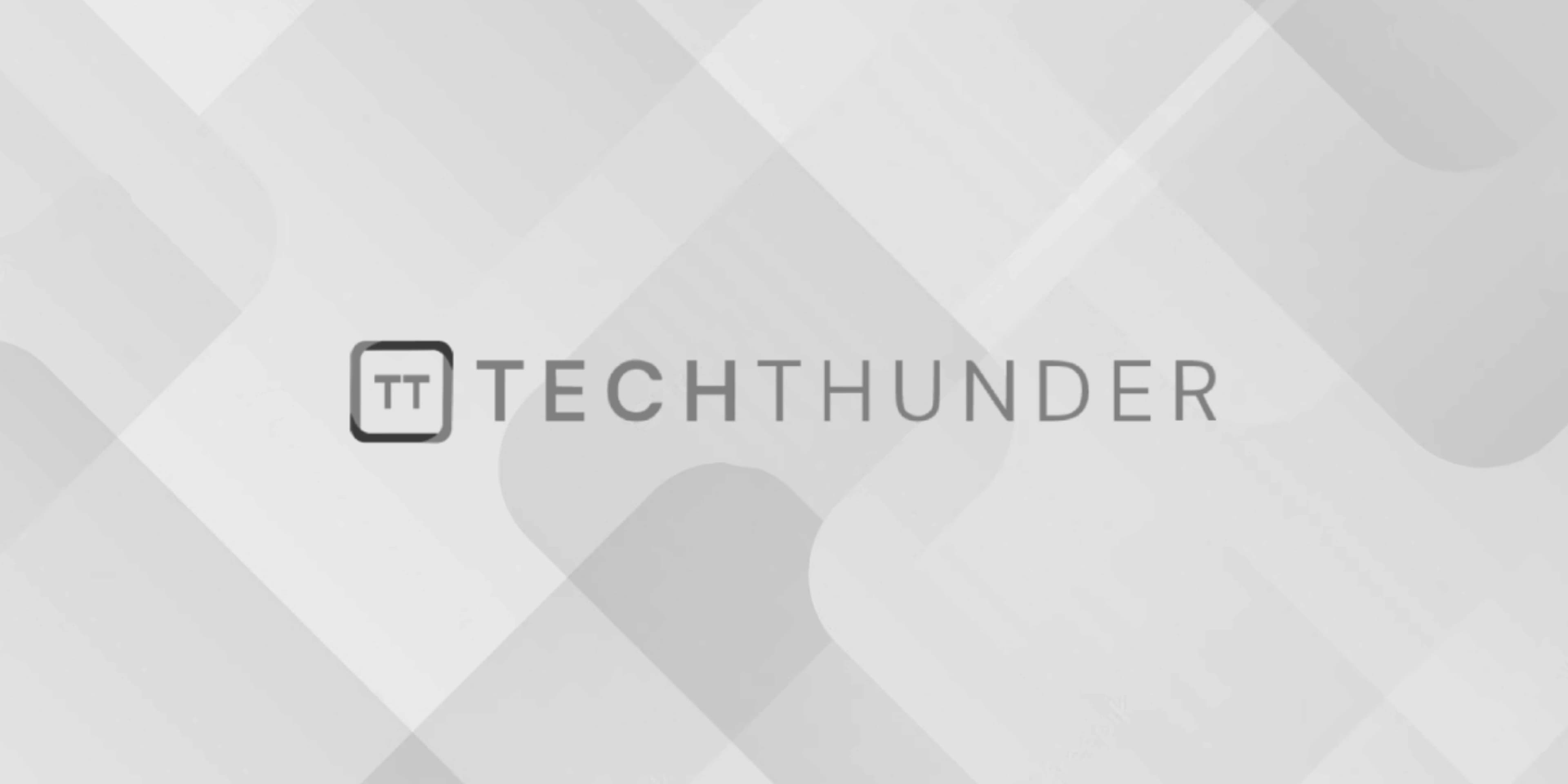
183 views
Internationalization of RESTful Services
Internationalization (i18n) is the process of designing your application to support multiple languages and cultures. Spring Boot provides built-in support for internationalization, making it relatively easy to implement in your RESTful services. Here’s how you can internationalize your Spring Boot application:
- Create Message Source Files:
Create properties files with messages for different languages. These files should be named based on the locale they represent, likemessages_en.properties
for English andmessages_fr.properties
for French. Place these files in thesrc/main/resources
directory. Examplemessages_en.properties
:
greeting=Hello
Example messages_fr.properties
:
greeting=Bonjour
- Configure Message Source:
In your application’s configuration, configure the message source to look for messages in the resource bundle. You can define thebasename
for your properties files.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.support.ReloadableResourceBundleMessageSource;
@Configuration
public class InternationalizationConfig {
@Bean
public ReloadableResourceBundleMessageSource messageSource() {
ReloadableResourceBundleMessageSource messageSource = new ReloadableResourceBundleMessageSource();
messageSource.setBasename("classpath:messages");
messageSource.setDefaultEncoding("UTF-8");
return messageSource;
}
}
- Use MessageSource in Your Controller:
Inject theMessageSource
bean into your controller to retrieve localized messages.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.MessageSource;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/greeting")
public class GreetingController {
@Autowired
private MessageSource messageSource;
@GetMapping
public String getGreeting() {
return messageSource.getMessage("greeting", null, LocaleContextHolder.getLocale());
}
}
- Testing:
You can now test your internationalized RESTful service by sending requests with differentAccept-Language
headers. For example, usingcurl
:
curl -H "Accept-Language: fr" http://localhost:8080/greeting
You should receive a response based on the locale.
Remember that this approach works well for simple text messages. For more complex scenarios, such as messages with dynamic values, consider using placeholders and parameterized messages in your properties files. Also, you can use MessageSourceAccessor
to simplify message retrieval in your code.