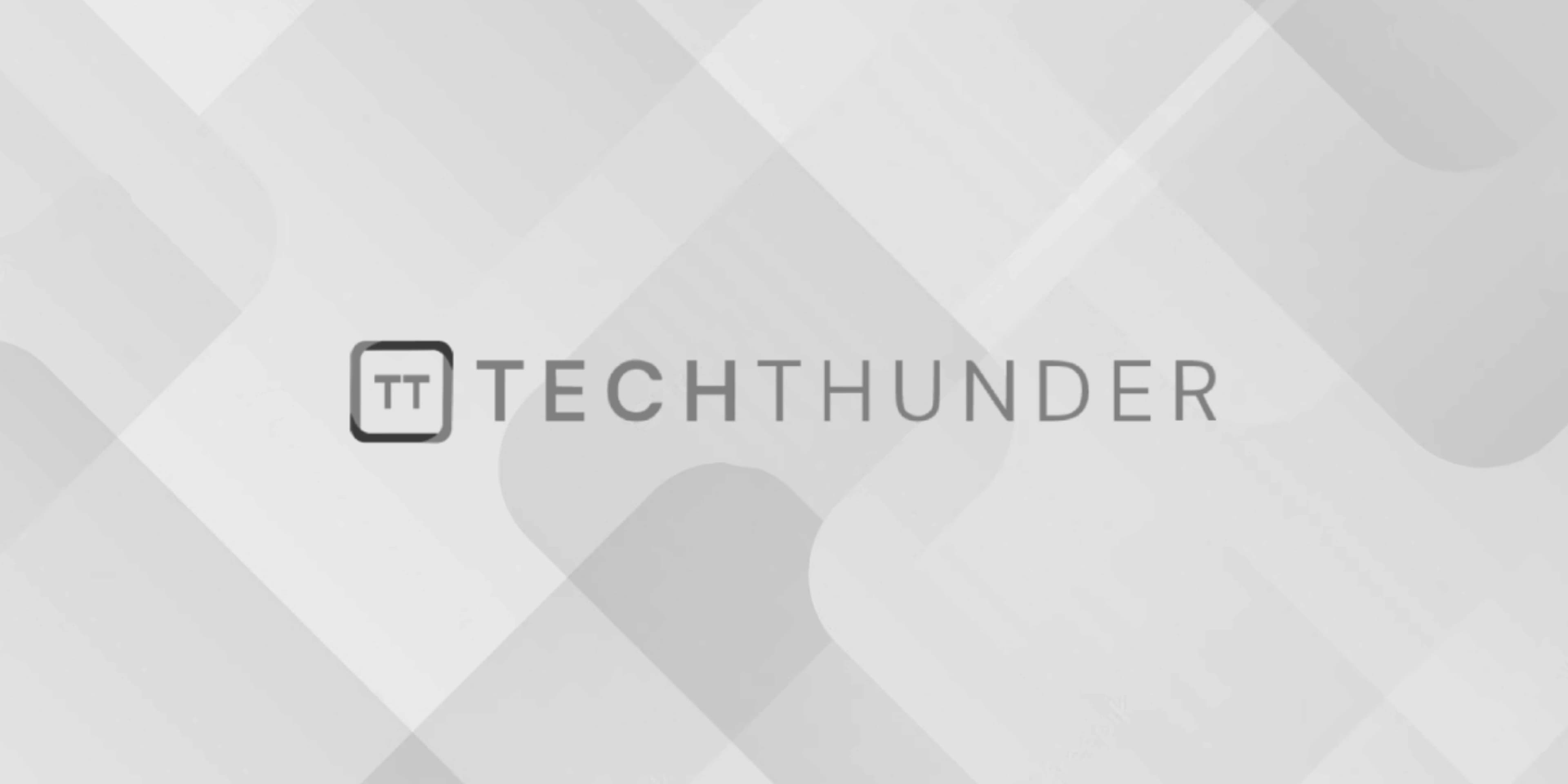
Spring Boot CRUD Operations
The Spring Boot application, performing CRUD (Create, Read, Update, Delete) operations is a common task when interacting with a database. You can achieve this by using Spring Data JPA, which simplifies database operations. Here’s a step-by-step guide on how to perform CRUD operations in a Spring Boot application:
Step 1: Create a Spring Boot Project:
You can create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Set Up the Database:
Configure the database connection settings in your application.properties
or application.yml
file, depending on your database choice (e.g., MySQL, PostgreSQL, H2, etc.). Include the URL, username, and password.
Step 3: Define Entity Classes:
Create entity classes that represent your data model. Annotate these classes with JPA annotations to define the mapping between Java objects and database tables.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Constructors, getters, setters
}
Step 4: Create a Repository Interface:
Create a repository interface for each entity. Spring Data JPA provides interfaces like JpaRepository
to handle basic CRUD operations. You can also define custom queries or methods in the repository interface.
public interface UserRepository extends JpaRepository<User, Long> {
// Custom queries or methods (if needed)
}
Step 5: Create a Service Layer:
Create a service class that encapsulates business logic and interacts with the repository. The service layer is where you implement custom business logic related to CRUD operations.
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<User> getAllUsers() {
return userRepository.findAll();
}
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
public User createUser(User user) {
return userRepository.save(user);
}
public User updateUser(User user) {
return userRepository.save(user);
}
public void deleteUser(Long id) {
userRepository.deleteById(id);
}
}
Step 6: Create RESTful Endpoints (Controller):
Create a controller class to define the RESTful endpoints for your CRUD operations. Use annotations like @RestController
, @RequestMapping
, and HTTP method annotations (@GetMapping
, @PostMapping
, @PutMapping
, @DeleteMapping
) to map the endpoints to your service methods.
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping
public List<User> getAllUsers() {
return userService.getAllUsers();
}
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
@PostMapping
public User createUser(@RequestBody User user) {
return userService.createUser(user);
}
@PutMapping("/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User user) {
user.setId(id);
return userService.updateUser(user);
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
userService.deleteUser(id);
}
}
Step 7: Run the Application:
Run your Spring Boot application, and it will automatically set up the database, create tables, and expose the RESTful endpoints defined in your controller.
Step 8: Test CRUD Operations:
Use tools like Postman, cURL, or your web browser to test the CRUD operations by making requests to the endpoints you defined in the UserController
.
This setup demonstrates a basic Spring Boot application with CRUD operations using Spring Data JPA. You can further enhance it by adding validation, error handling, security, and more complex queries as needed for your project.