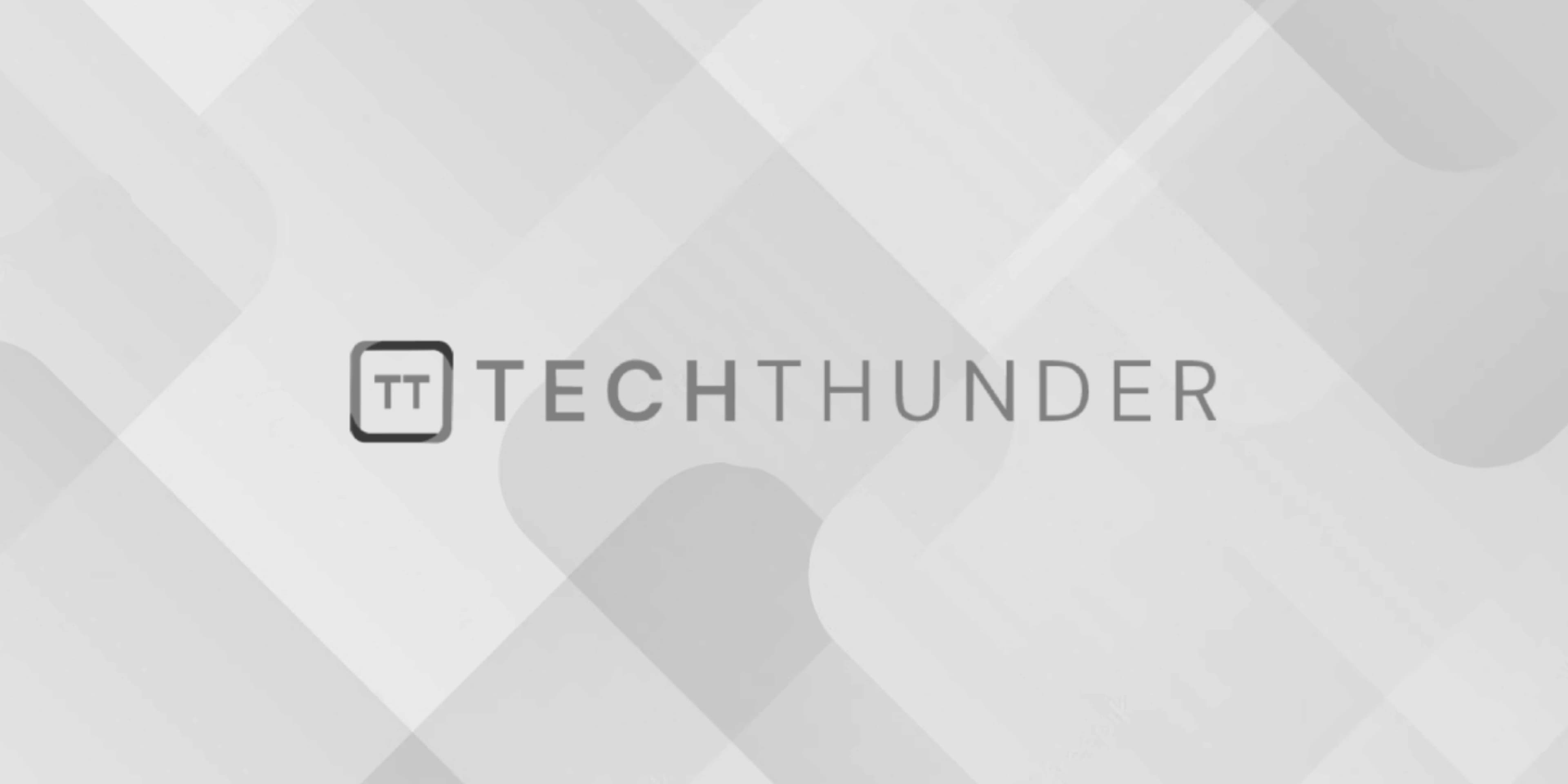
Implementing Static Filtering for RESTful Services
Static filtering for RESTful services involves removing or hiding specific fields from the response data before it’s sent to the client. This is typically done for security reasons or to limit the amount of data sent to the client. In Spring Boot, you can implement static filtering using annotations and Java classes. Here’s how to do it:
1. Create a Java Bean (POJO):
- Start by creating a Java bean (Plain Old Java Object) that represents the data you want to expose through your RESTful service. This bean should have all the fields you need, including the ones you want to filter out.
public class Employee {
private int id;
private String name;
private String department;
// getters and setters
}
2. Add Filtering Annotations:
- Spring Boot provides annotations that allow you to specify which fields should be included or excluded from the response. You can use
@JsonIgnore
to exclude specific fields.
public class Employee {
private int id;
private String name;
@JsonIgnore
private String department;
// getters and setters
}
In this example, the department
field is annotated with @JsonIgnore
, indicating that it should be excluded from the response.
3. Create a Resource Class:
- Create a resource class that will serve as your RESTful controller. This class will contain methods for handling HTTP requests and returning JSON responses.
@RestController
public class EmployeeResource {
@GetMapping("/employees/{id}")
public Employee retrieveEmployee(@PathVariable int id) {
// Create and return an Employee object
Employee employee = new Employee();
employee.setId(id);
employee.setName("John Doe");
employee.setDepartment("HR");
return employee;
}
}
4. Test the Endpoint:
- When you access the
/employees/{id}
endpoint, it will return anEmployee
object as JSON, but thedepartment
field will be excluded from the response due to the@JsonIgnore
annotation.
5. Customize for Multiple Endpoints:
- You can use the
@JsonIgnore
annotation on multiple fields in different beans or endpoints to control which fields are filtered out for each endpoint.
Static filtering is useful when you have fields that should always be hidden or excluded from the response, regardless of the client’s request. However, it’s important to note that this approach affects all clients of the API, and there’s no way for clients to request the excluded fields. If you need dynamic or client-specific filtering, you may consider implementing dynamic filtering using request parameters or other techniques.