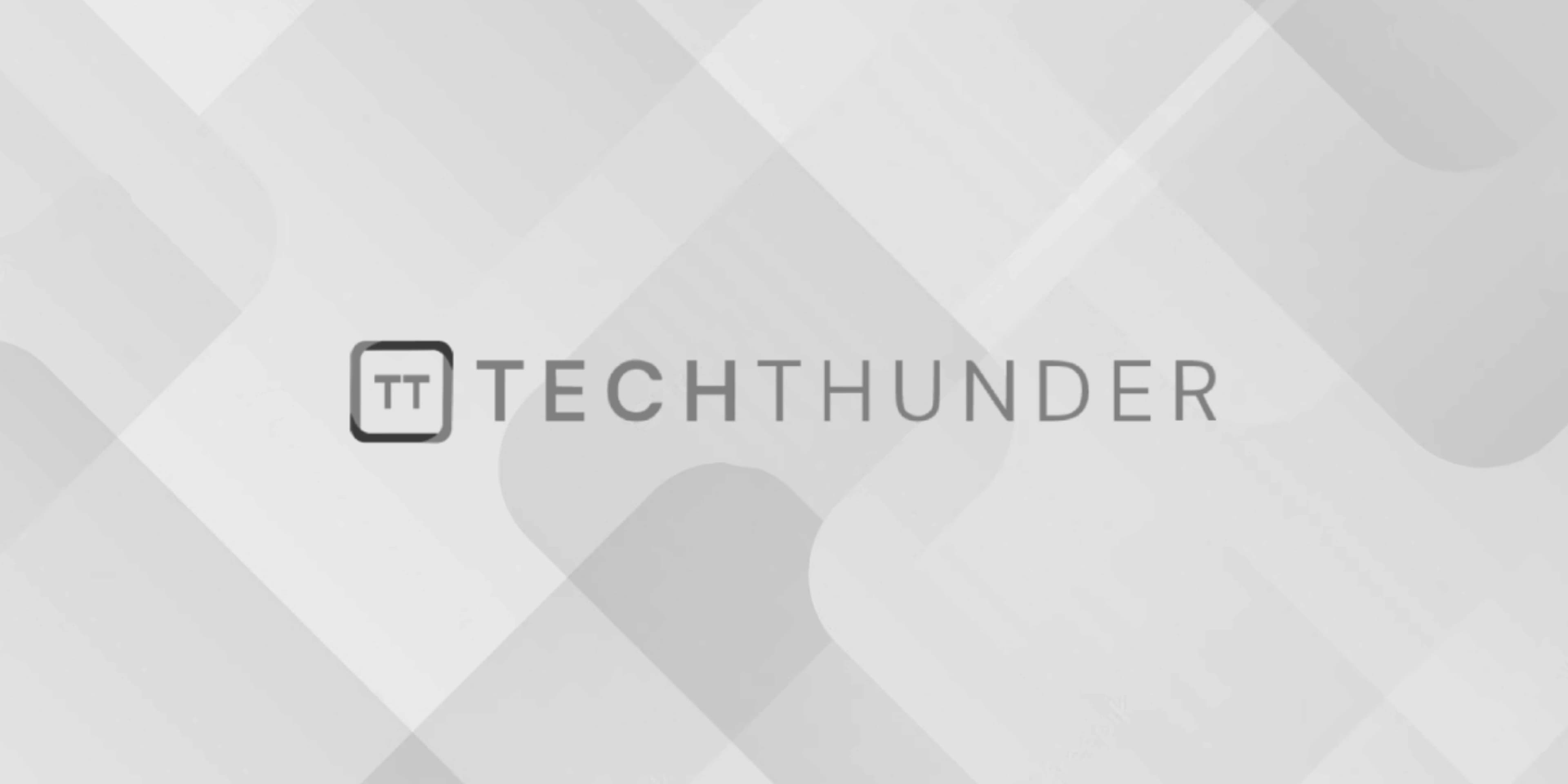
Spring Boot REST Example
Creating a simple RESTful API using Spring Boot is a common task. Here, I’ll provide you with a step-by-step guide to create a basic Spring Boot RESTful web service. We’ll create an endpoint that handles HTTP GET requests and returns a list of items.
Step 1: Create a Spring Boot Project
You can create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Define a Model Class
Create a model class that represents the data you want to expose through your API. For this example, let’s create a Product
class:
public class Product {
private Long id;
private String name;
private double price;
// Constructors, getters, and setters
}
Step 3: Create a Controller
Create a controller class that defines the REST endpoints. In this case, we’ll create an endpoint to retrieve a list of products.
@RestController
@RequestMapping("/api/products")
public class ProductController {
@GetMapping
public List<Product> getAllProducts() {
// Replace this with code to fetch a list of products from a database or elsewhere
List<Product> products = new ArrayList<>();
products.add(new Product(1L, "Product 1", 19.99));
products.add(new Product(2L, "Product 2", 29.99));
return products;
}
}
In this example, we’ve defined a controller with a @GetMapping
annotation that maps to the /api/products
URL. It returns a list of Product
objects.
Step 4: Run Your Application
Run your Spring Boot application. By default, Spring Boot applications usually run on port 8080. You can access your REST API at http://localhost:8080/api/products
.
Step 5: Test Your REST API
You can use tools like curl
, Postman
, or write a simple web client to test your REST API. To test it in a web browser, simply enter the URL http://localhost:8080/api/products
, and you should see a JSON response with a list of products.
Here’s an example of what the JSON response might look like:
[
{
"id": 1,
"name": "Product 1",
"price": 19.99
},
{
"id": 2,
"name": "Product 2",
"price": 29.99
}
]
That’s it! You’ve created a basic Spring Boot RESTful API. This example serves as a starting point, and you can extend it to handle more complex operations, such as creating, updating, and deleting resources, and connect it to a database for data persistence.