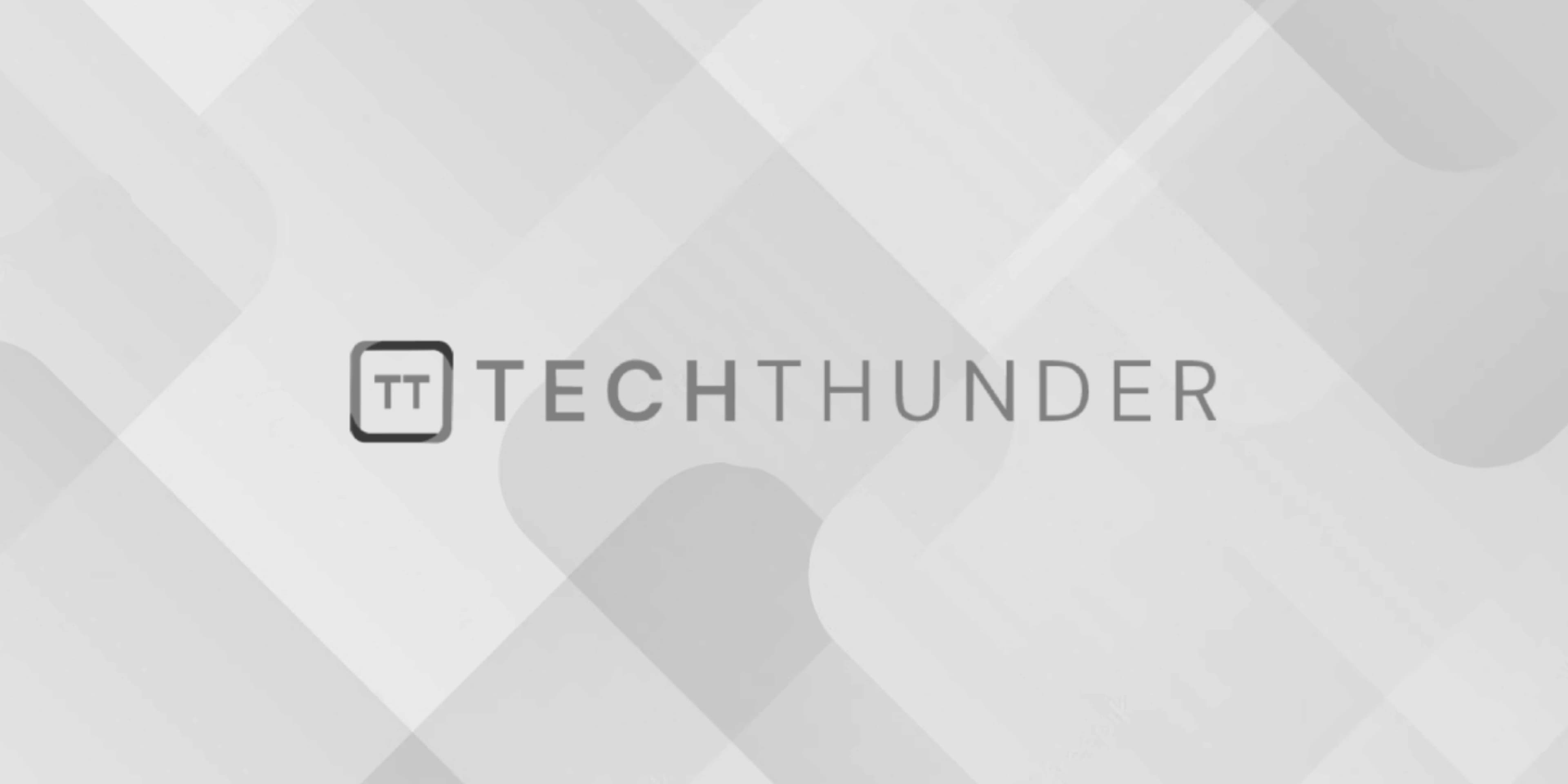
Spring Boot JDBC Example
The simple Spring Boot JDBC example that demonstrates how to create a Spring Boot application that interacts with a MySQL database using JDBC. In this example, we’ll create a simple user management system with basic CRUD (Create, Read, Update, Delete) operations.
Step 1: Create a Spring Boot Project:
You can create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Configure Database Connection:
In your application.properties
or application.yml
file, configure the database connection settings. Replace the values with your database URL, username, and password.
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Step 3: Create a User Entity:
Create a simple User
entity class that represents a user in your application:
public class User {
private Long id;
private String username;
private String email;
// Constructors, getters, and setters
}
Step 4: Create a UserRepository:
Create a repository interface that extends JdbcRepository<User, Long>
to handle database operations for the User
entity.
import org.springframework.data.jdbc.repository.query.Query;
import org.springframework.data.repository.CrudRepository;
public interface UserRepository extends CrudRepository<User, Long> {
}
Step 5: Create a UserService:
Create a service class to encapsulate business logic and interact with the repository:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<User> getAllUsers() {
return (List<User>) userRepository.findAll();
}
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
public User createUser(User user) {
return userRepository.save(user);
}
public void deleteUser(Long id) {
userRepository.deleteById(id);
}
}
Step 6: Create a UserController:
Create a controller class to define the RESTful endpoints:
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping
public List<User> getAllUsers() {
return userService.getAllUsers();
}
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
@PostMapping
public User createUser(@RequestBody User user) {
return userService.createUser(user);
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
userService.deleteUser(id);
}
}
Step 7: Run the Application:
Run your Spring Boot application. Spring Boot will automatically configure the data source and expose the RESTful endpoints defined in your controller.
You can now use tools like Postman or cURL to perform CRUD operations on the /users
endpoint.
This example demonstrates a basic Spring Boot application using JDBC for database access. You can further enhance it by adding validation, error handling, and security as needed for your project.