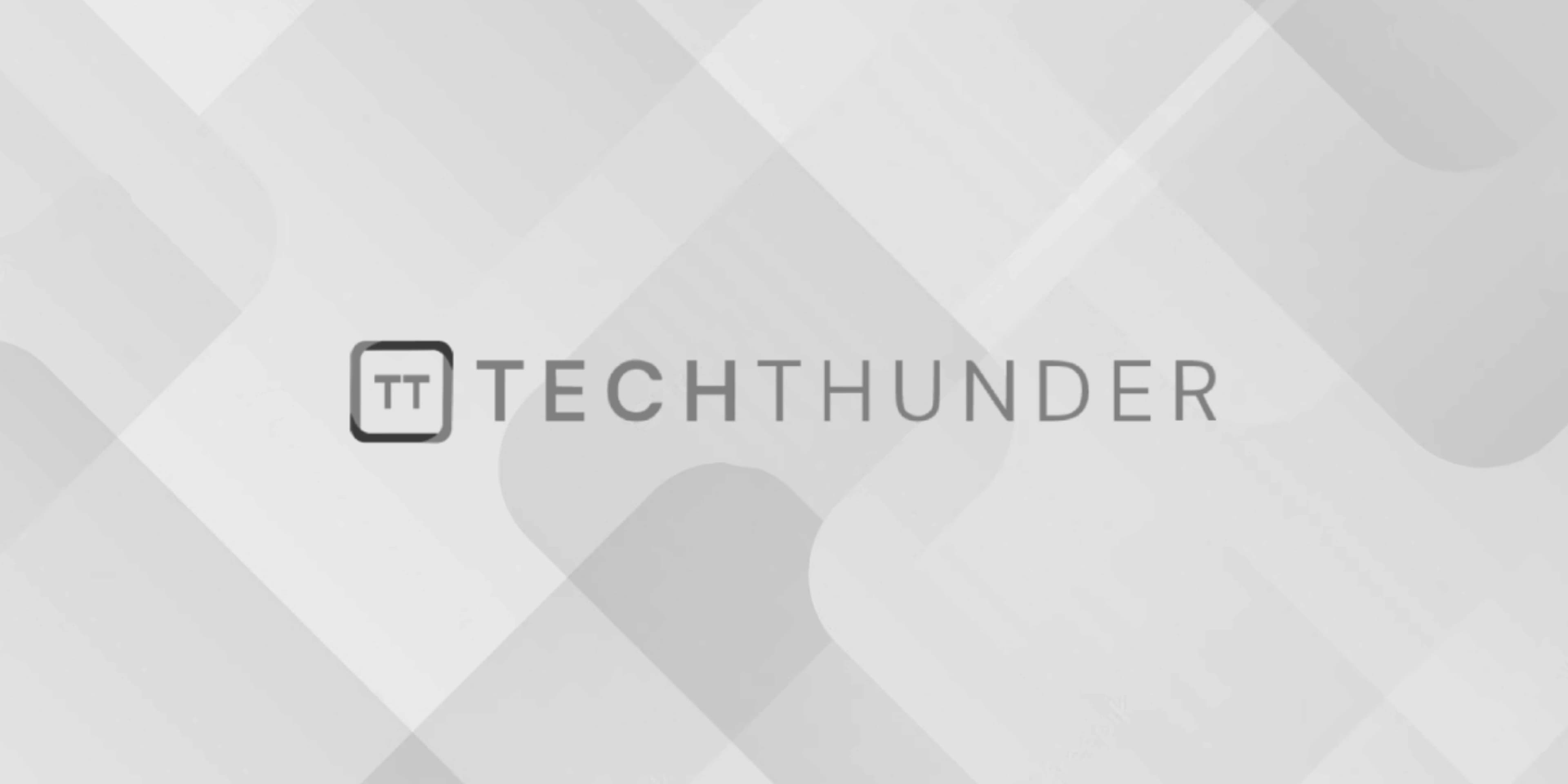
ReactJS Fragments
The ReactJS fragments are a way to group multiple children elements without adding an extra parent element to the DOM. Fragments allow you to group elements without introducing additional nodes in the rendered HTML output, which can be especially useful when you want to maintain a clean and semantic structure in your rendered components.
Here’s how you can use React fragments:
Using Empty Angle Brackets <> and :
In JSX, you can use empty angle brackets <></>
(also known as a shorthand for React fragments) to wrap multiple elements without creating an extra parent element in the DOM:
import React from 'react';
function MyComponent() {
return (
<>
<h1>Hello</h1>
<p>React Fragments</p>
</>
);
}
export default MyComponent;
Using <React.Fragment>
Explicitly:
You can also use <React.Fragment>
explicitly to achieve the same result:
import React from 'react';
function MyComponent() {
return (
<React.Fragment>
<h1>Hello</h1>
<p>React Fragments</p>
</React.Fragment>
);
}
export default MyComponent;
Key Usage Scenarios for React Fragments:
- Grouping Elements: Fragments are commonly used to group a set of elements without introducing an additional DOM element. This is useful when rendering a list of items, for example.
import React from 'react';
function ItemList({ items }) {
return (
<ul>
{items.map((item, index) => (
<React.Fragment key={index}>
<li>{item.name}</li>
<li>{item.description}</li>
</React.Fragment>
))}
</ul>
);
}
export default ItemList;
- Returning Multiple Elements from a Component: In a React component, you can only return a single element. Fragments allow you to return multiple elements from a component without wrapping them in a single parent element.
import React from 'react';
function MyComponent() {
return (
<>
<h1>Title</h1>
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</>
);
}
export default MyComponent;
- Improving Performance: In cases where you need to map over a list of items and render them within a parent element, using fragments can help improve performance because you avoid creating unnecessary parent elements in the DOM.
import React from 'react';
function ItemList({ items }) {
return (
<ul>
{items.map((item) => (
<React.Fragment key={item.id}>
<li>{item.name}</li>
<li>{item.description}</li>
</React.Fragment>
))}
</ul>
);
}
export default ItemList;
React fragments are a handy tool for structuring your components in a clean and semantic way while avoiding unnecessary elements in the rendered output. They are especially useful in situations where you need to group elements without introducing additional DOM nodes.