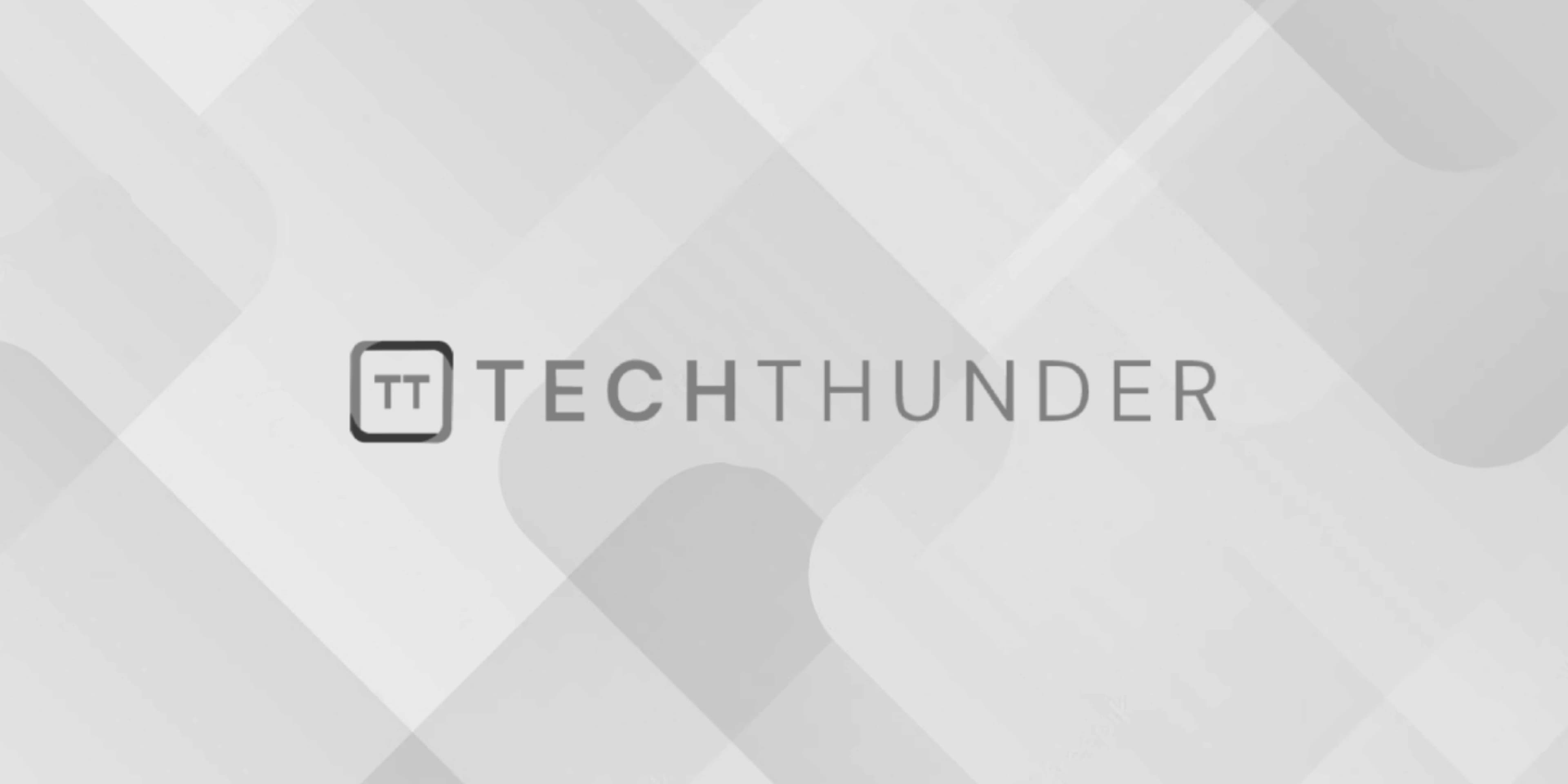
Carousel in ReactJS
Creating a carousel component in ReactJS allows you to display a rotating set of images or content items. There are different ways to implement a carousel, but one common approach is to use a library like react-responsive-carousel
for ease and functionality. Here’s how you can set up a basic image carousel using this library:
- Install the
react-responsive-carousel
library:
npm install react-responsive-carousel
- Create a Carousel Component:
Create a new React component to serve as your carousel. Here’s a simple example using images:
import React from 'react';
import { Carousel } from 'react-responsive-carousel';
import 'react-responsive-carousel/lib/styles/carousel.min.css';
function ImageCarousel() {
return (
<Carousel>
<div>
<img src="image1.jpg" alt="Image 1" />
</div>
<div>
<img src="image2.jpg" alt="Image 2" />
</div>
<div>
<img src="image3.jpg" alt="Image 3" />
</div>
</Carousel>
);
}
export default ImageCarousel;
- Use the Carousel Component:
Incorporate your ImageCarousel
component in your main app or another relevant component:
import React from 'react';
import ImageCarousel from './ImageCarousel';
function App() {
return (
<div className="App">
<h1>Image Carousel Example</h1>
<ImageCarousel />
</div>
);
}
export default App;
- Customization:
The react-responsive-carousel
library provides a range of options to customize the carousel’s behavior, styling, and navigation controls. Refer to the library’s documentation for details on available props and configuration.
Remember that this is just one example using the react-responsive-carousel
library. There are other carousel libraries and custom implementations available if you want to explore different options.