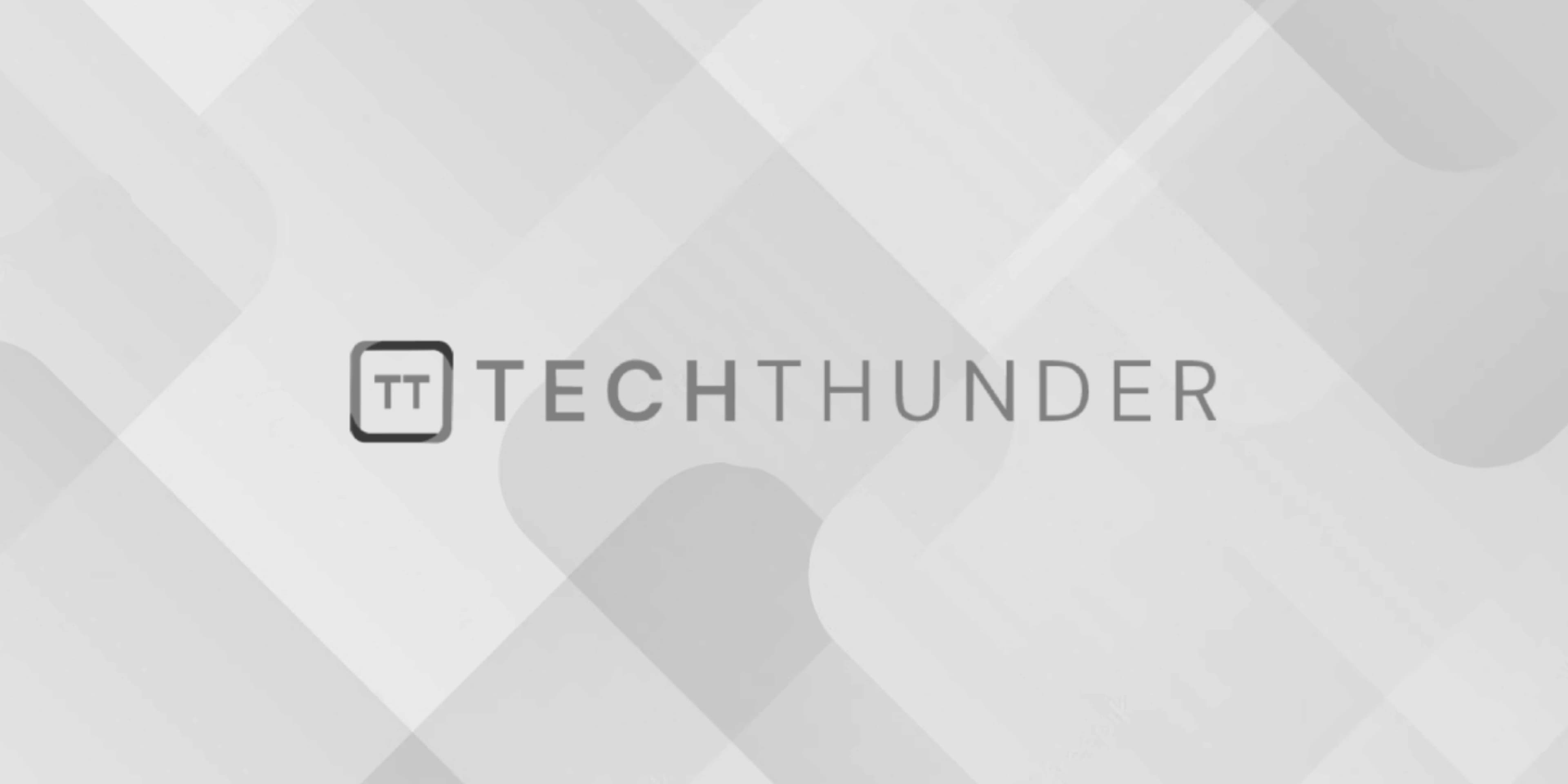
Updating GET Methods on User Resource to Use JPA
To update the GET
methods in your Spring Boot RESTful service to use JPA for data retrieval, you need to modify your controller methods to interact with the JPA repository. Here’s how you can do that:
Assuming you have a User
entity and a UserRepository
interface as described in the previous responses, here’s how you can update your UserController
to use JPA for retrieving user data:
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public List<User> getAllUsers() {
return userRepository.findAll();
}
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
Optional<User> user = userRepository.findById(id);
return user.map(ResponseEntity::ok)
.orElse(ResponseEntity.notFound().build());
}
// ... Other methods for creating, updating, and deleting users ...
}
In the code above:
- The
@Autowired
annotation injects theUserRepository
bean into the controller. - The
getAllUsers()
method usesuserRepository.findAll()
to retrieve all users from the database. - The
getUserById(Long id)
method usesuserRepository.findById(id)
to retrieve a user by their ID from the database. It then uses theOptional
returned byfindById
to either return anok
response with the user data or anotFound
response if the user doesn’t exist.
By using the JPA repository methods (findAll()
and findById(id)
) provided by Spring Data JPA, you can easily retrieve data from your database without writing explicit SQL queries. This abstraction simplifies your code and ensures that you’re adhering to good practices for interacting with your database.
Remember to configure your database connection and entity classes properly to ensure that JPA can map your data between the Java objects and the database tables.