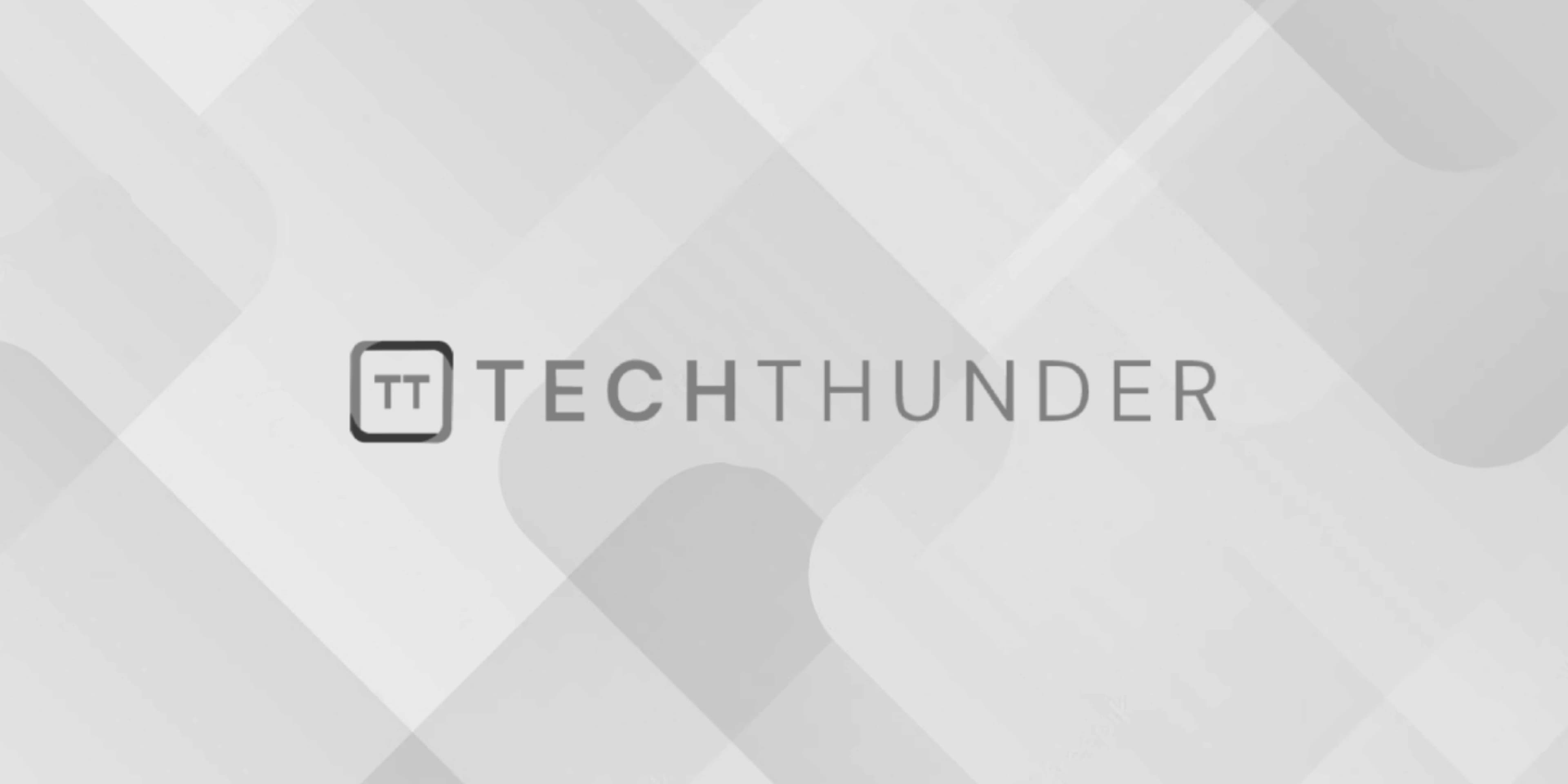
Spring Boot Auto-configuration
Spring Boot auto-configuration is a powerful feature that simplifies the configuration of your Spring Boot applications. It allows Spring Boot to automatically configure various beans and components based on the dependencies in your classpath, reducing the need for manual configuration. Auto-configuration is a key aspect of Spring Boot’s convention-over-configuration philosophy.
Here’s how Spring Boot auto-configuration works and how you can take advantage of it in your projects:
How Spring Boot Auto-Configuration Works:
- Classpath Scanning: Spring Boot scans the classpath for specific libraries, dependencies, and configuration classes to determine what features are available for auto-configuration.
- Condition Checks: For each auto-configuration class, Spring Boot performs condition checks to determine if the conditions for that auto-configuration are met. Conditions can be based on the presence or absence of specific classes, properties, or other conditions.
- Bean Creation: If the conditions for a particular auto-configuration are satisfied, Spring Boot creates and configures the necessary beans, services, and components. These beans are added to the Spring application context.
- Property Overrides: Spring Boot allows you to override auto-configuration properties in your application.properties or application.yml files, giving you fine-grained control over the auto-configuration.
How to Use Spring Boot Auto-Configuration:
- Add Dependencies: When you add dependencies to your Spring Boot project, Spring Boot’s auto-configuration mechanism will automatically configure relevant components. For example, adding the
spring-boot-starter-data-jpa
dependency will configure a DataSource and EntityManagerFactory for working with JPA. - Customize Configuration: If you need to customize the auto-configured beans, you can do so by defining your own configuration classes. Create a configuration class annotated with
@Configuration
and provide your own bean definitions or property overrides. Spring Boot will use your configuration in preference to the auto-configured defaults.
@Configuration
public class MyConfiguration {
@Bean
public MyBean customBean() {
return new MyBean();
}
}
- Property Overrides: You can override auto-configuration properties by specifying them in your application.properties or application.yml files. These property files allow you to customize various aspects of your Spring Boot application.
# Example: Override the default server port
server.port=8080
Benefits of Spring Boot Auto-Configuration:
- Reduced Configuration Boilerplate: Auto-configuration reduces the need for extensive configuration files, making your codebase cleaner and more focused on business logic.
- Convention Over Configuration: Spring Boot follows sensible defaults and conventions, allowing you to get started quickly with minimal configuration.
- Flexibility: You can easily customize and override auto-configured beans and properties to adapt to your specific requirements.
- Compatibility: Spring Boot’s auto-configuration is designed to be compatible with various libraries and frameworks, making it easier to integrate third-party components.
Spring Boot’s auto-configuration simplifies the development of Spring applications by providing a sensible and opinionated set of defaults. It empowers developers to focus on building application features rather than spending time on tedious configuration.