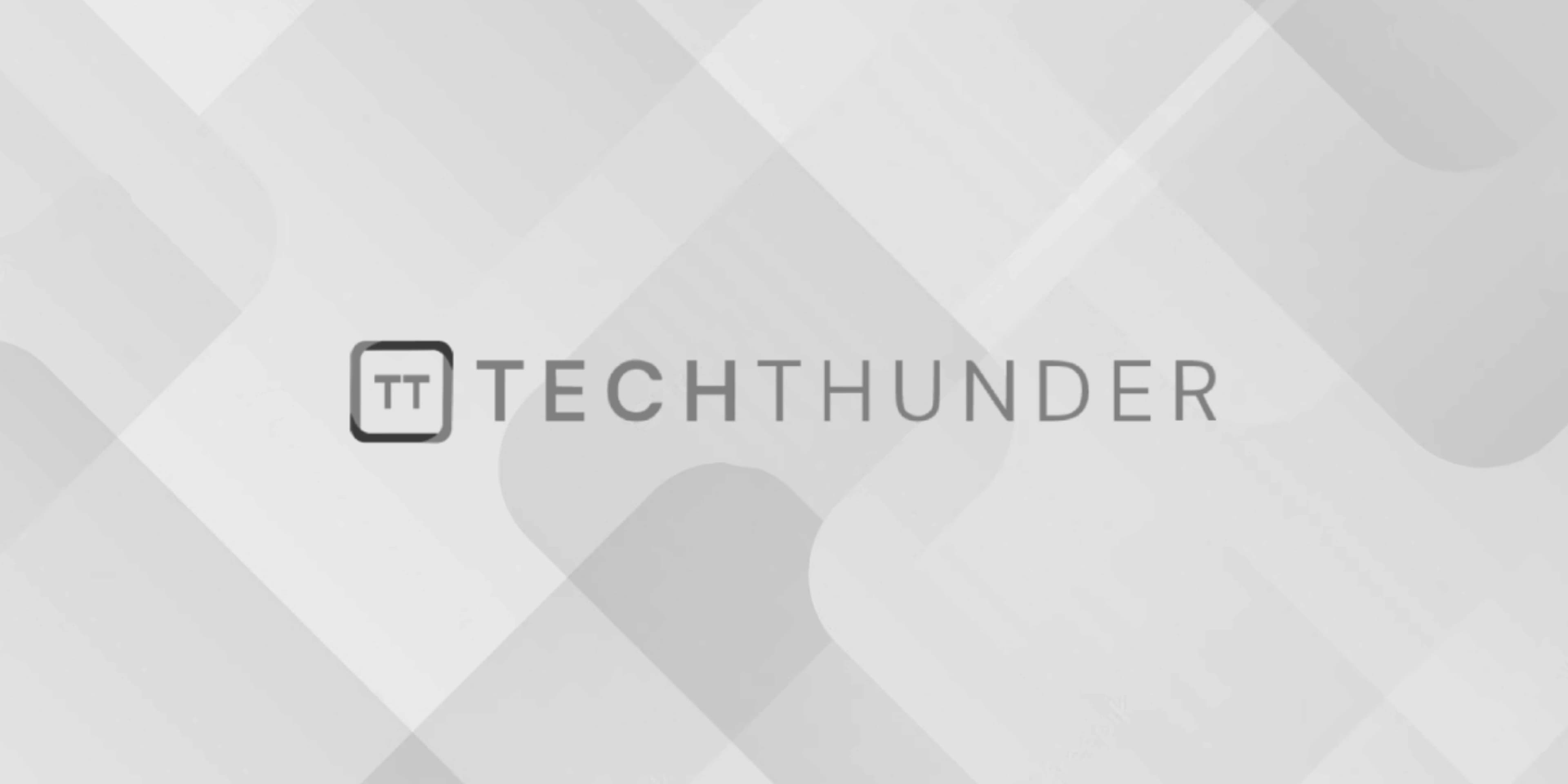
Implementing the POST Method to create User Resource
To implement the POST method to create a user resource in a Spring Boot application, you need to create a RESTful endpoint that accepts HTTP POST requests, processes the incoming data, and stores the new user resource. Here’s a step-by-step guide to implementing this functionality:
Step 1: Create a User Entity Class:
Start by defining a User
entity class that represents the user data you want to store. This class typically includes attributes such as id
, name
, email
, and any other relevant information.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Constructors, getters, and setters
}
Make sure to annotate the class with @Entity
to mark it as a JPA entity and define the primary key field and its generation strategy using @Id
and @GeneratedValue
.
Step 2: Create a UserRepository:
Create a repository interface that extends Spring Data JPA’s JpaRepository
to handle CRUD operations for the User
entity.
public interface UserRepository extends JpaRepository<User, Long> {
}
Step 3: Create a UserController:
Create a controller class to define the RESTful endpoints for managing user resources, including the POST method for creating users.
@RestController
@RequestMapping("/users")
public class UserController {
private final UserRepository userRepository;
@Autowired
public UserController(UserRepository userRepository) {
this.userRepository = userRepository;
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User savedUser = userRepository.save(user);
return new ResponseEntity<>(savedUser, HttpStatus.CREATED);
}
}
In this controller:
- We use the
@RestController
and@RequestMapping
annotations to define a base path for all user-related endpoints (e.g.,/users
). - The
UserController
constructor is autowired with aUserRepository
bean for data access. - The
createUser
method is annotated with@PostMapping
to handle HTTP POST requests. It accepts aUser
object in the request body (received as JSON) and saves it to the database using theuserRepository
. It then returns the saved user object in the response with a201 Created
status code.
Step 4: Configure Database and Spring Data JPA:
Ensure that your application.properties or application.yml file contains database configuration properties, such as the database URL, username, and password. Also, configure Spring Data JPA by adding the @EnableJpaRepositories
annotation to your main application class or a configuration class.
Step 5: Test the POST Endpoint:
You can now test the POST endpoint by sending POST requests with user data (in JSON format) to the /users
endpoint. For example:
POST /users
Content-Type: application/json
{
"name": "John Doe",
"email": "[email protected]"
}
The server will create a new user resource in the database and respond with the created user object.
That’s it! You’ve implemented the POST method to create a user resource in your Spring Boot application. You can further enhance this functionality by adding validation, error handling, and additional endpoints to manage users.