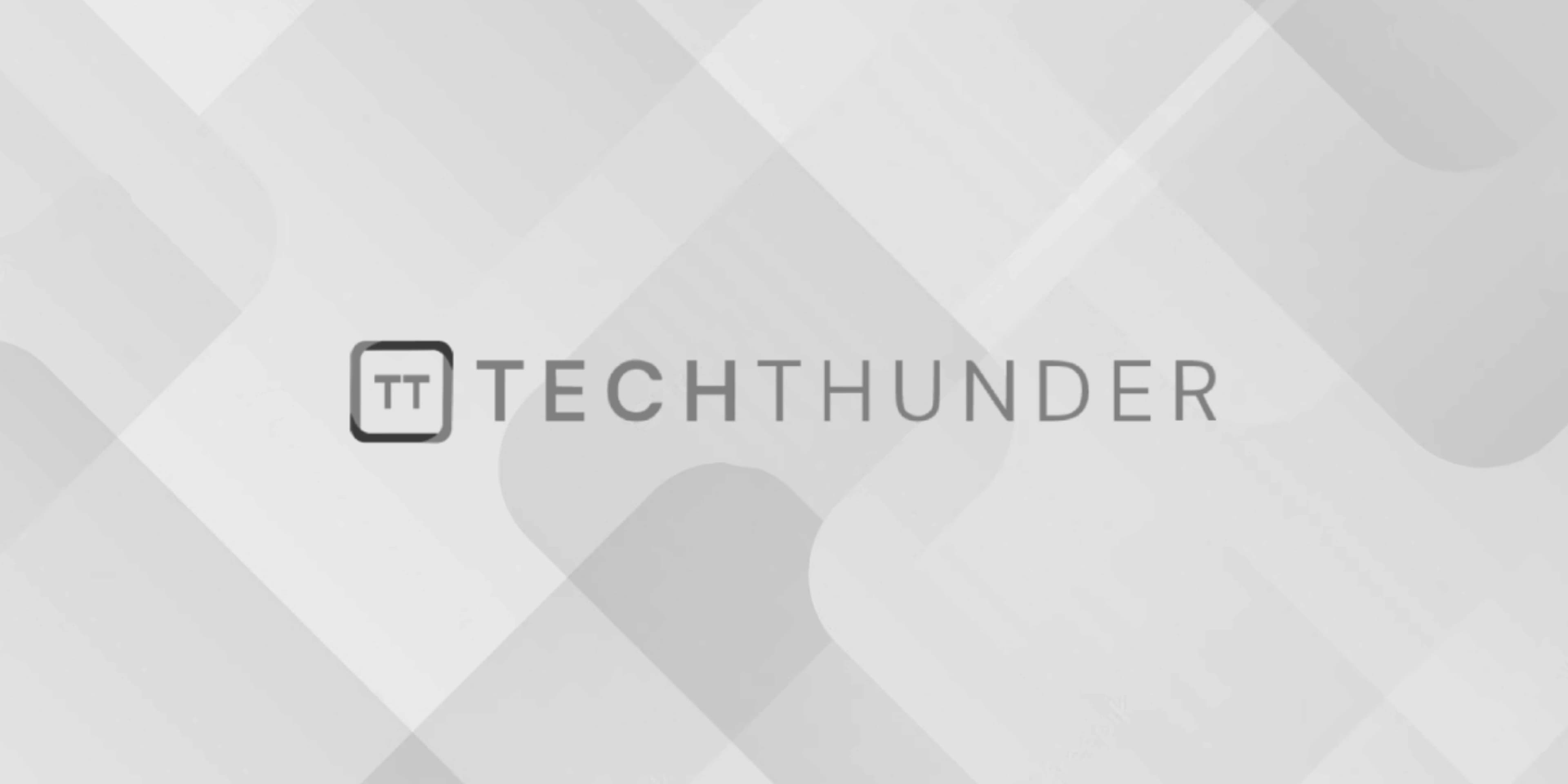
Implementing POST Service to Create a Post for a User
I can guide you through implementing a POST service to create a post for a user in a Spring Boot application. In this example, we’ll assume you’re creating a basic social media-like system where users can create posts.
1. Create a Spring Boot Project:
Start by creating a new Spring Boot project using Spring Initializr or your preferred development environment.
2. Create Entity Classes:
Create two entity classes: User
and Post
.
User.java
:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
// Getters and setters
}
Post.java
:
@Entity
public class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String content;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
// Getters and setters
}
3. Create Repositories:
Create repositories for the User
and Post
entities.
UserRepository.java
:
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
PostRepository.java
:
public interface PostRepository extends JpaRepository<Post, Long> {
List<Post> findByUser(User user);
}
4. Create Controllers:
Create a controller for handling user and post creation.
UserController.java
:
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@PostMapping
public User createUser(@RequestBody User user) {
return userRepository.save(user);
}
}
PostController.java
:
@RestController
@RequestMapping("/posts")
public class PostController {
@Autowired
private UserRepository userRepository;
@Autowired
private PostRepository postRepository;
@PostMapping("/{userId}")
public Post createPost(@PathVariable Long userId, @RequestBody Post post) {
User user = userRepository.findById(userId)
.orElseThrow(() -> new IllegalArgumentException("User not found"));
post.setUser(user);
return postRepository.save(post);
}
}
5. Test the Service:
You can now test the POST service using tools like curl
, Postman, or any other HTTP client.
- To create a user, send a POST request to
http://localhost:8080/users
with a JSON payload containing user information. - To create a post for a user, send a POST request to
http://localhost:8080/posts/{userId}
(replace{userId}
with the actual user ID) with a JSON payload containing the post content.
6. Run the Application:
Run your Spring Boot application and test the POST services using the provided endpoints.
This example demonstrates how to create a simple service to allow users to create posts. In a real-world application, you would likely include more features, security measures, and data validation to ensure the integrity and security of your system.