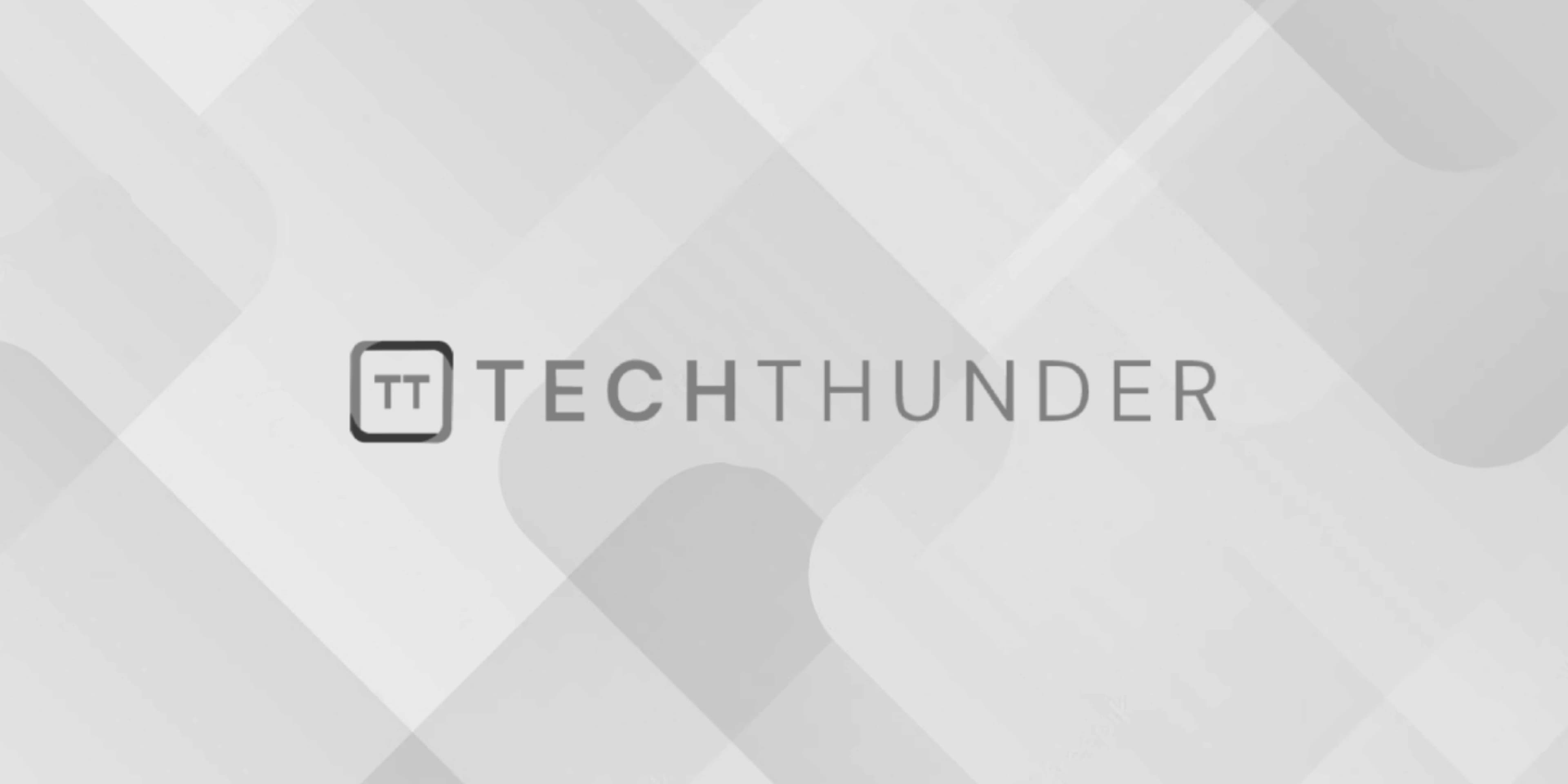
Introduction to RESTful Web Services With Spring Boot
RESTful Web Services are a popular architectural style for building web-based APIs (Application Programming Interfaces) that allow different software applications to communicate over the internet. These APIs are designed to be simple, lightweight, and stateless, making them suitable for a wide range of applications. When building RESTful Web Services with Spring Boot, you leverage the power of the Spring framework to create efficient and scalable APIs. Here’s an introduction to RESTful Web Services with Spring Boot:
1. What is REST?
- REST stands for Representational State Transfer and is an architectural style for designing networked applications. It is characterized by the use of standard HTTP methods (GET, POST, PUT, DELETE) and the concept of resources (represented as URLs) that can be manipulated using these methods.
2. RESTful Principles:
- RESTful APIs follow several principles:
- Stateless: Each request from a client to a server must contain all the information needed to understand and process the request. The server should not store any client state between requests.
- Client-Server: The client and server are separate entities that communicate over a stateless protocol (usually HTTP). This separation allows them to evolve independently.
- Uniform Interface: RESTful APIs have a consistent and uniform interface, making it easy for clients to understand and interact with resources.
- Resource-Based: Resources are the core abstractions in REST. They are identified by URIs (Uniform Resource Identifiers) and can represent data entities, such as users, products, or orders.
- CRUD Operations: Resources can be manipulated using standard CRUD (Create, Read, Update, Delete) operations, mapped to HTTP methods (POST, GET, PUT, DELETE).
- Representation: Resources can have multiple representations, such as JSON or XML, which clients can choose based on their preferences.
3. Spring Boot for RESTful Services:
- Spring Boot is a framework that simplifies the development of Java-based web applications, including RESTful APIs. It provides a wide range of features, including embedded web servers, automatic configuration, and seamless integration with various data sources and security mechanisms.
4. Key Components of a Spring Boot RESTful API:
- When building a RESTful API with Spring Boot, you’ll typically work with the following components:
- Controller: Defines the API endpoints and request handling logic.
- Service: Contains business logic and acts as an intermediary between the controller and data access layer.
- Repository or DAO: Handles data access, retrieval, and storage.
- Model or DTO (Data Transfer Object): Represents the data exchanged between the client and the server.
- HTTP Methods: Map to CRUD operations (GET, POST, PUT, DELETE) for resource manipulation.
- Request and Response Serialization: Converts data between Java objects and JSON or XML representations.
- Spring Boot Annotations: Such as
@RestController
,@RequestMapping
, and@Autowired
, simplify the configuration and implementation of RESTful endpoints.
5. Building and Testing:
- Spring Boot provides tools for rapidly building and testing RESTful APIs. You can use tools like Spring Boot Initializr to generate a project skeleton, and Spring Boot’s embedded web server allows you to run and test your API locally.
6. Securing RESTful APIs:
- RESTful APIs often require security measures. Spring Security can be seamlessly integrated with Spring Boot to add authentication and authorization mechanisms to your API.
7. Versioning and Documentation:
- Versioning is essential to manage changes in your API. Spring Boot supports various versioning strategies. Additionally, you can generate API documentation using tools like Swagger to help clients understand your API.
Spring Boot simplifies the process of building RESTful APIs by providing a streamlined development experience and robust support for REST principles. It has become a popular choice for developing RESTful services due to its ease of use and integration capabilities.