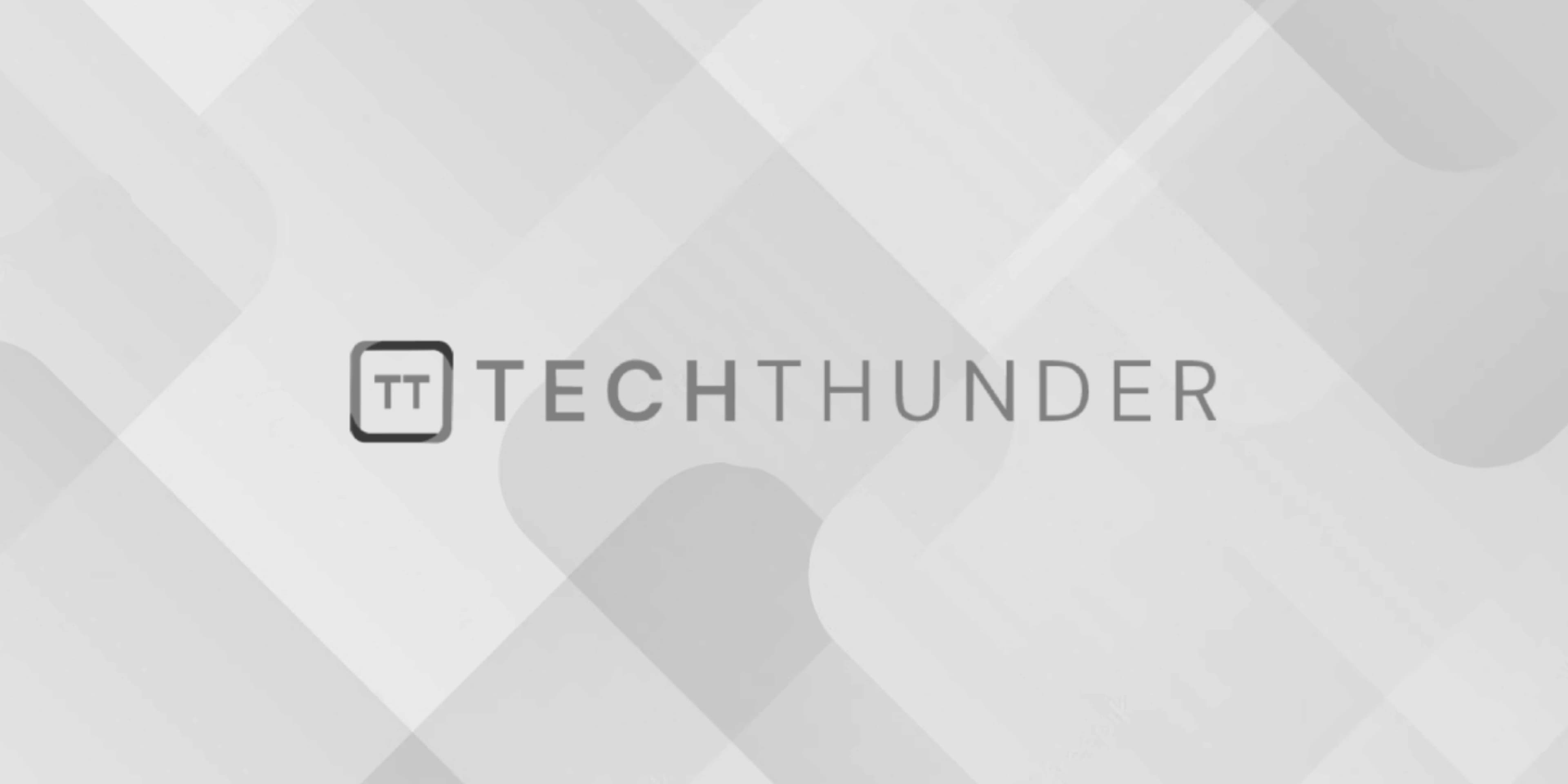
Initializing a RESTful Web Services
Initializing a RESTful Web Service with Spring Boot involves creating a new Spring Boot project and configuring it to handle RESTful API requests. Here are the steps to initialize a basic RESTful Web Service using Spring Boot:
Step 1: Set Up Your Development Environment
Ensure that you have Java and a development environment (e.g., Eclipse, IntelliJ IDEA, or Visual Studio Code) set up on your computer. You’ll also need Spring Boot, which you can add as a dependency in your project.
Step 2: Create a New Spring Boot Project
You can create a Spring Boot project using Spring Initializr, which is a web-based tool for generating Spring Boot projects. Here’s how:
- Visit the Spring Initializr website.
- Configure your project by specifying the following options:
- Project: Choose “Maven Project” or “Gradle Project.”
- Language: Choose “Java.”
- Spring Boot: Choose the desired version.
- Group: Enter a group name for your project.
- Artifact: Enter an artifact name (your project’s name).
- Packaging: Choose “Jar” or “War” (for deploying as a standalone JAR or a web application, respectively).
- Java: Choose your preferred Java version (e.g., 8, 11, 16).
- Dependencies: Add “Spring Web” as a dependency. You can also add other dependencies depending on your project requirements (e.g., Spring Data, Spring Security).
- Click the “Generate” button to download a ZIP file containing your Spring Boot project.
Step 3: Create a REST Controller
In your Spring Boot project, create a REST controller class. This class will define the RESTful endpoints and the logic for handling HTTP requests. Here’s an example of a simple REST controller:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class HelloWorldController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
In this example, we’ve defined a controller with a single endpoint, /api/hello
, which returns a “Hello, World!” message when accessed via a GET request.
Step 4: Run Your Application
You can run your Spring Boot application by executing the main
method in the class containing the main
method (typically named Application
or similar). Alternatively, you can use the mvn spring-boot:run
command if you’re using Maven or ./gradlew bootRun
if you’re using Gradle.
Step 5: Access Your RESTful API
Once your application is running, you can access your RESTful API by making HTTP requests to the defined endpoints. In this example, you can access the “Hello, World!” message by opening a web browser or using tools like curl
or Postman to send a GET request to http://localhost:8080/api/hello
.
That’s it! You’ve initialized a basic RESTful Web Service using Spring Boot. You can now extend your application by adding more endpoints, business logic, database access, and other features as needed for your project.