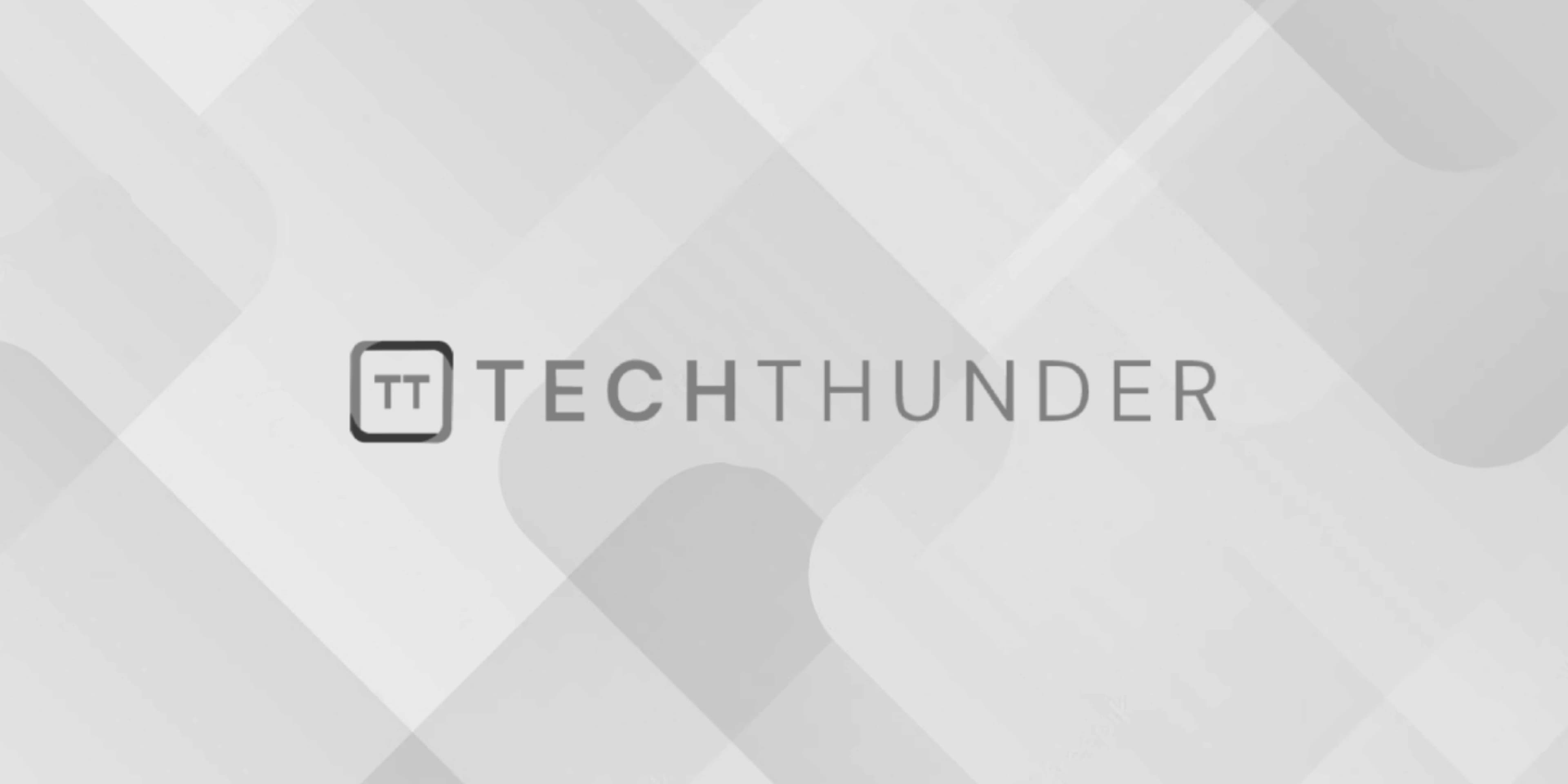
Creating Post Entity and Many to One Relationship with User Entity
To establish a @ManyToOne
relationship between a Post
entity and a User
entity in a Spring Boot application, follow these steps:
- Create the
Post
Entity:
@Entity
public class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String content;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "user_id", nullable = false)
private User user;
// Constructors, getters, setters
}
- Update the
User
Entity:
Assuming your User
entity has already been defined, you need to update it to include a collection of Post
entities:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL)
private List<Post> posts = new ArrayList<>();
// Constructors, getters, setters
}
- Cascade and Ownership:
By specifying cascade = CascadeType.ALL
in the @OneToMany
relationship, you ensure that when a User
is deleted, all associated Post
entities are also deleted.
- Update the
UserRepository
Interface:
If you haven’t already, define the UserRepository
interface by extending JpaRepository<User, Long>
.
- Creating Posts and Associating with Users:
When creating a new Post
, you’ll need to associate it with a specific User
. Here’s how you can do that in a service or controller:
@Service
public class PostService {
@Autowired
private UserRepository userRepository;
@Autowired
private PostRepository postRepository;
public Post createPost(Long userId, Post post) {
Optional<User> user = userRepository.findById(userId);
if (user.isPresent()) {
post.setUser(user.get());
return postRepository.save(post);
} else {
throw new UserNotFoundException("User not found with ID: " + userId);
}
}
}
In this example, UserNotFoundException
is a custom exception you might define to handle cases where a user with the given ID doesn’t exist.
- Update the
UserController
(if applicable):
If you have a UserController
where you handle API requests related to posts, update it to use the PostService
to create posts.
Remember to handle the required imports and ensure that your database tables are updated according to these changes (e.g., using Hibernate’s auto-update feature or using database migration tools).
With these steps, you’ve established a @ManyToOne
relationship between the Post
and User
entities, allowing you to associate posts with users and manage them using JPA in your Spring Boot application.