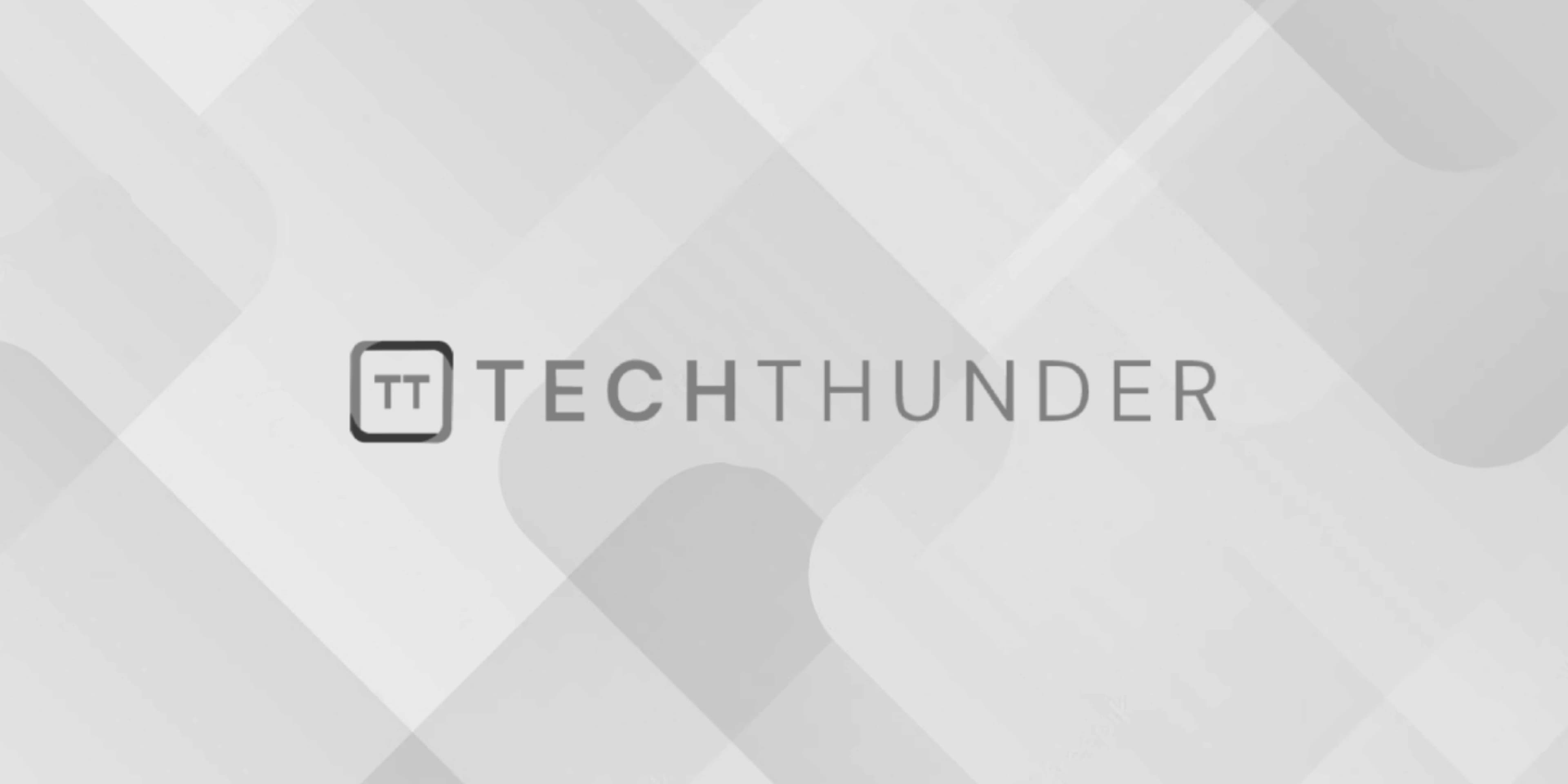
What is the useState in ReactJS
The useState
is a hook in React.js that allows functional components to manage state. Prior to the introduction of hooks in React (before version 16.8), state management was primarily done using class components and the setState
method. Hooks like useState
provide a more concise and intuitive way to manage state in functional components.
Here’s how you can use the useState
hook in a functional component:
import React, { useState } from 'react';
function Counter() {
// useState returns an array with two elements:
// 1. The current state value.
// 2. A function to update the state.
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
In the example above, the useState
hook is used to initialize a state variable called count
with an initial value of 0
. The hook returns an array where the first element is the current state (count
) and the second element is a function (setCount
) to update that state.
When the button is clicked, the increment
function is called, which uses setCount
to update the count
state. React will then re-render the component, reflecting the updated state.
In summary, useState
is a fundamental React hook that allows functional components to manage their own state, making it possible to create dynamic and interactive user interfaces without using class components.