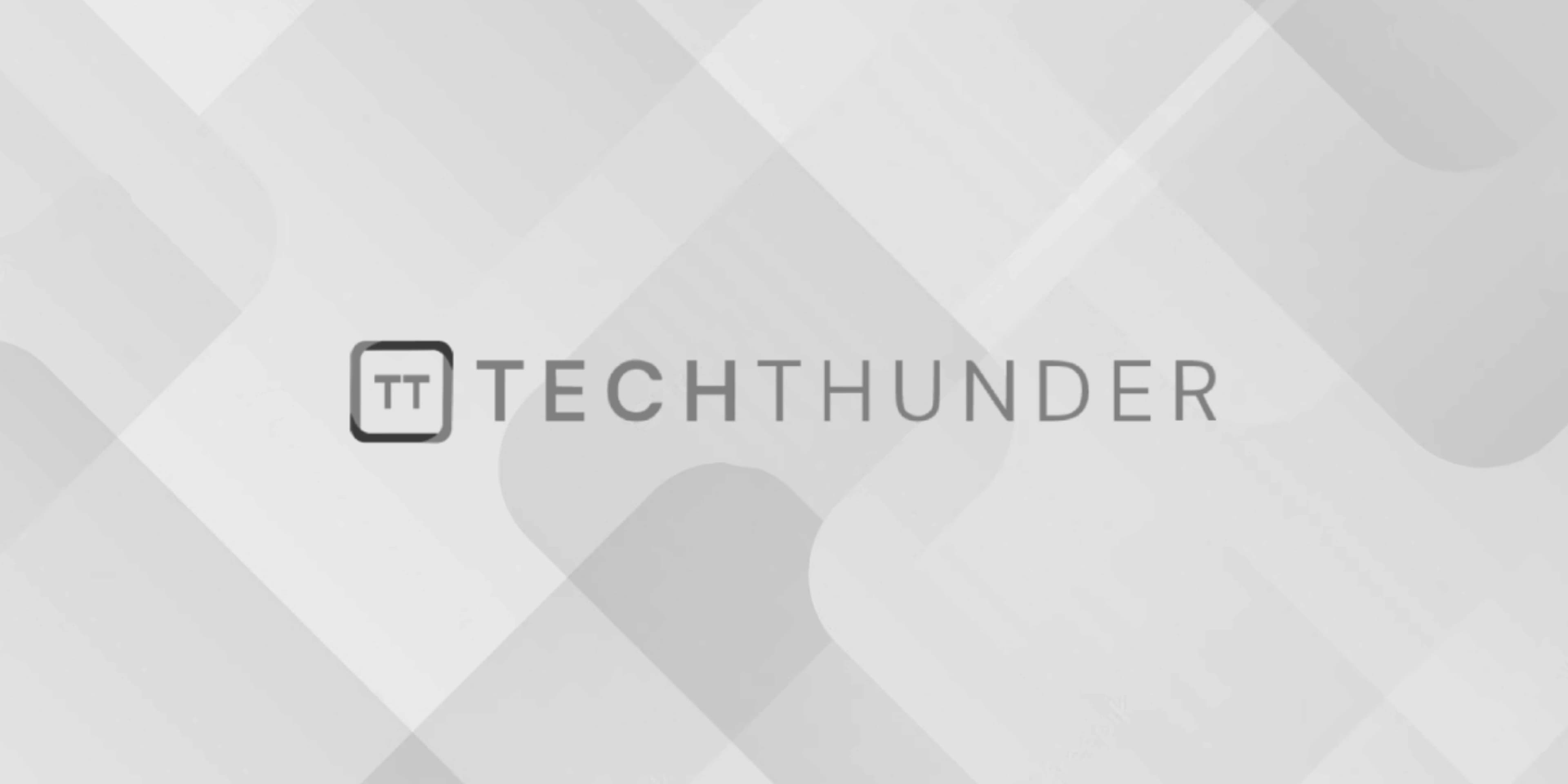
275 views
RESTful Web Services Best Practice
Creating RESTful web services in Spring Boot involves following certain best practices to ensure a well-organized, maintainable, and efficient API. Here are some best practices for designing RESTful web services using Spring Boot:
- Use Proper HTTP Methods:
- Map CRUD operations to appropriate HTTP methods: GET (retrieve), POST (create), PUT (update), DELETE (delete).
- Follow the semantics of each HTTP method for their intended purposes.
- Resource Naming:
- Use nouns in the URL path to represent resources (e.g.,
/users
,/orders
). - Avoid verbs in the URL path.
- Use Plural Nouns:
- Use plural nouns to represent collections of resources (e.g.,
/users
instead of/user
).
- Versioning:
- Consider versioning your APIs in the URL (e.g.,
/v1/users
). - Alternatively, use request headers or media types for versioning.
- Use HTTP Status Codes:
- Use appropriate HTTP status codes to indicate the result of an operation (e.g., 200 for success, 404 for not found, 201 for created).
- Return relevant error responses with proper status codes and error messages.
- Consistent Error Handling:
- Create a consistent error response format, possibly using a custom error model.
- Handle exceptions and errors gracefully, returning standardized error responses.
- Request and Response Body Format:
- Use JSON as the default format for request and response bodies.
- Provide a clear and well-documented request payload structure.
- Follow the principles of JSON:API or other standards if applicable.
- Use Proper HTTP Caching:
- Utilize HTTP caching headers (e.g.,
Cache-Control
,ETag
) for efficient caching of resources.
- Pagination and Filtering:
- Implement pagination for large collections using query parameters like
page
andsize
. - Allow filtering and sorting using query parameters.
- HATEOAS (Hypermedia as the Engine of Application State):
- Consider implementing HATEOAS to provide clients with links to related resources.
- Include relevant links in your response payloads to enable easier navigation.
- Input Validation:
- Validate user input and request parameters to ensure data integrity.
- Use validation annotations provided by Spring (e.g.,
@Valid
,@NotBlank
).
- Security:
- Implement proper authentication and authorization mechanisms (e.g., JWT, OAuth2) to secure your APIs.
- Apply security annotations to restrict access to specific endpoints.
- Internationalization and Localization:
- Support internationalization by providing messages in different languages based on user preferences.
- Testing:
- Write unit tests, integration tests, and end-to-end tests to ensure the correctness of your API.
- Utilize tools like JUnit and Mockito for testing.
- Documentation:
- Provide clear and comprehensive API documentation using tools like Swagger or Springfox.
- Include information about endpoints, request and response formats, and example requests.
- Code Organization:
- Organize your codebase using a modular structure.
- Use appropriate packages for controllers, services, repositories, models, etc.
By following these best practices, you can create well-designed and maintainable RESTful web services using Spring Boot, ensuring consistency, usability, and robustness in your APIs.