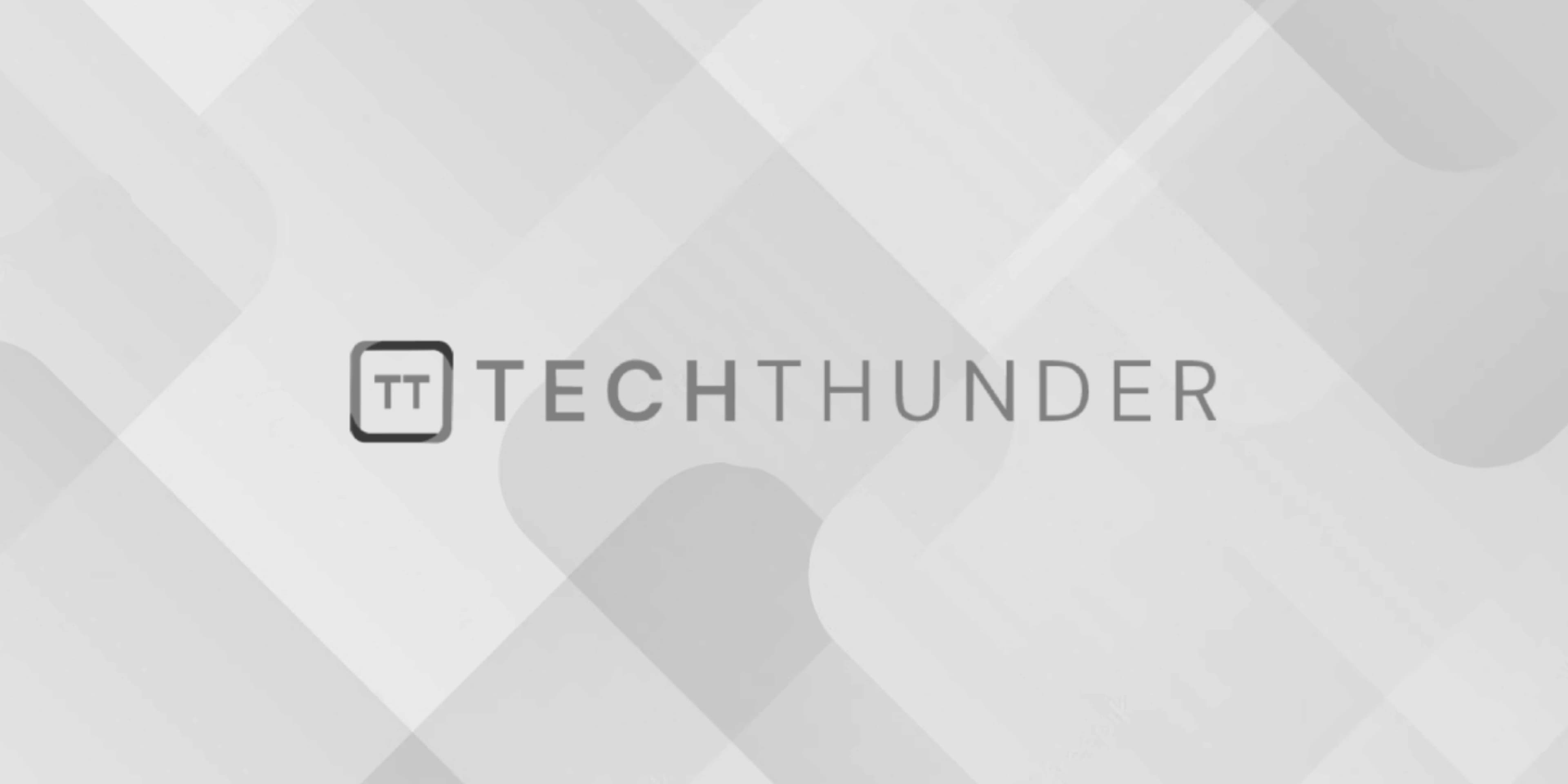
Spring Boot EhCaching
Spring Boot allows you to easily integrate Ehcache as a caching provider in your applications. Ehcache is a popular in-memory caching library that provides various caching strategies, eviction policies, and supports distributed caching. Here’s a step-by-step guide on how to set up Ehcache in a Spring Boot application:
Step 1: Create a Spring Boot Project:
You can create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Add Dependencies:
In your pom.xml
(if you’re using Maven) or build.gradle
(if you’re using Gradle), add the following dependencies to include Ehcache and the Spring Boot starter for Ehcache:
For Maven:
<dependencies>
<!-- Spring Boot Starter for Ehcache -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<!-- Ehcache 3.x -->
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.0</version> <!-- Use the latest version -->
</dependency>
</dependencies>
For Gradle:
dependencies {
// Spring Boot Starter for Ehcache
implementation 'org.springframework.boot:spring-boot-starter-cache'
// Ehcache 3.x
implementation 'org.ehcache:ehcache:3.9.0' // Use the latest version
}
Step 3: Enable Caching in Your Application:
In your Spring Boot application’s main class, annotate it with @EnableCaching
to enable caching.
@SpringBootApplication
@EnableCaching
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Step 4: Configure Ehcache:
Create an Ehcache configuration file (e.g., ehcache.xml
) in the src/main/resources
directory to specify the cache settings. Here’s a basic example:
<config xmlns="http://www.ehcache.org/v3">
<cache alias="myCache">
<resources>
<heap unit="entries">100</heap> <!-- Max entries in cache -->
</resources>
</cache>
</config>
Step 5: Configure Caching in application.properties
or application.yml
:
Specify the location of your Ehcache configuration file in your application.properties
or application.yml
file:
For application.properties
:
spring.cache.type=ehcache
spring.cache.ehcache.config=classpath:ehcache.xml
For application.yml
:
spring:
cache:
type: ehcache
ehcache:
config: classpath:ehcache.xml
Step 6: Use Caching in Your Service:
In your service class, annotate the methods you want to cache using @Cacheable
. Specify the cache name from your Ehcache configuration.
@Service
public class MyService {
@Cacheable("myCache")
public String getCachedData(String key) {
// Expensive operation to fetch data
// This result will be cached
return fetchExpensiveData(key);
}
}
Step 7: Test the Caching:
Run your Spring Boot application and test the caching behavior by making requests to the cached method. The data will be fetched and cached upon the first request and then served from the cache for subsequent requests with the same arguments.
Ehcache provides various advanced features such as distributed caching and configuration options for eviction policies. You can further customize your Ehcache configuration to suit your application’s specific caching requirements.
This setup demonstrates a basic Spring Boot application using Ehcache as the caching provider. You can extend this to implement more complex caching strategies and optimize your application’s performance.