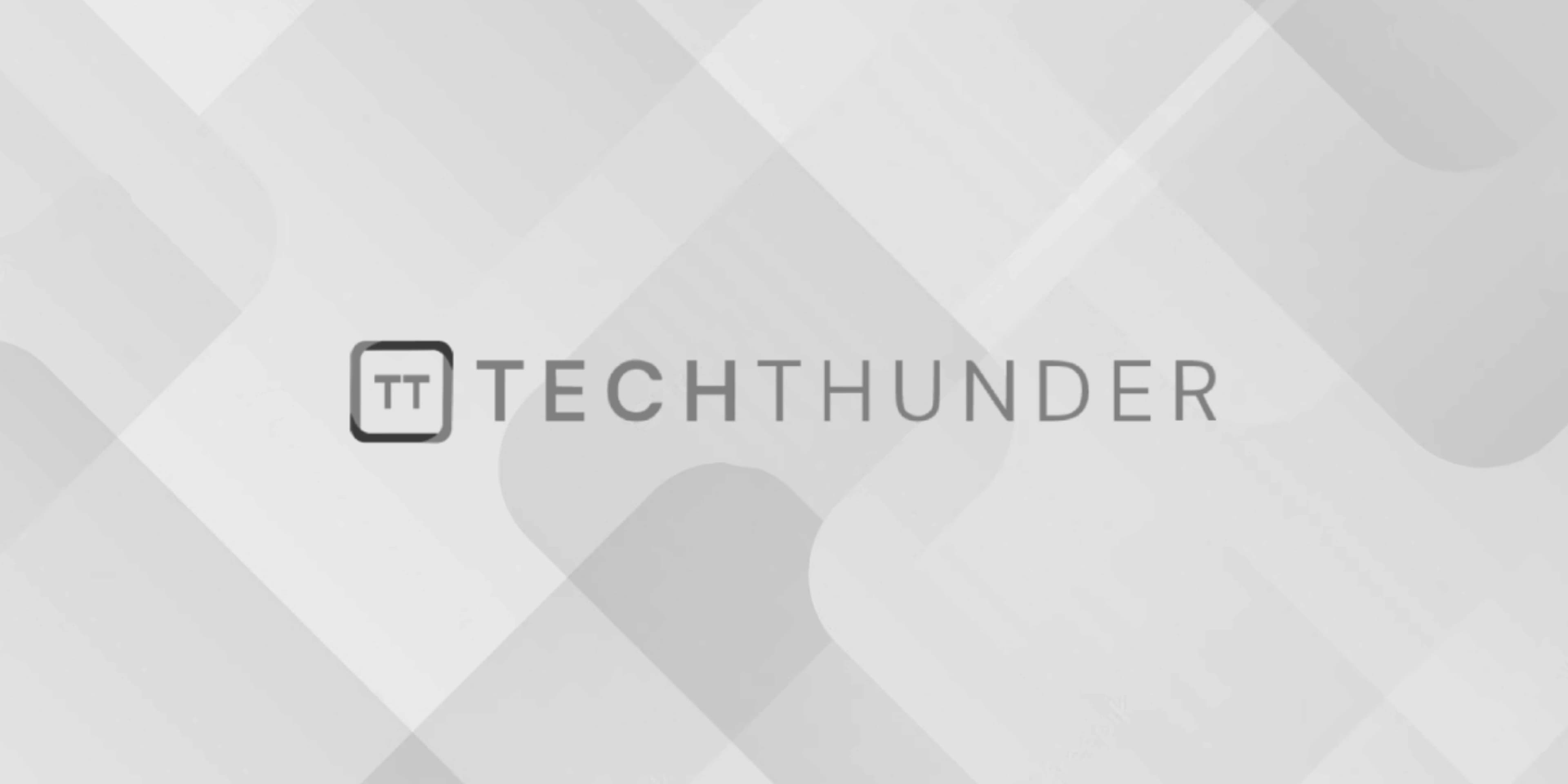
93 views
Implementing HATEOAS for RESTful Services
HATEOAS (Hypermedia as the Engine of Application State) is an important concept in RESTful APIs that provides links in the API responses, allowing clients to discover and navigate related resources dynamically. Spring HATEOAS is a Spring Boot module that makes it easy to implement HATEOAS principles in your API. Here’s how you can implement HATEOAS in your Spring Boot RESTful services:
- Add Spring HATEOAS Dependency:
Include the Spring HATEOAS dependency in yourbuild.gradle
orpom.xml
: For Gradle:
implementation 'org.springframework.boot:spring-boot-starter-hateoas'
For Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-hateoas</artifactId>
</dependency>
- Use Resource and ControllerLinkBuilder:
Spring HATEOAS provides theResource
class to wrap your data and add links to it. TheControllerLinkBuilder
class helps create links.
import org.springframework.hateoas.Resource;
import org.springframework.hateoas.mvc.ControllerLinkBuilder;
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public Resource<User> getUser(@PathVariable Long id) {
User user = userService.getUserById(id);
Resource<User> resource = new Resource<>(user);
// Add self link
resource.add(ControllerLinkBuilder.linkTo(
ControllerLinkBuilder.methodOn(UserController.class).getUser(id)
).withSelfRel());
// Add other relevant links
// ...
return resource;
}
}
- Use EntityModel:
TheEntityModel
class is an alternative toResource
that provides more flexibility. It allows adding links to any object.
import org.springframework.hateoas.EntityModel;
import org.springframework.hateoas.Link;
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public EntityModel<User> getUser(@PathVariable Long id) {
User user = userService.getUserById(id);
Link selfLink = Link.of(
ControllerLinkBuilder.linkTo(UserController.class).slash(id).withSelfRel().getHref()
);
return EntityModel.of(user, selfLink);
}
}
- Enable Hypermedia Support:
Ensure that your main application class is annotated with@EnableHypermediaSupport(type = EnableHypermediaSupport.HypermediaType.HAL)
. This enables HAL (Hypertext Application Language) JSON responses, which include links.
import org.springframework.hateoas.config.EnableHypermediaSupport;
@SpringBootApplication
@EnableHypermediaSupport(type = EnableHypermediaSupport.HypermediaType.HAL)
public class YourApplication {
public static void main(String[] args) {
SpringApplication.run(YourApplication.class, args);
}
}
- Test the API:
When you request the API endpoint, you should receive responses that include links in HAL format.
By following these steps, you’ll be able to implement HATEOAS principles using Spring HATEOAS in your Spring Boot RESTful services, making your API responses more discoverable and navigable for clients.