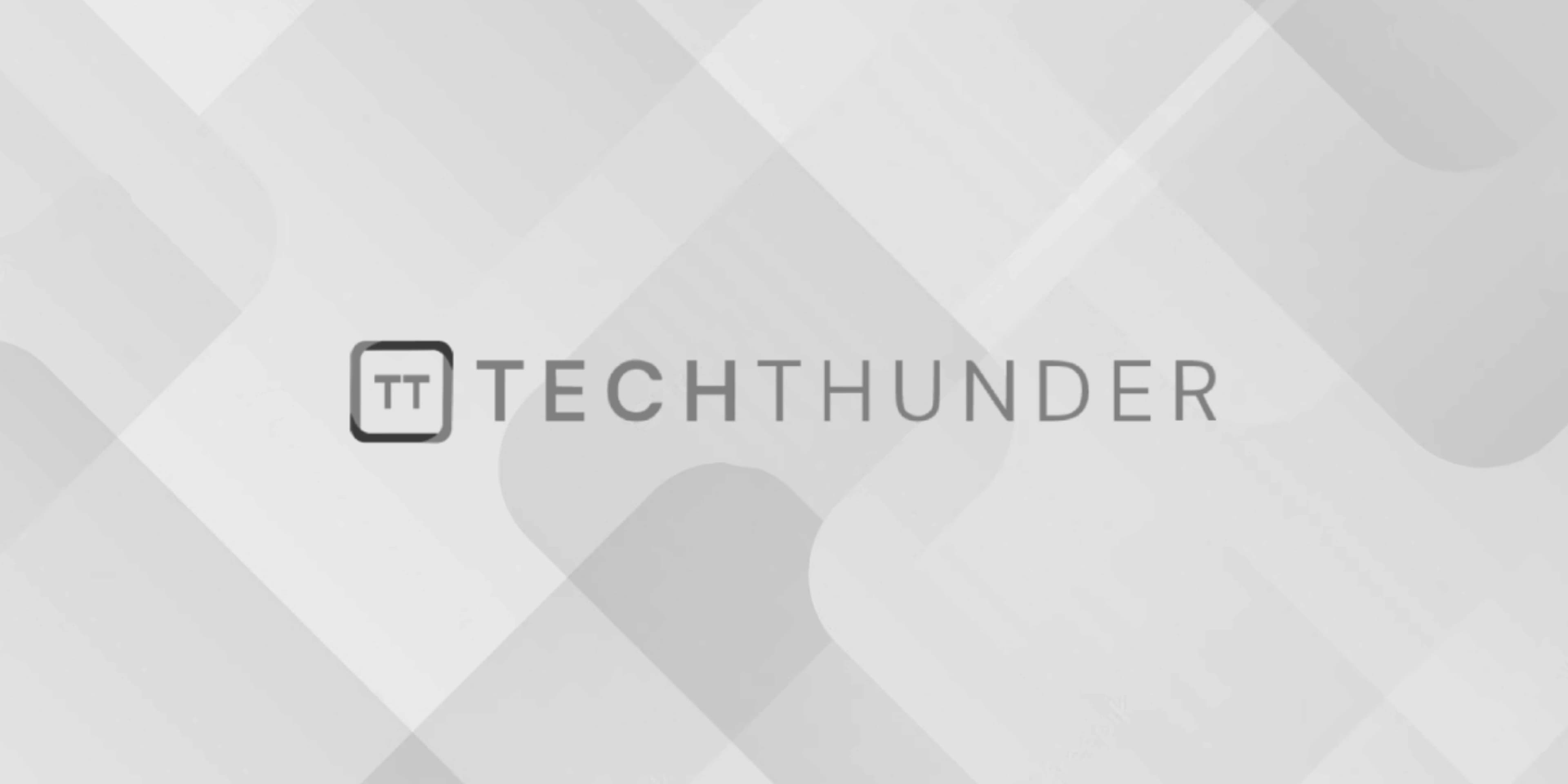
Enhancing Swagger Documentation with Custom Annotations
Enhancing Swagger documentation with custom annotations allows you to provide additional information and instructions about your API endpoints, parameters, responses, and other elements beyond what’s covered by the basic OpenAPI Specification (OAS) or Swagger documentation. Custom annotations are especially useful when you want to add context, descriptions, or metadata that are specific to your API. Here’s how you can enhance Swagger documentation with custom annotations:
- Select a Framework or Library:
- Choose a programming language and web framework that supports Swagger and custom annotations. Many popular frameworks have extensions or libraries for Swagger that enable custom annotations.
- Install the Swagger Library:
- Install the Swagger library or extension for your chosen framework. The library should provide support for generating Swagger documentation and parsing custom annotations.
- Define Custom Annotations:
- Create custom annotations or decorators in your code to annotate various elements of your API. These custom annotations can include descriptions, examples, validation rules, and other metadata that you want to include in your documentation.
- Use Custom Annotations:
- Annotate your API endpoints, request parameters, response objects, and other relevant elements with your custom annotations. This is typically done using decorators or annotations provided by your chosen framework.
- Generate Swagger Documentation:
- Use the Swagger library to generate Swagger documentation from your code, including the custom annotations. The library should be able to scan your codebase, identify the custom annotations, and incorporate them into the generated Swagger documentation.
- Serve Swagger Documentation:
- Configure your web application to serve the Swagger documentation, including the custom annotations, at a specific URL (e.g., “/swagger” or “/api-docs”). Users can access this documentation to learn about your API and its custom features.
Here’s an example of enhancing Swagger documentation with custom annotations in a Python Flask application using the flask-restx
extension (formerly known as flask-restplus
):
from flask import Flask
from flask_restx import Api, Resource, fields
app = Flask(__name__)
api = Api(app, version='1.0', title='Custom Annotations Example', description='Custom Annotations in Swagger')
# Custom annotation for a parameter
@api.param('custom_param', 'Custom parameter description', required=True)
# Custom annotation for a model (schema)
custom_model = api.model('CustomModel', {
'field1': fields.String(description='Field 1 description'),
'field2': fields.Integer(description='Field 2 description')
})
@api.route('/example')
class ExampleResource(Resource):
@api.doc('example_endpoint')
@api.response(200, 'Success', custom_model) # Custom response annotation
def get(self):
"""This is an example endpoint with custom annotations."""
return {'field1': 'value1', 'field2': 42}
if __name__ == '__main__':
app.run(debug=True)
In this example, custom annotations are used to add descriptions to a parameter, a response model (schema), and an API endpoint. The @api.param
, @api.model
, @api.doc
, and @api.response
decorators provided by flask-restx
are used to annotate the API elements.
When you generate Swagger documentation using this code, the custom annotations will be included, providing additional context and information for API consumers.
The exact syntax and usage of custom annotations may vary depending on the programming language, framework, and Swagger library you are using. Be sure to consult the documentation of your chosen tools for detailed instructions on implementing custom annotations.