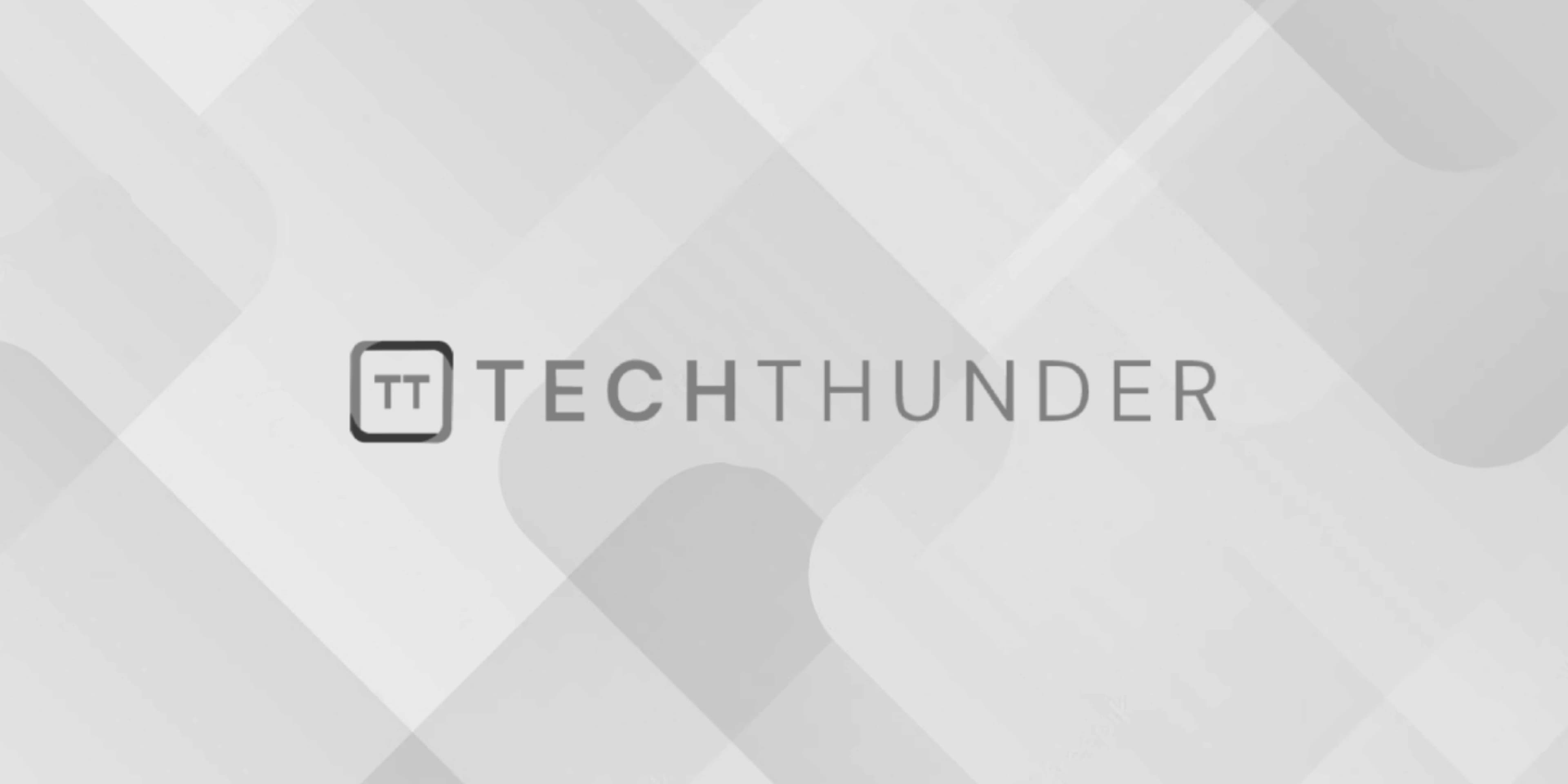
Spring Boot Caching
Spring Boot provides built-in support for caching to improve the performance of your applications by reducing the need to repeatedly fetch data or perform expensive calculations. Caching stores the results of expensive operations and serves them quickly when the same operation is requested again. Here’s a step-by-step guide on how to implement caching in a Spring Boot application:
Step 1: Create a Spring Boot Project:
You can create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Add Caching Dependency:
In your pom.xml
(if you’re using Maven) or build.gradle
(if you’re using Gradle), add the caching dependency. For example, if you want to use the default caching provider, which is based on ConcurrentHashMap, add the following Maven dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
Step 3: Enable Caching in Your Application:
In your Spring Boot application’s main class, annotate it with @EnableCaching
to enable caching.
@SpringBootApplication
@EnableCaching
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Step 4: Define Cache Configuration:
You can configure caching using properties in your application.properties
or application.yml
file. For example, to configure a simple in-memory cache with a maximum of 100 entries, add the following to application.properties
:
# Cache configuration
spring.cache.type=simple
spring.cache.cache-names=myCache
spring.cache.simple.mode=CONCURRENT
spring.cache.simple.maximum-size=100
Step 5: Annotate Methods to Cache:
In your service classes, annotate the methods that you want to cache with @Cacheable
. You can specify the cache name and optional key generation strategy.
@Service
public class MyService {
@Cacheable("myCache")
public String getCachedData(String key) {
// Expensive operation to fetch data
// This result will be cached
return fetchExpensiveData(key);
}
}
Step 6: Using the Cache:
When you call the cached method, the result will be stored in the cache for subsequent calls with the same arguments.
@RestController
public class MyController {
private final MyService myService;
@Autowired
public MyController(MyService myService) {
this.myService = myService;
}
@GetMapping("/data")
public String getData(@RequestParam String key) {
return myService.getCachedData(key);
}
}
Now, when you call /data?key=someKey
multiple times with the same someKey
, the expensive data retrieval operation will only be performed once, and subsequent calls will retrieve the data from the cache.
Step 7: Test the Caching:
Run your Spring Boot application and test the caching behavior using different input values. You can observe the performance improvement when fetching data that has already been cached.
This setup demonstrates a basic Spring Boot application with caching. You can further customize your caching strategy, use different cache providers (e.g., Ehcache, Redis), and configure eviction policies and cache management as needed for your application’s requirements.