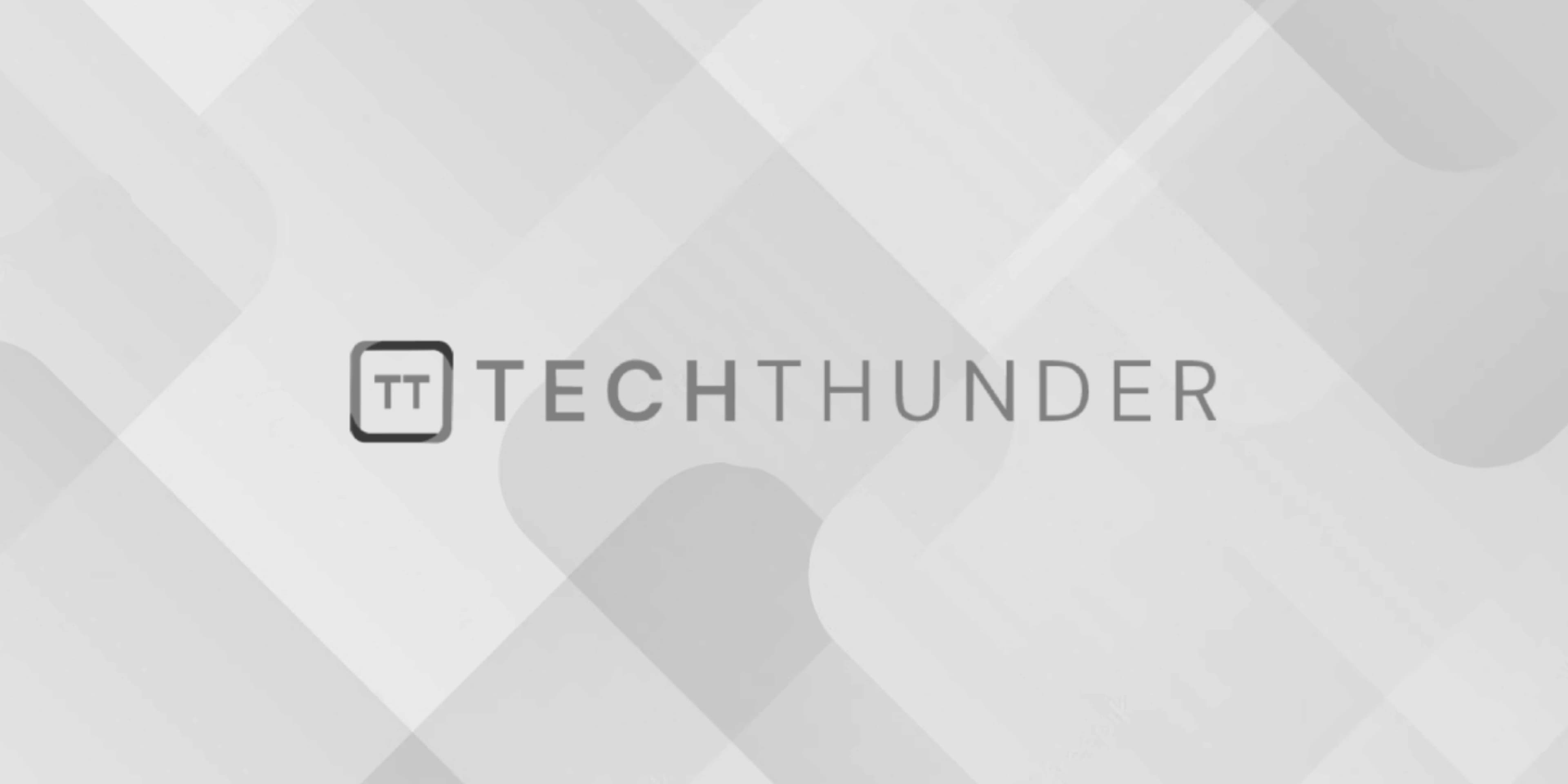
Run Spring Boot Application
To run a Spring Boot application, follow these steps:
Step 1: Create a Spring Boot Project
If you haven’t already created a Spring Boot project, you can do so using Spring Initializr or your preferred development environment. Make sure your project includes the necessary dependencies for your application, such as Spring Web, Spring Data JPA, and any other dependencies you may need.
Step 2: Write Your Application Code
Write the code for your Spring Boot application. This includes creating controllers, services, repositories, and any other components that your application requires. Make sure your application is properly configured with any required properties, database settings, and application-specific logic.
Here’s an example of a simple Spring Boot application:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Step 3: Build Your Project
If you’re using a build tool like Maven or Gradle, you’ll need to build your project to compile your code and package it into a runnable JAR or WAR file. Use the following commands:
For Maven:
mvn clean install
For Gradle:
./gradlew build
Step 4: Run Your Spring Boot Application
Once your project is built successfully, you can run your Spring Boot application. There are several ways to do this:
- Using the Command Line: If you’re using Maven, you can run your application with the following command:
mvn spring-boot:run
If you’re using Gradle, you can run your application with the following command:
./gradlew bootRun
- Using an IDE: Most modern integrated development environments (IDEs) have built-in support for running Spring Boot applications. Simply find the main class of your application (the one annotated with
@SpringBootApplication
) and run it as a Java application from your IDE. - Running the Packaged JAR: If you’ve built your project and created a JAR file (e.g.,
my-application.jar
), you can run it using thejava -jar
command:
java -jar my-application.jar
- Using Docker (Optional): If you want to containerize your Spring Boot application, you can create a Docker image and run it inside a Docker container. This requires Docker to be installed on your system.
- Create a Dockerfile in your project’s root directory:
FROM openjdk:11-jre-slim EXPOSE 8080 COPY target/my-application.jar app.jar ENTRYPOINT ["java", "-jar", "app.jar"]
- Build the Docker image:
docker build -t my-application .
- Run the Docker container:
docker run -p 8080:8080 my-application
Step 5: Access Your Application
Once your Spring Boot application is running, you can access it by opening a web browser and navigating to the specified URL. By default, Spring Boot applications typically run on http://localhost:8080
, but this can vary depending on your application’s configuration.
Congratulations! Your Spring Boot application is now up and running. You can start using and testing your application’s functionality.