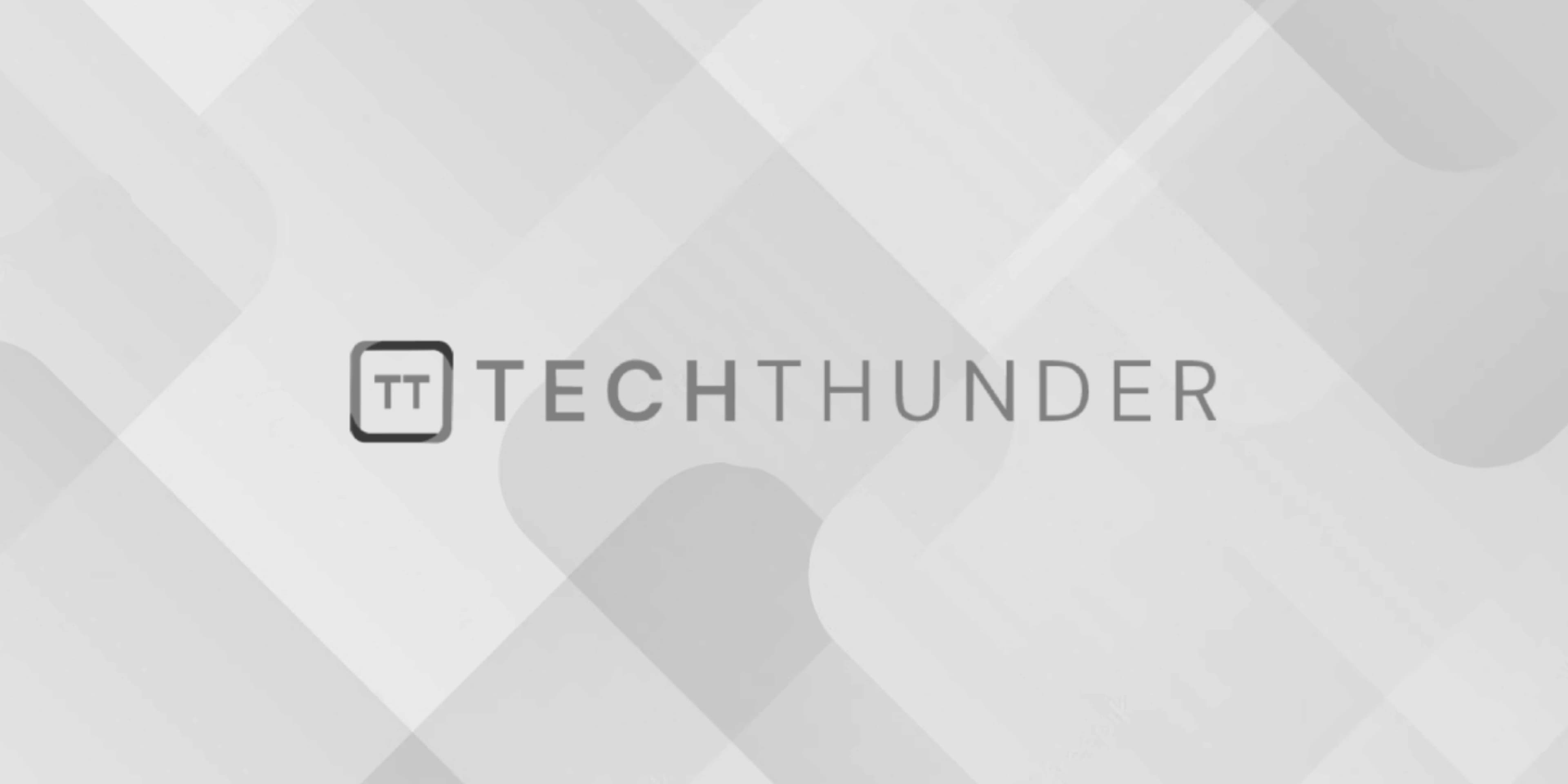
Versioning RESTful Web Services-Basic Approach With URIs
Versioning RESTful web services is essential to manage changes and updates to your API while ensuring backward compatibility with existing clients. One common approach to versioning is using URIs (Uniform Resource Identifiers) to specify the API version in the endpoint path. Here’s a basic approach to versioning RESTful web services using URIs:
1. Choose a Versioning Strategy:
- Decide on a versioning strategy that suits your application’s needs. Common versioning strategies include using numeric versions (e.g., v1, v2) or date-based versions (e.g., 2023-10-01).
2. Include the Version in the URI:
- Modify your API endpoints to include the version in the URI path. Typically, you would prepend a version identifier to the existing endpoint paths. For example:
/api/v1/resource
for version 1/api/v2/resource
for version 2
3. Maintain Backward Compatibility:
- When introducing a new version, strive to maintain backward compatibility with the previous version as much as possible. This means not removing or changing existing endpoints that clients depend on.
4. Document the Changes:
- Clearly document the changes and improvements introduced in each API version. Provide release notes or detailed documentation so that clients can understand what has changed and how to adapt their requests.
5. Update Clients Gradually:
- Inform clients about the new version and encourage them to update gradually. You may want to provide a deprecation period for the old version, during which both versions are supported.
6. Handle Versioning in Your Code:
- In your code, handle version-specific logic based on the version identifier extracted from the URI path. You can use conditional statements or routing mechanisms to direct requests to the appropriate version-specific code.
Example in Spring Boot:
Here’s an example of versioning a RESTful web service using Spring Boot:
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/v1/resource")
public ResponseEntity<String> getResourceV1() {
// Version 1 implementation
return ResponseEntity.ok("This is version 1 of the resource.");
}
@GetMapping("/v2/resource")
public ResponseEntity<String> getResourceV2() {
// Version 2 implementation
return ResponseEntity.ok("This is version 2 of the resource.");
}
}
In this example, there are two versions of the /api/resource
endpoint, each implemented in a separate method. Clients can access the desired version by including the version identifier in the URI path.
Using URI-based versioning is a straightforward approach and provides clear version differentiation. However, it can lead to longer and potentially less aesthetically pleasing URIs. Depending on your application’s needs, you might explore other versioning strategies such as using request headers or custom media types (e.g., Accept headers). The choice of versioning strategy should align with your API’s goals and the needs of your clients.