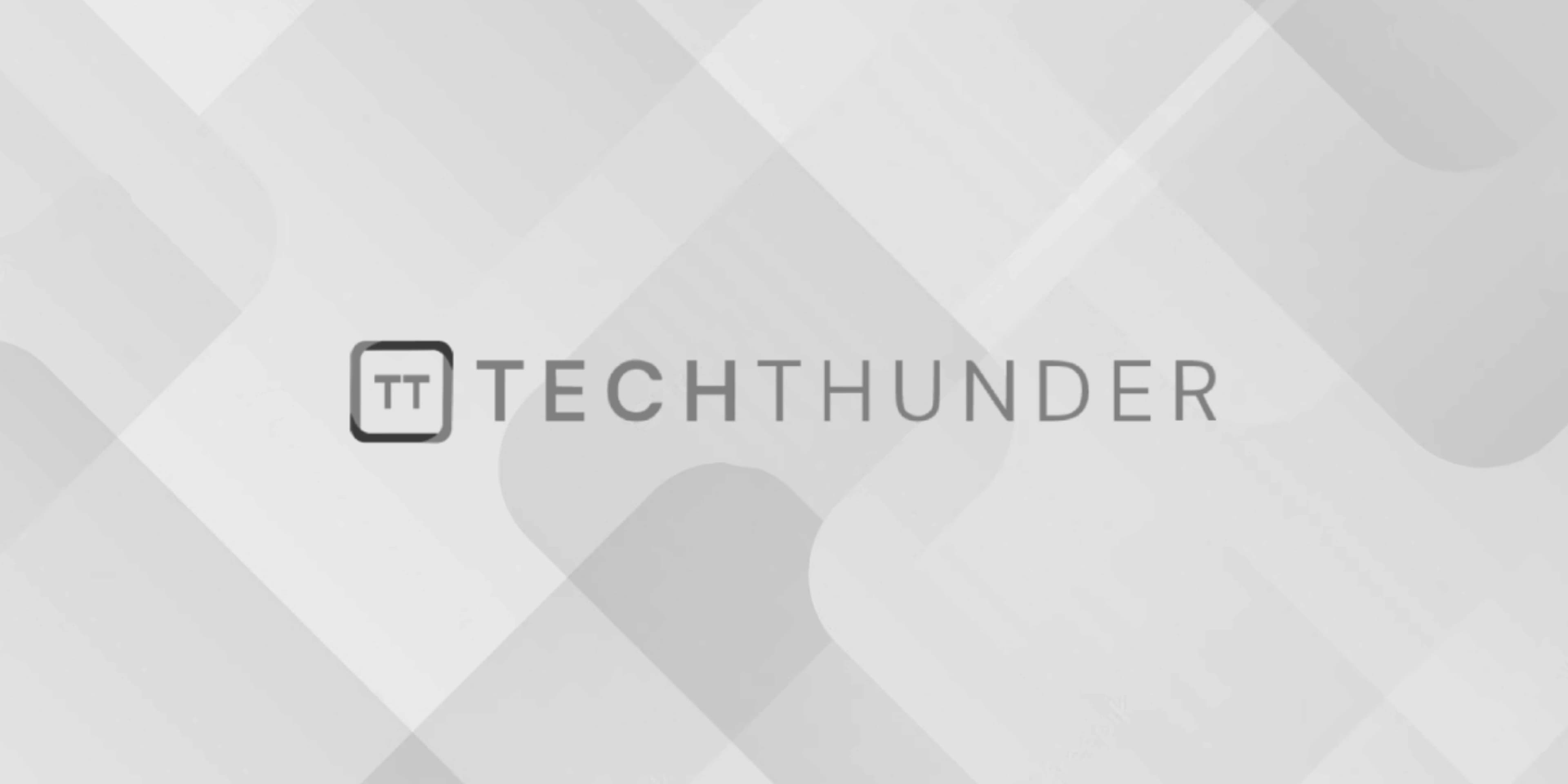
Spring Boot JDBC
Spring Boot with JDBC (Java Database Connectivity) allows you to build Java applications that interact with relational databases using standard JDBC APIs. Spring Boot simplifies the development of database-driven applications by providing auto-configuration and built-in support for JDBC data sources. Here’s how to use Spring Boot with JDBC to create a database-driven application:
Step 1: Create a Spring Boot Project:
You can create a Spring Boot project using Spring Initializr, which is a web-based tool for generating Spring Boot projects. Visit the Spring Initializr website and configure your project by selecting the required dependencies, including “Spring Web” and “Spring Data JDBC” or “Spring Data JPA” if you prefer JPA for data access.
Step 2: Configure Database Connection:
In your application.properties
or application.yml
file, configure the database connection settings, including the database URL, username, and password. Here’s an example for MySQL:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Step 3: Define Entity Classes (Optional):
If you choose to use Spring Data JPA, define entity classes that represent your database tables, as shown in the previous response.
If you prefer to use Spring Data JDBC, you can create simple DTO (Data Transfer Object) classes to map the data from your database tables. You do not need annotations like @Entity
in this case.
Step 4: Create a Repository Interface:
Create a repository interface that extends JdbcRepository
(Spring Data JDBC) or JpaRepository
(Spring Data JPA) to handle CRUD (Create, Read, Update, Delete) operations on the database.
For Spring Data JDBC:
import org.springframework.data.jdbc.repository.query.Query;
import org.springframework.data.repository.CrudRepository;
public interface UserRepository extends CrudRepository<User, Long> {
// Define custom queries or methods here if needed
}
For Spring Data JPA, you can follow the instructions in the previous response.
Step 5: Service Layer (Optional):
Create a service layer to encapsulate business logic and interact with the repositories. The service layer typically contains methods that provide high-level operations on the data.
Step 6: Controller Layer:
Create controller classes to define the RESTful endpoints that interact with your service layer. Use annotations like @RestController
and @RequestMapping
to define the endpoints.
Step 7: Run the Application:
Run your Spring Boot application. Spring Boot will automatically configure the data source, create tables (if specified in the configuration), and expose the RESTful endpoints defined in your controllers.
Step 8: Perform Database Operations:
You can now use your application to perform CRUD operations on the database. Your application will use JDBC (or JPA) to connect to the database, execute SQL queries, and retrieve or manipulate data.
This is a basic setup for using Spring Boot with JDBC. Depending on your project’s requirements, you can further enhance it by adding validation, security, error handling, and more advanced database operations as needed.