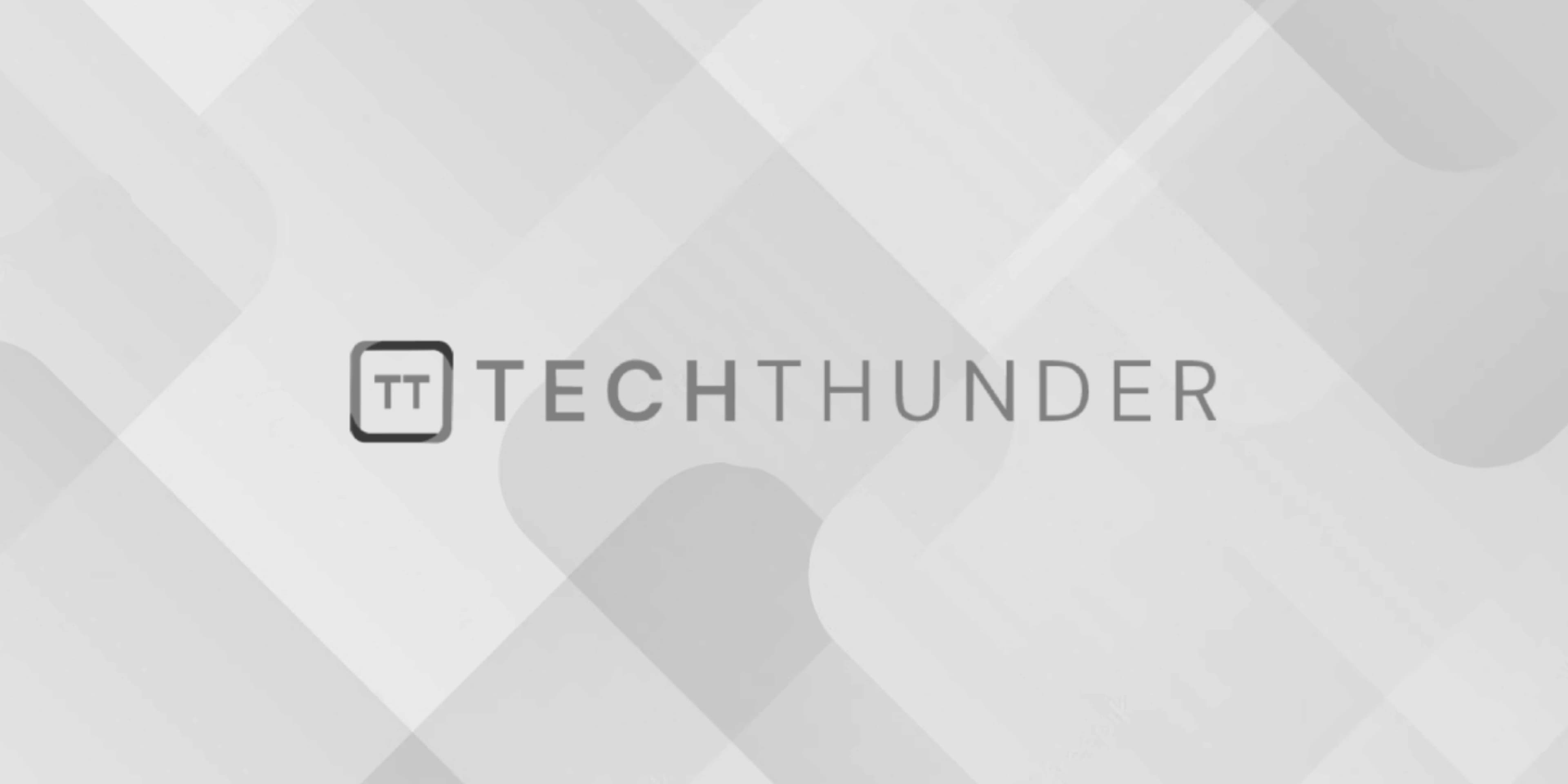
Implementing Dynamic Filtering for RESTful Services
Dynamic filtering for RESTful services allows clients to request specific fields from the response data based on their preferences. Spring Boot provides a flexible way to implement dynamic filtering using various techniques such as query parameters or custom annotations. Here’s how you can implement dynamic filtering in Spring Boot:
1. Create a Java Bean (POJO):
- Start by creating a Java bean that represents the data you want to expose through your RESTful service. This bean should have all the fields you need.
public class Employee {
private int id;
private String name;
private String department;
// getters and setters
}
2. Use a Custom Annotation (Optional):
- If you prefer, you can create a custom annotation to mark fields that should be included or excluded from the response based on the client’s request. Here’s an example of a custom annotation:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface ExcludeFromResponse { }
You can then annotate fields with @ExcludeFromResponse
to indicate that they should be excluded from the response by default.
3. Create a Resource Class:
- Create a resource class (RESTful controller) that will handle HTTP requests and return JSON responses. This class should contain methods for handling dynamic filtering based on client requests.
@RestController
public class EmployeeResource {
@GetMapping("/employees/{id}")
public MappingJacksonValue retrieveEmployee(@PathVariable int id, @RequestParam(name = "fields", required = false) String fields) {
// Create an Employee object
Employee employee = new Employee();
employee.setId(id);
employee.setName("John Doe");
employee.setDepartment("HR");
// Apply dynamic filtering based on client request
MappingJacksonValue mapping = new MappingJacksonValue(employee);
if (fields != null) {
SimpleBeanPropertyFilter filter = SimpleBeanPropertyFilter.filterOutAllExcept(fields.split(","));
FilterProvider filters = new SimpleFilterProvider().addFilter("employeeFilter", filter);
mapping.setFilters(filters);
}
return mapping;
}
}
In this example, the fields
query parameter allows clients to specify which fields they want in the response. The MappingJacksonValue
class is used to apply dynamic filtering to the response based on the specified fields.
4. Configure Dynamic Filtering:
- In your application’s configuration, you can define filters that correspond to specific dynamic filtering scenarios. For example, you can configure a filter named “employeeFilter” that defines which fields should be included or excluded.
@JsonFilter("employeeFilter")
public class Employee { ... }
5. Test the Endpoint:
- Clients can make requests to the
/employees/{id}
endpoint and include thefields
query parameter to specify which fields they want in the response. For example, a request to/employees/1?fields=id,name
would return only theid
andname
fields.
Dynamic filtering allows clients to tailor the response data to their needs, making your API more flexible. It’s essential to properly validate and sanitize the fields
parameter to prevent security vulnerabilities, such as SQL injection or data leakage. Additionally, consider providing documentation for clients to understand how to use dynamic filtering effectively.