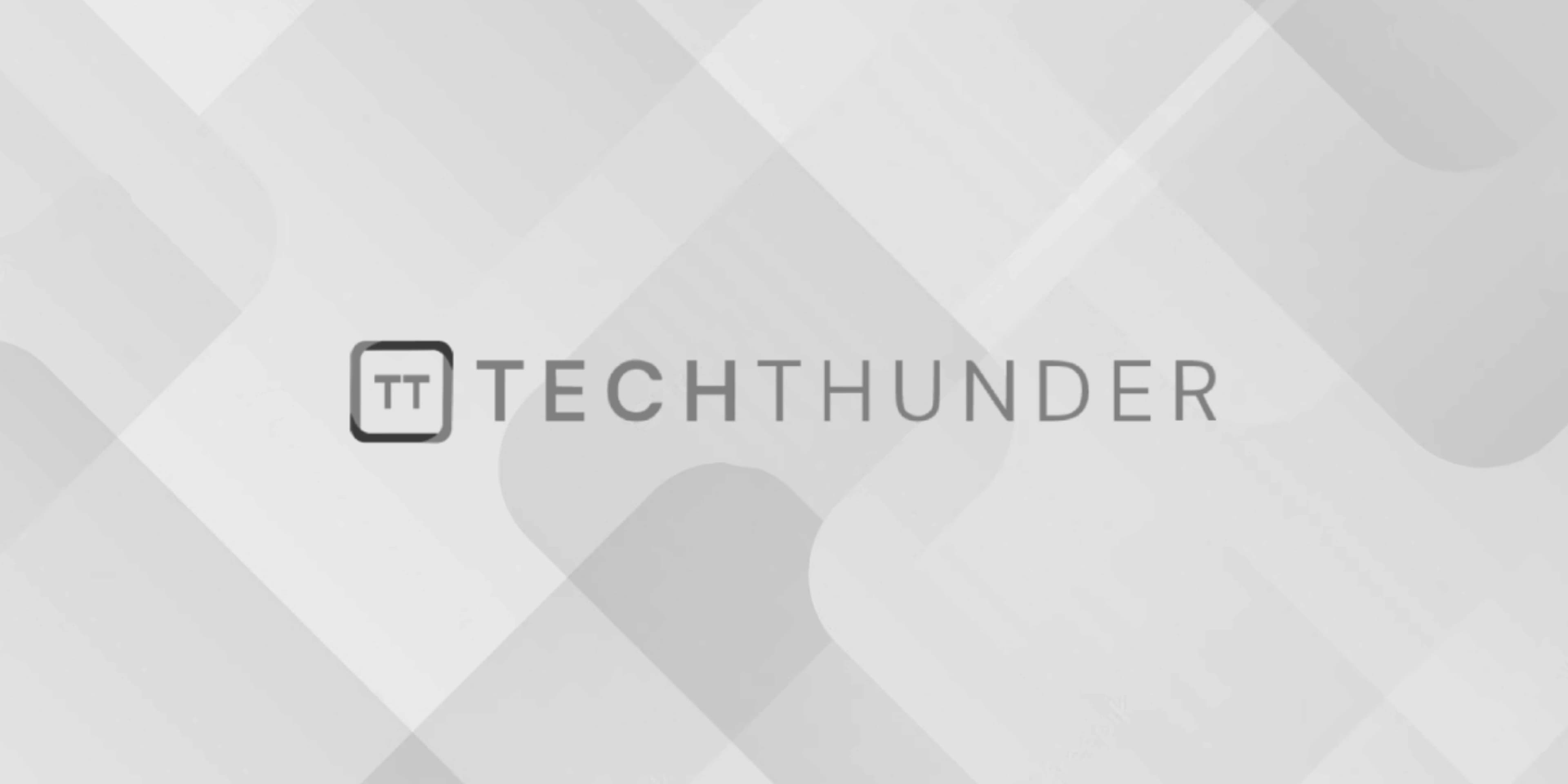
Spring Boot AOP After Advice
The Spring Boot AOP, an “after” advice is an aspect that executes after the target method has completed its execution. After advice is used to perform actions or processing after the method has finished executing, such as logging or resource cleanup. Here’s how to create an “after” advice using Spring Boot AOP:
Step 1: Create a Spring Boot Project
If you haven’t already, create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Add AOP Dependencies
In your project’s pom.xml
(Maven) or build.gradle
(Gradle), add the necessary dependencies for Spring AOP:
For Maven:
<dependencies>
<!-- ... other dependencies ... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
</dependencies>
For Gradle:
dependencies {
// ... other dependencies ...
implementation 'org.springframework.boot:spring-boot-starter-aop'
}
Step 3: Create an “After” Advice
Now, create an “after” advice class that will be executed after the target method has completed its execution. Here’s an example of an “after” advice that logs the method invocation:
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class AfterLoggingAdvice {
@After("execution(* com.example.myapp.service.*.*(..))")
public void logAfterMethod(JoinPoint joinPoint) {
String methodName = joinPoint.getSignature().getName();
System.out.println("After invoking method: " + methodName);
}
}
In this example, we’ve created an aspect named AfterLoggingAdvice
, and it defines an “after” advice method logAfterMethod
. The @After
annotation specifies the pointcut expression that determines when this advice should be executed. In this case, it is executed after any method in the com.example.myapp.service
package.
Step 4: Use AOP Annotations
To apply the “after” advice to specific methods or classes, you can use AOP annotations such as @Before
, @After
, @Around
, and others. Here’s an example of how to use @After
to apply the “after” advice to a specific method:
import org.springframework.stereotype.Service;
@Service
public class MyService {
public void doSomething() {
// This is the target method
System.out.println("Doing something...");
}
}
In this example, we’ve applied the “after” advice to the doSomething
method of the MyService
class.
Step 5: Run Your Spring Boot Application
Run your Spring Boot application as you normally would. When the doSomething
method is invoked, the “after” advice (logAfterMethod
) will execute after the method has finished its execution, and you will see the log message.
You’ve created and applied an “after” advice using Spring Boot AOP. You can use this approach to perform actions or processing after the execution of specific methods in your application, such as logging, resource cleanup, and response modification.