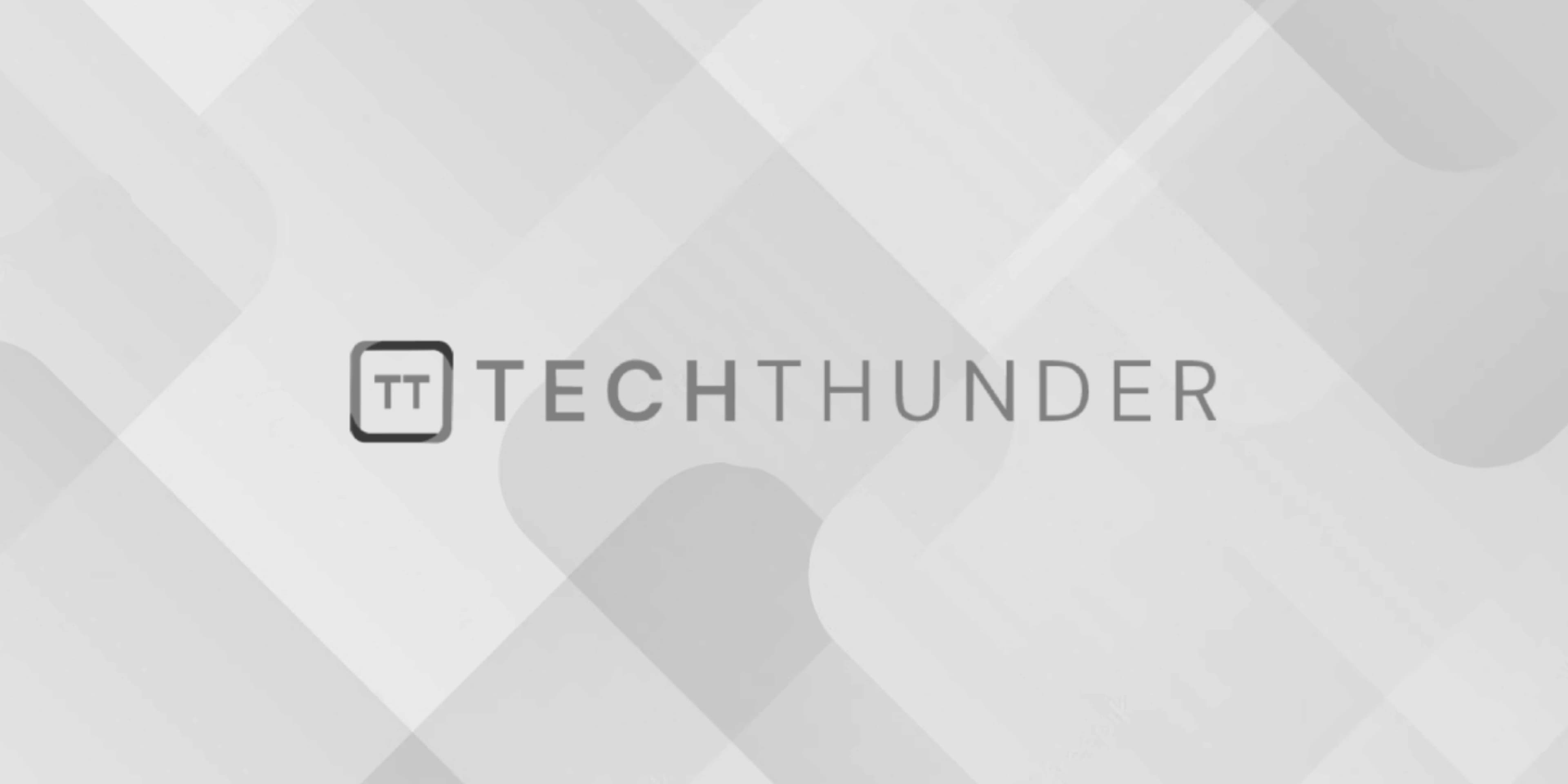
Spring Boot After Throwing Advice
The Spring Boot, “after-throwing” advice is a type of AOP (Aspect-Oriented Programming) advice that allows you to intercept and perform actions after an exception is thrown during the execution of a method. This advice is particularly useful for implementing error handling, logging, or cleanup operations in response to exceptions. You can implement after-throwing advice using Spring’s AOP capabilities. Here’s how to do it:
Step 1: Create an Aspect Class:
Start by creating an aspect class that contains methods annotated with @AfterThrowing
. These methods will execute after an exception is thrown in the specified pointcut. For example:
import org.aspectj.lang.annotation.AfterThrowing;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class ExceptionLoggingAspect {
@AfterThrowing(
pointcut = "execution(* com.example.myapp..*.*(..))",
throwing = "exception"
)
public void logException(Exception exception) {
// Log or perform actions in response to the exception
System.out.println("An exception occurred: " + exception.getMessage());
}
}
In this example:
@Aspect
indicates that the class is an aspect.@Component
marks it as a Spring component, allowing Spring to scan and recognize it.- The
logException
method is annotated with@AfterThrowing
, which defines the pointcut expression using thepointcut
attribute. In this case, it captures all methods in thecom.example.myapp
package and its subpackages. - The
throwing
attribute specifies the name of the parameter that will hold the thrown exception.
Step 2: Configure Spring to Enable AspectJ and Component Scanning:
In your Spring Boot application’s configuration, make sure you have enabled AspectJ support and component scanning:
@SpringBootApplication
@EnableAspectJAutoProxy
@ComponentScan(basePackages = "com.example.myapp")
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
@EnableAspectJAutoProxy
enables AspectJ proxying for Spring beans.@ComponentScan
specifies the base package(s) to scan for components, including the aspect class.
Step 3: Throw an Exception in Your Application:
To trigger the after-throwing advice, you need to throw an exception in one of your application’s methods. For example:
@Service
public class MyService {
public void performOperation() {
// Some code...
if (/* some condition */) {
throw new RuntimeException("An error occurred");
}
// More code...
}
}
In the performOperation
method, if a certain condition is met, it throws a RuntimeException
.
Step 4: Run the Application:
Run your Spring Boot application. When the performOperation
method throws an exception, the logException
method in your aspect class will be triggered, and you will see the exception message printed to the console.
This is a simple example of after-throwing advice in Spring Boot. You can customize the logException
method to perform various actions in response to exceptions, such as logging the error, sending notifications, or performing cleanup tasks.