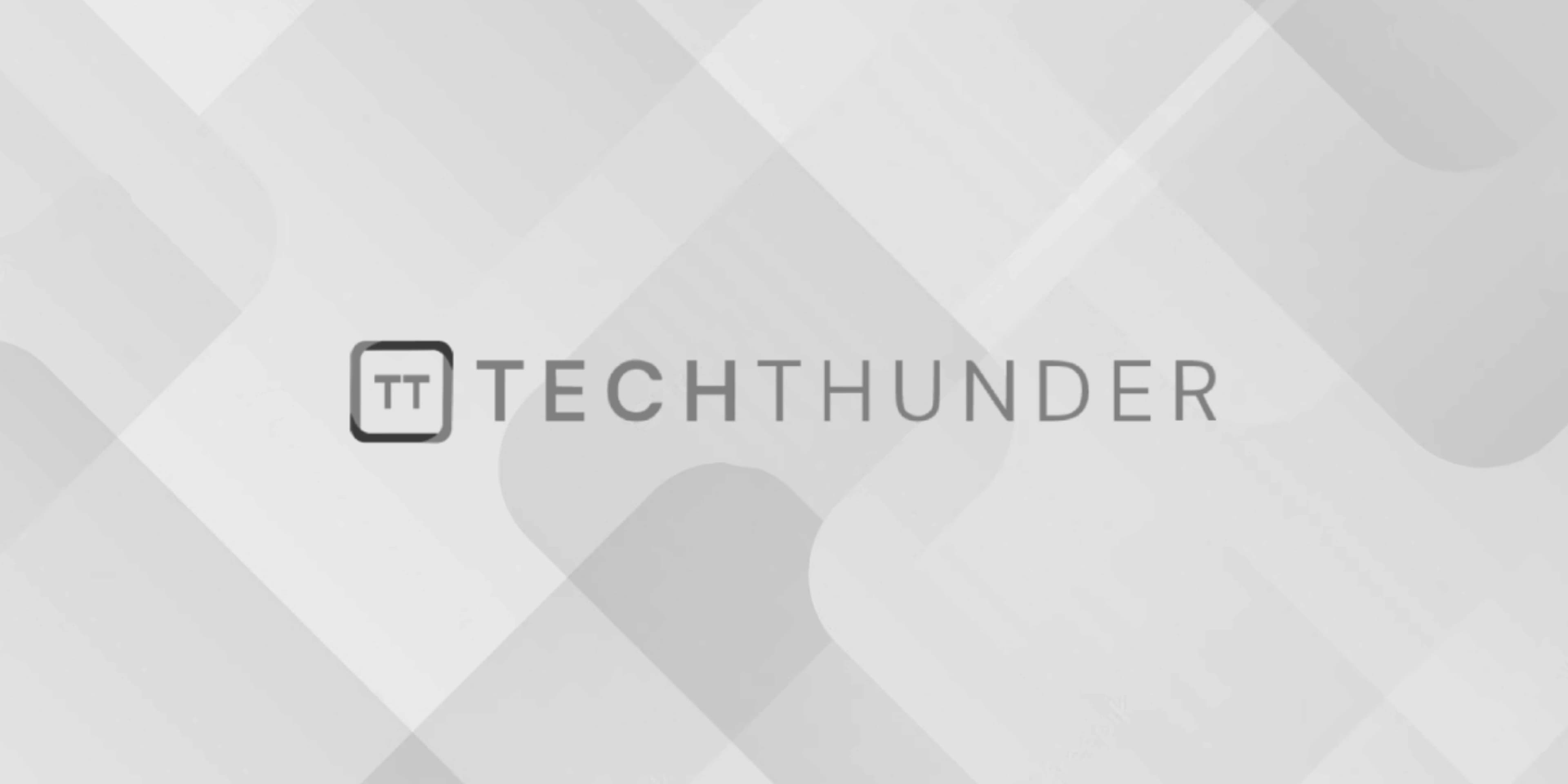
Spring Boot H2 Database
Spring Boot with the H2 database is a great combination for developing and testing Java applications that require an embedded, in-memory database. H2 is particularly useful for rapid prototyping, unit testing, and development scenarios where you need a lightweight, easily configurable database. Here’s how to set up a Spring Boot application with an H2 database:
Step 1: Create a Spring Boot Project:
You can create a Spring Boot project using Spring Initializr or your preferred development environment. When configuring your project, select the “H2” dependency from the available options.
Step 2: Configure H2 Database in application.properties
or application.yml
:
Spring Boot provides auto-configuration for H2 when it detects the H2 dependency. You typically don’t need to specify a data source URL, username, or password for H2 because it runs in-memory by default. However, you can customize the H2 configuration if needed.
For an in-memory H2 database, your application.properties
or application.yml
can look like this:
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
# H2 Console configuration (optional)
spring.h2.console.enabled=true
spring.h2.console.path=/h2-console
In this configuration:
jdbc:h2:mem:testdb
specifies an in-memory H2 database named “testdb.”org.h2.Driver
is the JDBC driver class for H2.sa
is the default username for H2.password
is the password for H2.
The spring.h2.console
properties enable the H2 console, which provides a web-based interface to interact with the database. It’s helpful for debugging and exploring your data during development. The /h2-console
path is where you can access the H2 console.
Step 3: Create Entity Classes:
Create entity classes that represent your application’s data model. Annotate these classes with JPA annotations to define the database schema.
Step 4: Create Repositories:
Create repository interfaces that extend JpaRepository
or similar Spring Data interfaces to handle CRUD operations on your entities.
Step 5: Create Services and Controllers:
Create service and controller classes to implement business logic and define RESTful endpoints, as described in previous responses.
Step 6: Run the Application:
Run your Spring Boot application. Spring Boot will automatically configure the H2 database and expose the RESTful endpoints defined in your controllers.
Step 7: Access the H2 Console (Optional):
If you enabled the H2 console in your configuration, you can access it by opening a web browser and navigating to the URL specified in the spring.h2.console.path
property (e.g., http://localhost:8080/h2-console
if your application runs on port 8080).
Step 8: Perform CRUD Operations:
You can use tools like Postman or cURL to perform CRUD operations on your application’s endpoints, and the data will be stored in the H2 in-memory database.
Remember that the H2 database is for development and testing purposes. For production scenarios, you would typically configure a more robust database like MySQL, PostgreSQL, or others.
This setup demonstrates a basic Spring Boot application with an embedded H2 database. You can customize it further by adding validation, error handling, and security features as needed for your project.