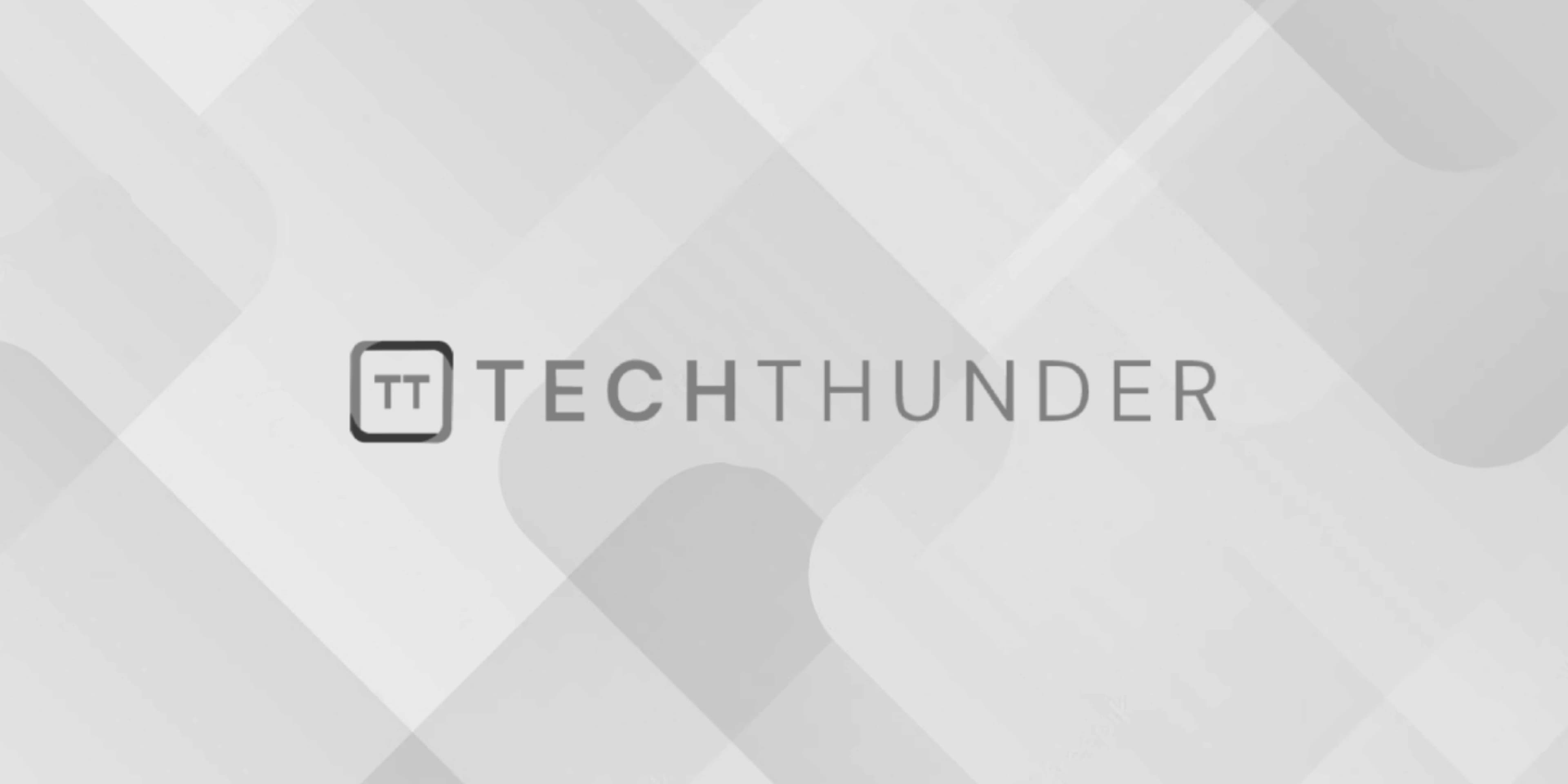
206 views
Updating POST and DELETE methods on UserResource to use JPA
To update the POST
and DELETE
methods in your UserController
to use JPA for creating and deleting users in a Spring Boot application:
Assuming you have your User
entity and UserRepository
as described earlier, here’s how you can update the relevant methods:
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
// ... Other methods for getting users ...
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userRepository.save(user);
return ResponseEntity.created(URI.create("/users/" + createdUser.getId())).body(createdUser);
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteUser(@PathVariable Long id) {
Optional<User> user = userRepository.findById(id);
if (user.isPresent()) {
userRepository.deleteById(id);
return ResponseEntity.noContent().build();
} else {
return ResponseEntity.notFound().build();
}
}
}
In the updated code:
- The
createUser(@RequestBody User user)
method usesuserRepository.save(user)
to persist the new user entity into the database. TheResponseEntity.created
method is used to create a201 Created
response along with the location of the created resource in the response header. - The
deleteUser(@PathVariable Long id)
method usesuserRepository.findById(id)
to check if the user with the given ID exists in the database. If the user exists, it’s deleted usinguserRepository.deleteById(id)
and a204 No Content
response is returned. If the user does not exist, a404 Not Found
response is returned.
By utilizing Spring Data JPA’s methods for saving and deleting entities, you simplify the process of interacting with the database, and your code becomes more readable and maintainable.
Make sure to properly handle error cases and validation in your methods as needed based on your application’s requirements.