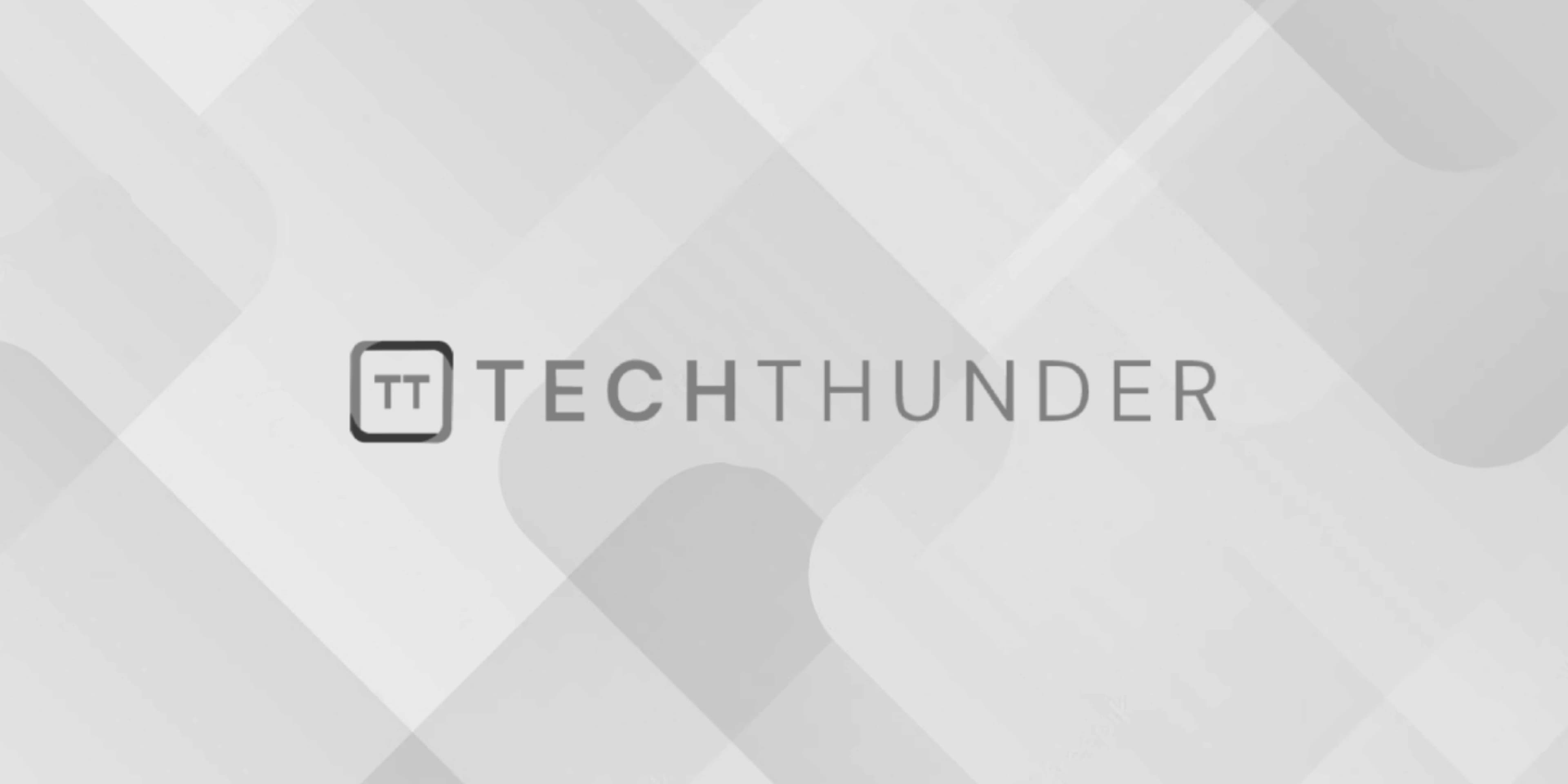
Spring Boot Project Deployment Using Tomcat
Deploying a Spring Boot project to Apache Tomcat is a common practice when you want to run your Spring Boot application in a traditional servlet container. Here are the steps to deploy a Spring Boot project using Tomcat:
Step 1: Create a Spring Boot Project
If you haven’t already, create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Modify the Project Configuration
- Open your
pom.xml
file (Maven) orbuild.gradle
(Gradle) and ensure that you have the necessary dependencies. Make sure that you have thespring-boot-starter-web
dependency, which is required for web applications. For Maven:
<dependencies>
<!-- ... other dependencies ... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
For Gradle:
dependencies {
// ... other dependencies ...
implementation 'org.springframework.boot:spring-boot-starter-web'
}
- By default, Spring Boot uses an embedded servlet container (e.g., Tomcat) for running web applications. To make your application deployable to an external Tomcat server, you need to mark the embedded container as “provided” to prevent it from being packaged into the final WAR file. To do this, add the following configuration to your
pom.xml
(Maven) orbuild.gradle
(Gradle): For Maven:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludeDevtools>false</excludeDevtools>
</configuration>
</plugin>
</plugins>
</build>
For Gradle:
configurations {
providedRuntime
}
dependencies {
providedRuntime 'org.springframework.boot:spring-boot-starter-tomcat'
}
Step 3: Create a WAR File
Spring Boot applications can be packaged as WAR (Web Application Archive) files for deployment to external servlet containers like Tomcat. To build a WAR file, use the following steps:
For Maven:
mvn clean package
For Gradle:
./gradlew build
This command will generate a WAR file in the target
(Maven) or build/libs
(Gradle) directory of your project.
Step 4: Deploy to Tomcat
- Copy the generated WAR file (e.g.,
my-application.war
) from thetarget
orbuild/libs
directory to the Tomcatwebapps
directory. - Start or restart your Tomcat server.
- Your Spring Boot application should be automatically deployed by Tomcat. You can access it at
http://localhost:8080/your-war-file-name
whereyour-war-file-name
is the name of your WAR file without the.war
extension.
Step 5: Access Your Application
Open a web browser and navigate to the URL where your Spring Boot application is deployed on Tomcat. You should be able to access and interact with your application.
That’s it! You’ve successfully deployed a Spring Boot application to Apache Tomcat. You can now access and use your application in a production environment.