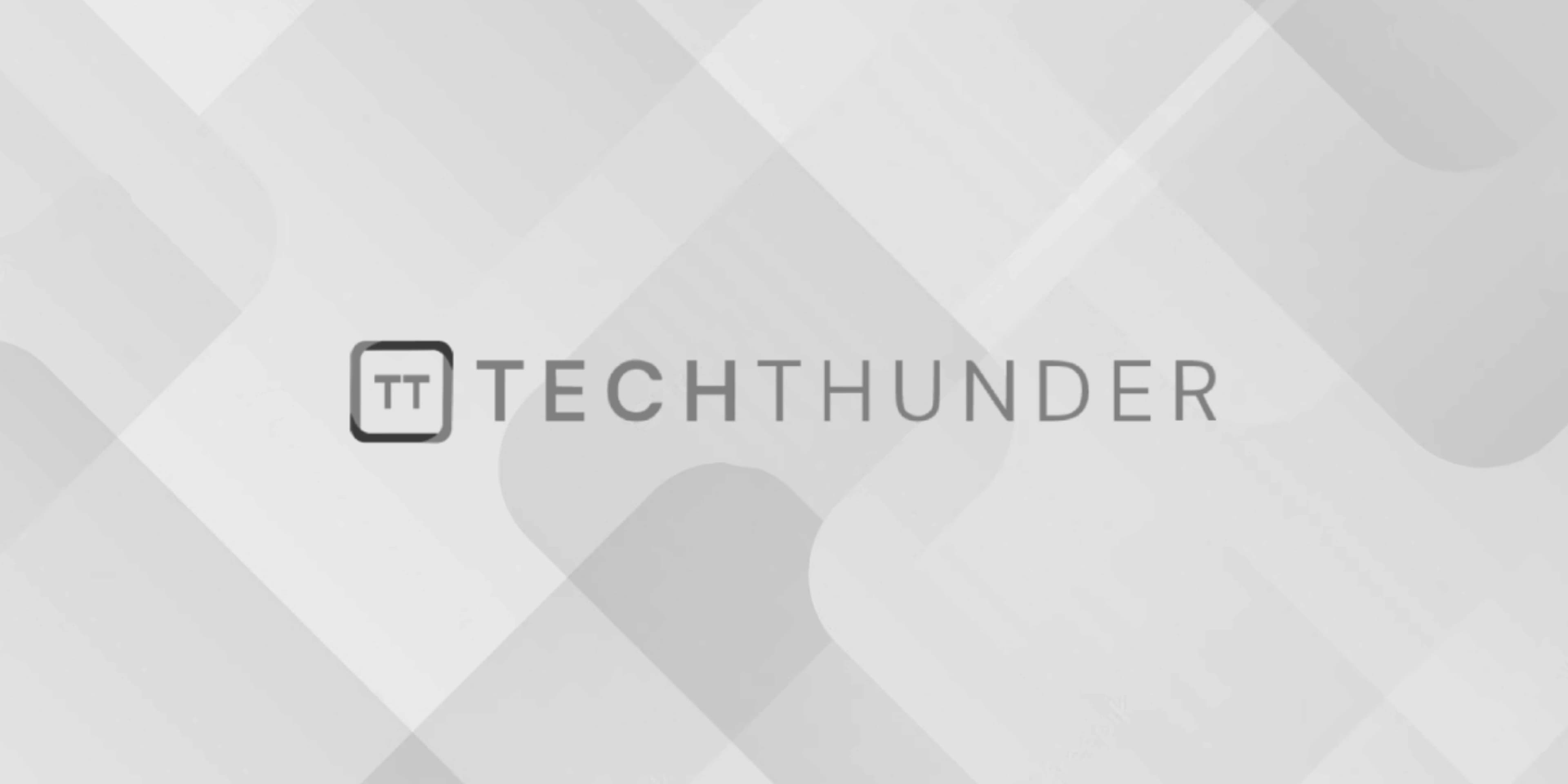
119 views
Implementing DELETE Method to Delete a User Resource
To implement the DELETE
method to delete a user resource in a Spring Boot application, you can follow these steps:
- Create a Controller:
Create a controller class that handles the RESTful endpoints for managing users. Inject theUserService
(assuming you have a service layer) to handle the deletion logic.
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
// Other methods for getting, creating, and updating users
@DeleteMapping("/{userId}")
public ResponseEntity<Void> deleteUser(@PathVariable Long userId) {
boolean deleted = userService.deleteUser(userId);
if (deleted) {
return ResponseEntity.noContent().build();
} else {
return ResponseEntity.notFound().build();
}
}
}
- Create a Service:
Create a service class to handle the business logic for deleting users. Inject theUserRepository
to interact with the database.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public boolean deleteUser(Long userId) {
Optional<User> user = userRepository.findById(userId);
if (user.isPresent()) {
userRepository.delete(user.get());
return true;
} else {
return false;
}
}
// Other methods for getting, creating, and updating users
}
- Testing:
You can now test theDELETE
endpoint by sending aDELETE
request to the URL pattern/users/{userId}
. For example, usingcurl
:
curl -X DELETE http://localhost:8080/users/1
This would delete the user with ID 1
.
Remember to handle proper error cases, validation, and security considerations based on your application’s requirements. In the code above, a boolean
is returned from the deleteUser
method to indicate whether the deletion was successful. You can adjust this approach to return more detailed responses as needed.