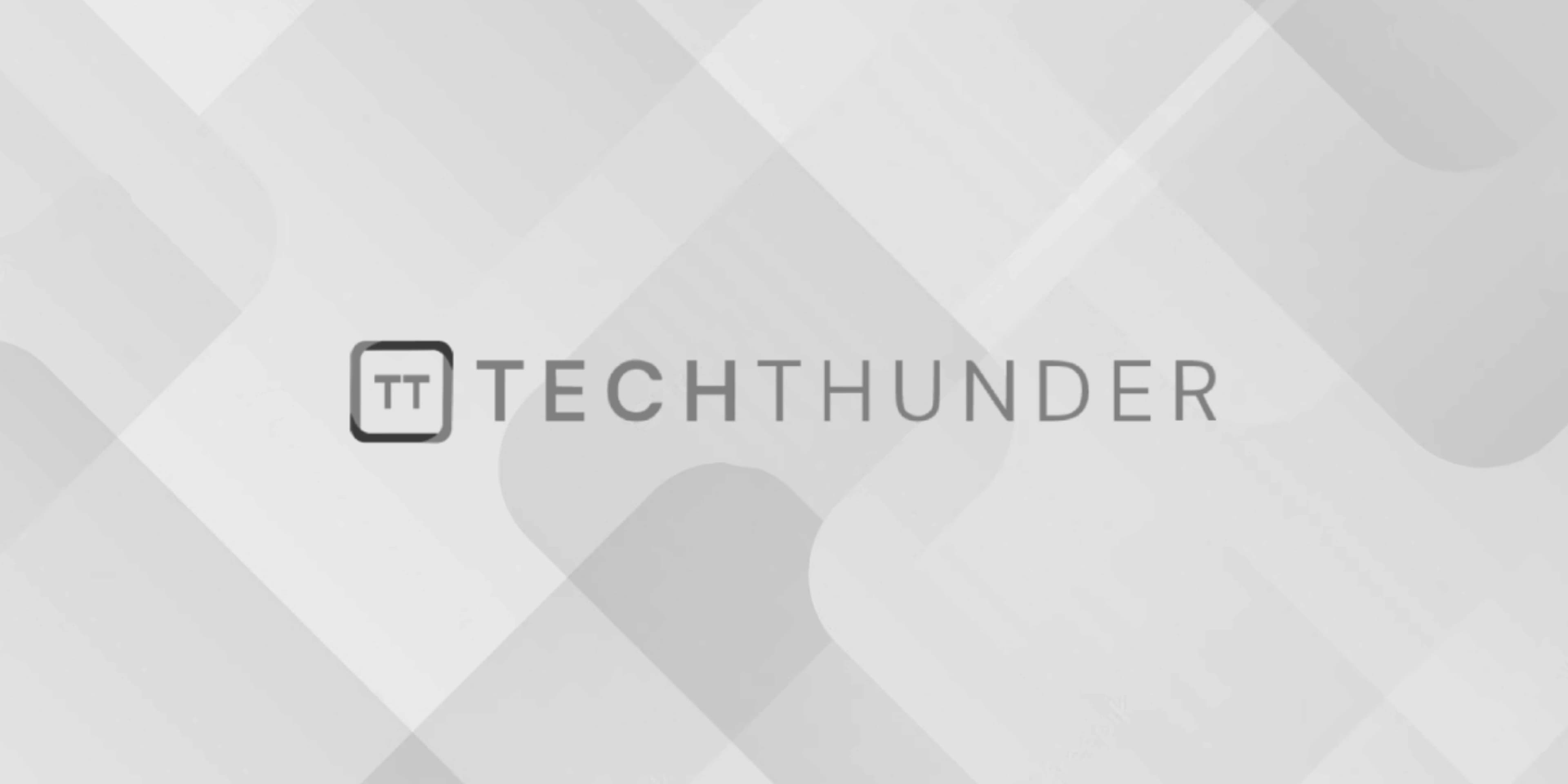
Configuring Auto Generation of Swagger Documentation
Swagger is a popular framework for documenting RESTful APIs. It provides a user-friendly interface for exploring and testing API endpoints. To configure auto-generation of Swagger documentation for your API, you’ll typically use a tool like Swagger UI or Swagger/OpenAPI specifications in combination with your web framework. Here’s a general outline of how to set this up:
- Choose a Web Framework:
- First, ensure that you are using a web framework that supports Swagger documentation. Some popular choices include Flask, Django, Express.js (Node.js), Spring Boot (Java), Ruby on Rails, and others. Each framework may have its own Swagger integration.
- Install Necessary Libraries:
- You’ll need to install specific libraries or packages that integrate Swagger with your chosen web framework. These packages often include tools for generating Swagger documentation from your code.
- Generate Swagger/OpenAPI Specifications:
- Define your API endpoints using Swagger/OpenAPI specifications. These specifications are typically written in JSON or YAML and describe your API’s structure, endpoints, request parameters, response types, and other details. You can write these specifications manually or use annotations or decorators provided by your chosen framework to generate them automatically.
- Integrate Swagger UI:
- Swagger UI is a user interface that allows users to interact with your API and view the documentation. You can integrate Swagger UI with your web application by adding it as a separate route or endpoint. Most frameworks offer a way to do this.
- Serve Swagger Documentation:
- Configure your application to serve the Swagger documentation, usually at a specific URL like “/swagger” or “/api-docs.” When users access this URL, they should see the Swagger UI interface and be able to explore your API.
- Update and Maintain Swagger Specifications:
- As you develop your API, keep the Swagger specifications up to date. Ensure that any changes to your endpoints, request/response types, and parameters are reflected in the documentation.
Here’s an example of configuring Swagger documentation for a Python Flask application using the flask-restful-swagger
extension:
from flask import Flask
from flask_restful import Api, Resource
from flask_restful_swagger import swagger
app = Flask(__name__)
api = Api(app)
api_doc = swagger.docs(Api)
class HelloWorld(Resource):
def get(self):
"""This is the docstring for the GET method."""
return {'hello': 'world'}
api.add_resource(HelloWorld, '/hello')
if __name__ == '__main__':
app.run(debug=True)
In this example, the flask-restful-swagger
extension is used to generate Swagger documentation for a Flask API. The swagger.docs()
function is used to configure Swagger documentation for the API, and the get()
method of the HelloWorld
resource includes a docstring that describes the endpoint.
Please note that the specific steps and libraries you use may vary depending on your programming language and web framework. It’s essential to consult the documentation of your chosen framework and Swagger tools for detailed instructions on integration and configuration.