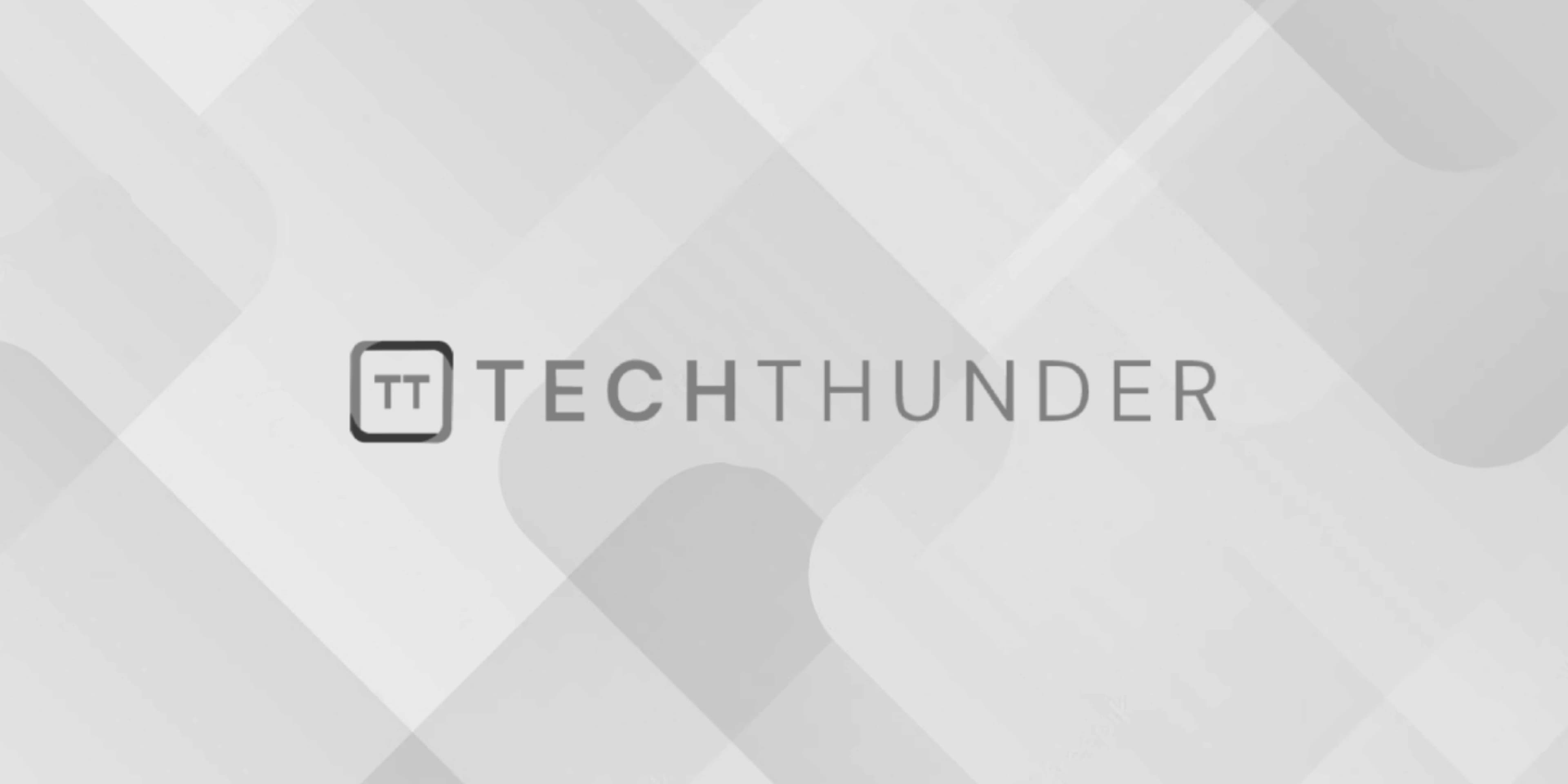
Implementing Basic Authentication with Spring Security
Implementing Basic Authentication with Spring Security in a Spring Boot application is a common way to secure your RESTful APIs. Basic Authentication requires clients to send credentials (username and password) with every request. Here’s how you can set it up:
- Add Dependencies:
In yourbuild.gradle
orpom.xml
, add the necessary Spring Security dependency. For Gradle:
implementation 'org.springframework.boot:spring-boot-starter-security'
For Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
- Configure Security:
Create a configuration class to customize Spring Security settings.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
The above configuration allows any request to access your API, but requires HTTP Basic Authentication for authentication.
- Define Users and Roles:
By default, Spring Security uses an auto-generated password for the useruser
. You can set your own users and passwords in theapplication.properties
orapplication.yml
:
spring.security.user.name=admin
spring.security.user.password=admin
spring.security.user.roles=USER,ADMIN
- Testing:
With Basic Authentication in place, you will need to include the credentials in the request headers for each request. For example, usingcurl
:
curl -u admin:admin http://localhost:8080/your-api-endpoint
Alternatively, you can use tools like Postman, Insomnia, or a web browser to test your authenticated API endpoints.
- Custom UserDetailsService (Optional):
If you want to provide your own user details for authentication, you can implement a customUserDetailsService
and override theconfigure
method in yourSecurityConfig
class.
import org.springframework.security.core.userdetails.UserDetailsService;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService);
}
}
Remember to secure your sensitive endpoints and resources with appropriate roles and permissions by customizing the authorizeRequests()
section in your security configuration.
This is a basic example of implementing Basic Authentication with Spring Security in a Spring Boot application. Depending on your application’s requirements, you may need to implement more advanced security features such as token-based authentication or OAuth2.