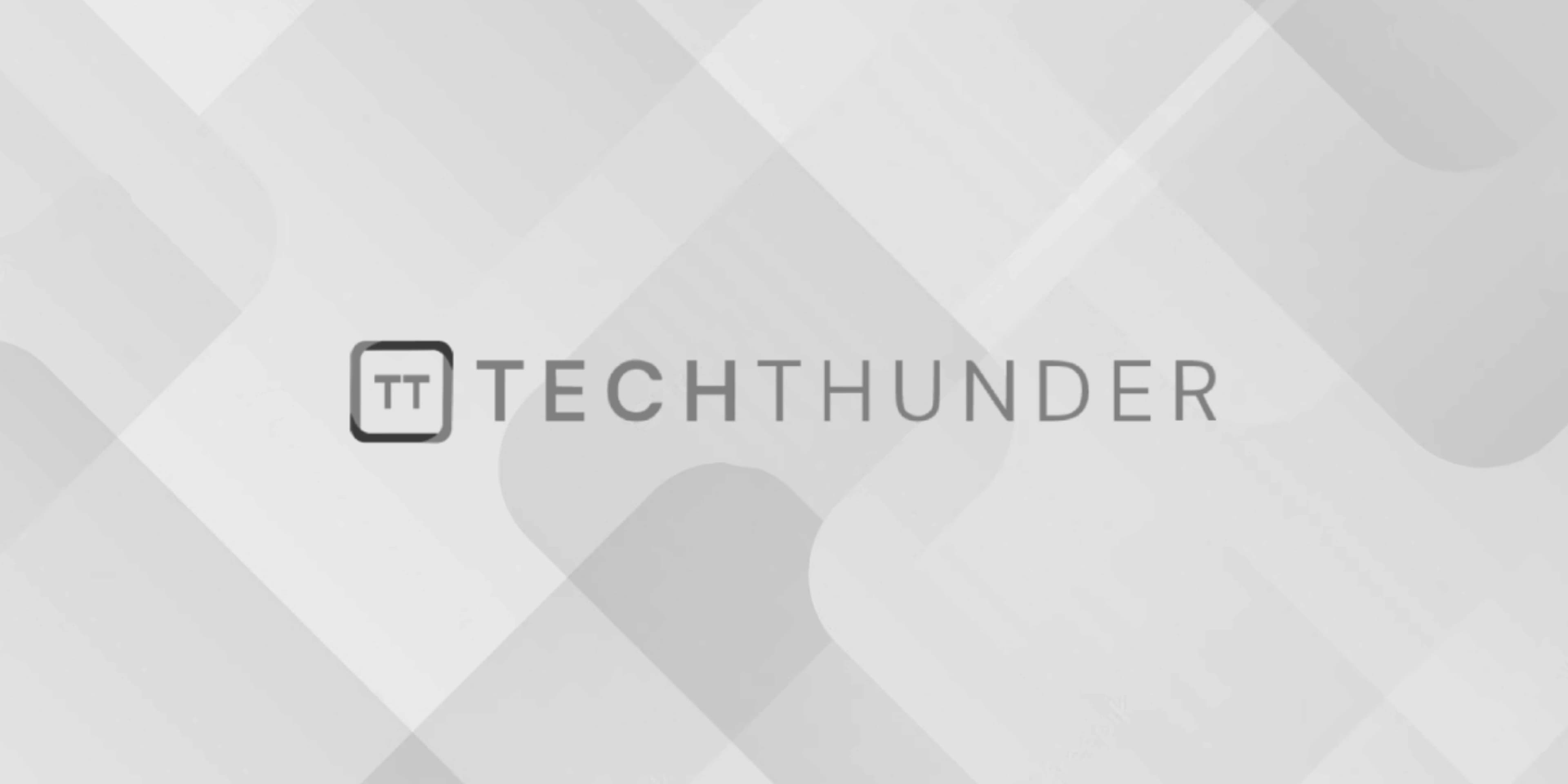
Content Negotiation Implementing Support for XML
Content negotiation is the process by which a client and a server agree on the format of data to be exchanged during an HTTP request. When implementing support for XML in content negotiation, you allow clients to request data in XML format, and the server responds accordingly. Here are the steps to implement content negotiation for XML in a web application:
- Define Supported Content Types:
- Decide which content types your web application will support. In this case, you want to support XML, so you’ll specify “application/xml” as one of the supported content types.
- Configure Your Web Server:
- Ensure that your web server is configured to handle XML content types correctly. This may involve configuring MIME types and ensuring that the server can serve XML files.
- Handle HTTP Request Headers:
- In your web application code, parse the incoming HTTP request headers, specifically the “Accept” header, to determine the client’s preferred content type. The “Accept” header specifies the content types the client can accept.
- Check for XML Request:
- Examine the “Accept” header to see if it contains “application/xml” or a similar XML-related content type. You can use regular expressions or string parsing to identify XML requests.
- Generate XML Response:
- If the client has requested XML, generate the response data in XML format. You can use libraries like XML ElementTree or generate XML manually as needed.
- Set Appropriate Response Headers:
- Set the “Content-Type” header in the HTTP response to “application/xml” to indicate that the response is in XML format. You can also set other relevant headers like “Content-Length.”
- Send the XML Response:
- Send the XML response data to the client. This can be done by writing the XML content directly to the response stream or by using a framework or library that simplifies this process.
Here’s a simplified example in Python using the Flask web framework to demonstrate content negotiation for XML:
from flask import Flask, request, Response
app = Flask(__name__)
@app.route('/data', methods=['GET'])
def get_data():
# Parse the Accept header to check for XML request
accept_header = request.headers.get('Accept')
if 'application/xml' in accept_header:
# Generate XML data (replace with your own data generation logic)
xml_data = '<data><item>Item 1</item><item>Item 2</item></data>'
# Set the response headers and return the XML response
response = Response(xml_data, content_type='application/xml')
return response
else:
# Handle other content types or provide a default response
return "Unsupported content type requested", 415 # Unsupported Media Type
if __name__ == '__main__':
app.run()
In this example, the web application checks the “Accept” header of the incoming request to determine whether the client wants XML content. If XML is requested, it generates and returns XML data as the response. If a different content type is requested, it responds with an “Unsupported Media Type” status (HTTP 415).
This is a basic example, and in a real-world application, you may have more complex XML data generation and error handling based on the specific requirements of your project.