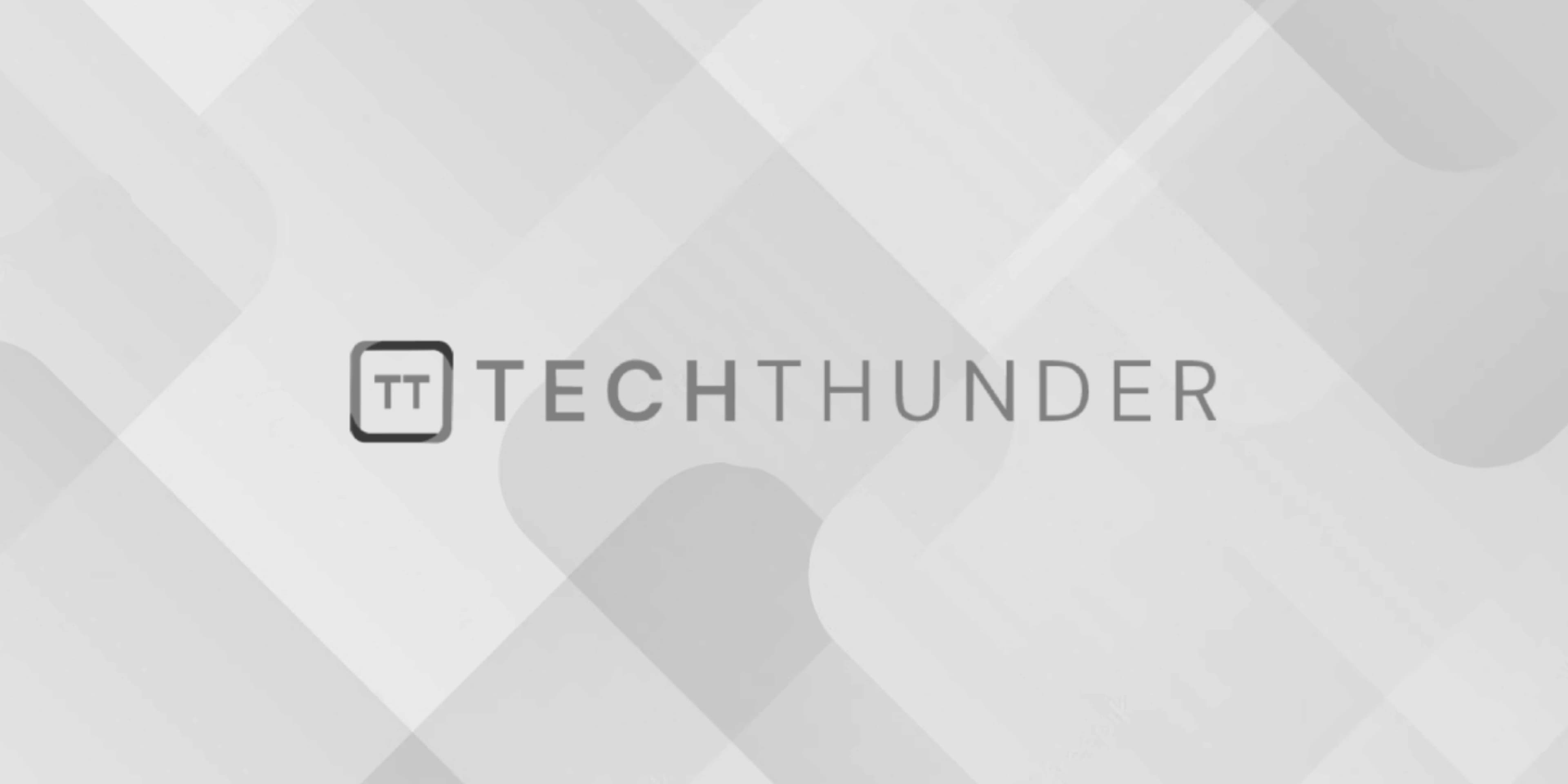
301 views
Richardson Maturity Model
Implementing the Richardson Maturity Model for RESTful APIs in Spring Boot involves structuring your API design according to the different levels of the model. Let’s look at how you can apply the Richardson Maturity Model concepts within a Spring Boot application:
- Level 0 – The Swamp of POX:
At this level, the API is essentially using HTTP as a transport mechanism, often relying on a single endpoint and not leveraging HTTP methods or proper resource identification. In Spring Boot, this might mean creating a single endpoint that handles all actions using HTTP POST requests. This approach is not recommended, as it doesn’t leverage the full capabilities of HTTP. - Level 1 – Resources:
This level introduces the concept of resources and resource identifiers (URIs). In Spring Boot, you would:
- Define multiple endpoints for different resources, like
/users
,/orders
, etc. - Use the appropriate HTTP methods for different actions, such as GET, POST, PUT, and DELETE.
- Implement controllers for each resource type to handle requests and perform actions on resources.
- Level 2 – HTTP Verbs:
At this level, you make better use of HTTP methods, each method corresponding to a specific action on a resource. In Spring Boot, you would:
- Create dedicated methods within your controllers for each HTTP method (GET, POST, PUT, DELETE).
- Utilize the
@GetMapping
,@PostMapping
,@PutMapping
, and@DeleteMapping
annotations to map methods to HTTP endpoints. - Implement appropriate business logic for each method to handle the corresponding action.
- Level 3 – Hypermedia Controls (HATEOAS):
This level adds hypermedia controls to your API, allowing clients to discover resources and their capabilities dynamically. In Spring Boot, you would:
- Include hypermedia links in your response payloads using libraries like Spring HATEOAS.
- Define resource representations as classes that extend
ResourceSupport
to include links. - Use
Link
objects to add links to related resources and actions. - Provide clients with navigation options through the links embedded in the responses.
Here’s a simple example of how you might implement the Richardson Maturity Model levels using Spring Boot:
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{userId}")
public ResponseEntity<User> getUser(@PathVariable Long userId) {
User user = userService.getUserById(userId);
// Create self link and additional links
Link selfLink = linkTo(UserController.class).slash(userId).withSelfRel();
user.add(selfLink);
return ResponseEntity.ok(user);
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.createUser(user);
// Create self link
Link selfLink = linkTo(UserController.class).slash(createdUser.getId()).withSelfRel();
createdUser.add(selfLink);
return ResponseEntity.created(selfLink.toUri()).body(createdUser);
}
// Similar methods for PUT and DELETE
}
In this example, linkTo
and withSelfRel
are methods from Spring HATEOAS used to create hypermedia links. By following these patterns, you can gradually progress through the Richardson Maturity Model levels while designing your Spring Boot RESTful API.