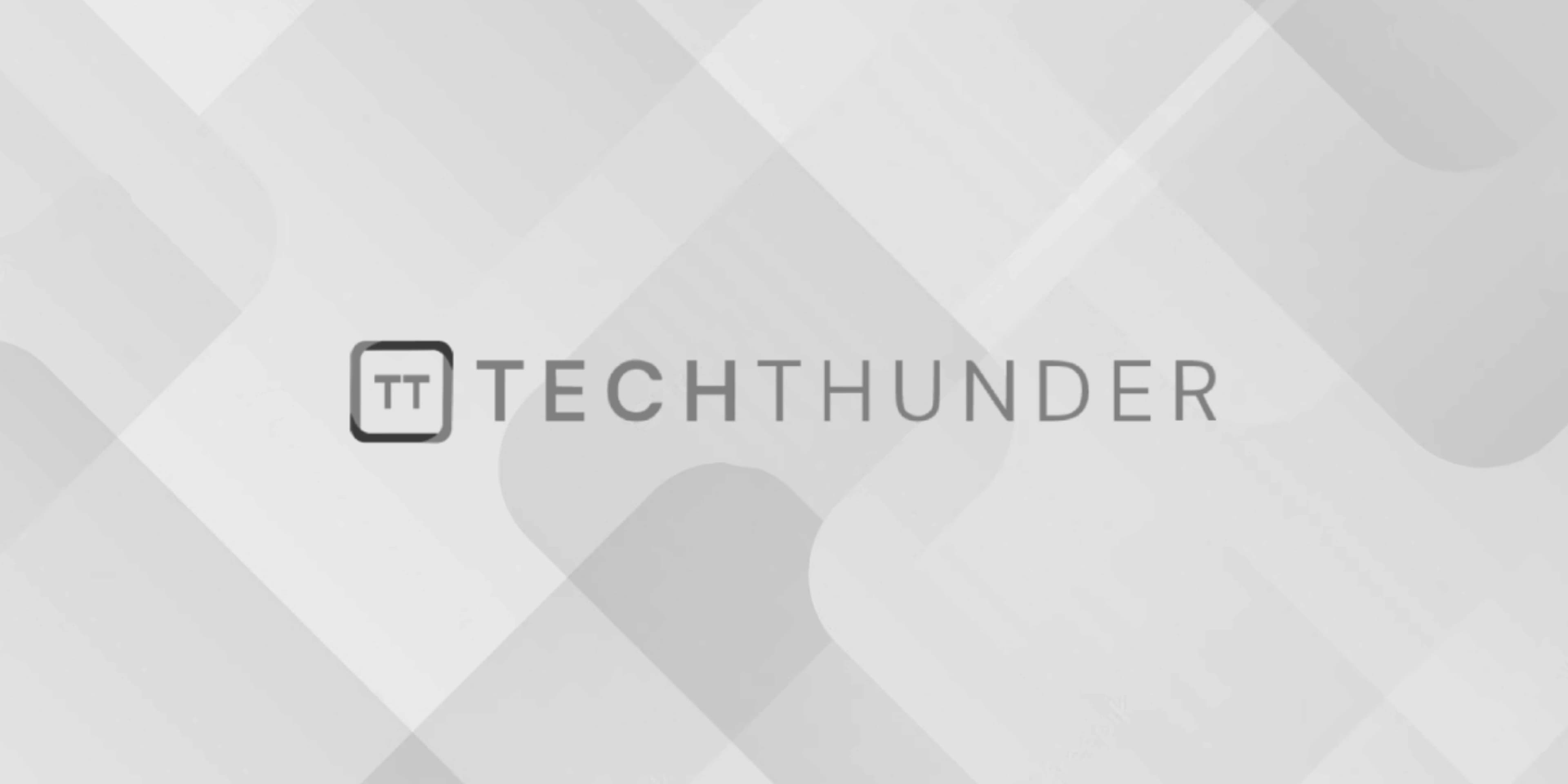
ReactJS Events
The ReactJS handle events in a way similar to handling events in traditional HTML and JavaScript. However, React introduces some syntactical differences and best practices. Here’s how you can work with events in React:
1. Event Handling Syntax:
In React, you use the onClick
(or other event names) prop to attach event handlers to elements. Instead of passing a string with JavaScript code, you pass a reference to a function that will be called when the event occurs.
import React from 'react';
function MyComponent() {
function handleClick() {
alert('Button clicked!');
}
return (
<button onClick={handleClick}>Click Me</button>
);
}
export default MyComponent;
In this example, the handleClick
function is called when the button is clicked.
2. Event Object:
React provides an event object that is similar to the native JavaScript event object, but it’s a synthetic event object with some cross-browser compatibility improvements.
import React from 'react';
function MyComponent() {
function handleInputChange(event) {
console.log(event.target.value);
}
return (
<input type="text" onChange={handleInputChange} />
);
}
export default MyComponent;
In this example, event.target.value
gives you the value of the input field when its value changes.
3. Event Handling in Class Components:
In class components, event handlers are defined as methods of the component class.
import React, { Component } from 'react';
class MyComponent extends Component {
handleClick() {
alert('Button clicked!');
}
render() {
return (
<button onClick={this.handleClick}>Click Me</button>
);
}
}
export default MyComponent;
4. Prevent Default Behavior:
You can use event.preventDefault()
to prevent the default behavior of an event, such as preventing a form from submitting or a link from navigating to a new page.
import React from 'react';
function MyComponent() {
function handleLinkClick(event) {
event.preventDefault();
alert('Link clicked but navigation prevented.');
}
return (
<a href="https://example.com" onClick={handleLinkClick}>Click Me</a>
);
}
export default MyComponent;
5. Binding Event Handlers:
When defining event handlers in class components, you may need to bind this
to the function in the constructor or use arrow functions to ensure the correct this
context.
Binding in the constructor:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor() {
super();
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
alert('Button clicked!');
}
render() {
return (
<button onClick={this.handleClick}>Click Me</button>
);
}
}
export default MyComponent;
Using arrow functions (no explicit binding needed):
import React, { Component } from 'react';
class MyComponent extends Component {
handleClick = () => {
alert('Button clicked!');
}
render() {
return (
<button onClick={this.handleClick}>Click Me</button>
);
}
}
export default MyComponent;
These are some common patterns for handling events in React. Keep in mind that event handling in React follows similar principles to vanilla JavaScript, but the syntax and patterns used in React components can be more declarative and intuitive.