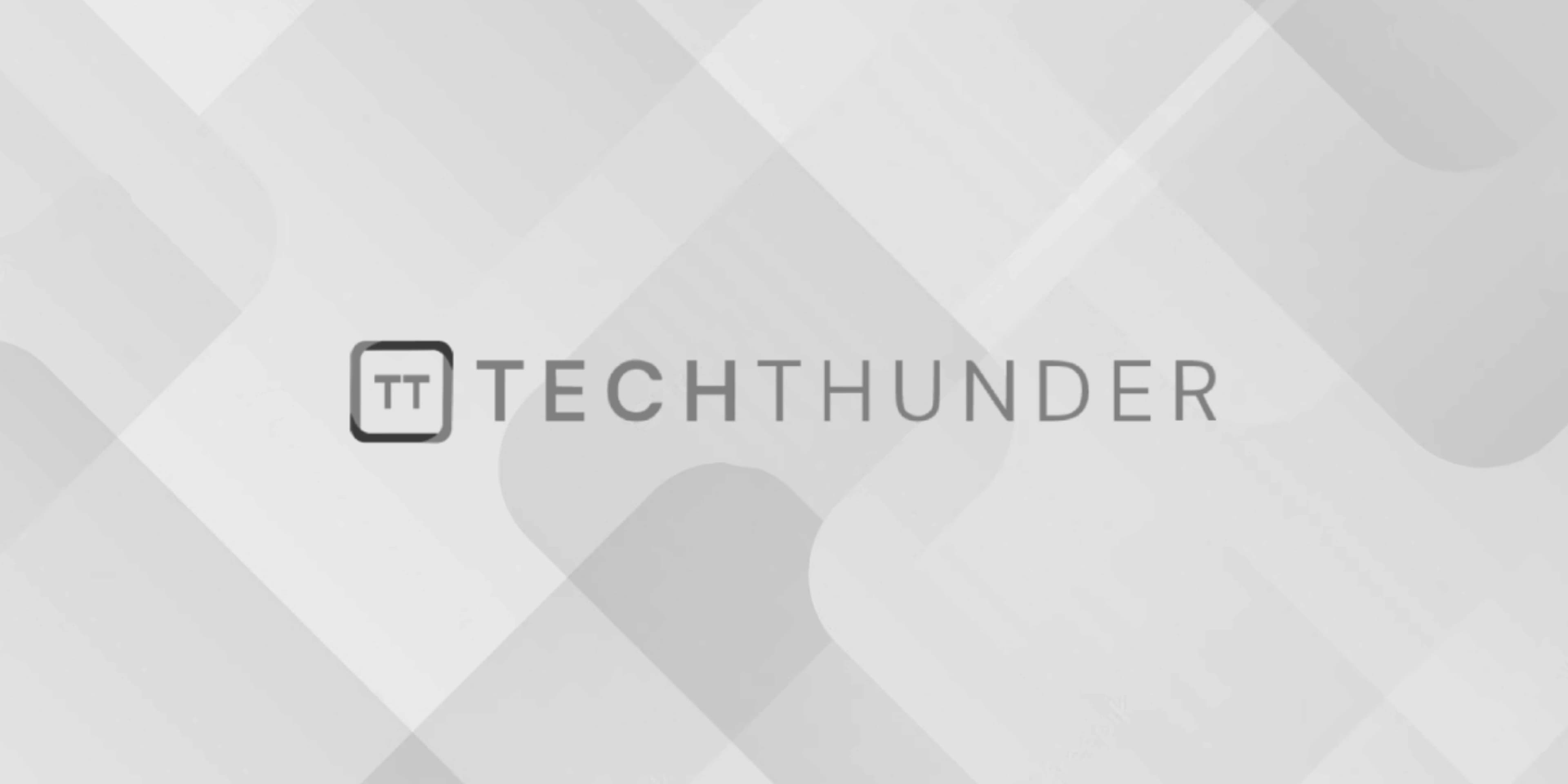
Spring Boot JPA
Spring Boot with JPA (Java Persistence API) is a powerful combination for building Java applications that interact with relational databases. JPA is a Java EE specification for object-relational mapping, and Spring Boot simplifies the development of Spring applications by providing auto-configuration and a variety of tools. Here’s how to use Spring Boot with JPA to create a database-driven application:
Step 1: Create a Spring Boot Project:
You can create a Spring Boot project using Spring Initializr, which is a web-based tool for generating Spring Boot projects. Visit the Spring Initializr website and configure your project by selecting the required dependencies, including “Spring Web” and “Spring Data JPA.”
Step 2: Define Entity Classes:
In JPA, entity classes represent database tables. Create entity classes for the tables you want to interact with in your database. Annotate these classes with JPA annotations to specify the mapping between the Java objects and the database tables.
Here’s an example of a simple entity class:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Constructors, getters, setters
}
Step 3: Create a Repository Interface:
Create a repository interface for each entity class. Spring Data JPA provides a convenient way to perform CRUD (Create, Read, Update, Delete) operations on the database.
public interface UserRepository extends JpaRepository<User, Long> {
// Define custom queries or methods here if needed
}
Step 4: Service Layer (Optional):
You can create a service layer to encapsulate business logic and interact with the repositories. The service layer typically contains methods that provide high-level operations on the entities.
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<User> getAllUsers() {
return userRepository.findAll();
}
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
public User createUser(User user) {
return userRepository.save(user);
}
public void deleteUser(Long id) {
userRepository.deleteById(id);
}
}
Step 5: Controller Layer:
Create controller classes to define the RESTful endpoints that interact with your service layer. Use annotations like @RestController
and @RequestMapping
to define the endpoints.
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping
public List<User> getAllUsers() {
return userService.getAllUsers();
}
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
@PostMapping
public User createUser(@RequestBody User user) {
return userService.createUser(user);
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
userService.deleteUser(id);
}
}
Step 6: Configure Database Connection:
In your application.properties
or application.yml
file, configure the database connection settings, including the database URL, username, and password.
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.jpa.hibernate.ddl-auto=update
Step 7: Run the Application:
You can now run your Spring Boot application. Spring Boot will automatically configure the data source, create tables (if specified in the configuration), and expose the RESTful endpoints defined in your controllers.
This is a basic setup for using Spring Boot with JPA. You can extend it by adding validation, security, error handling, and more advanced database operations as needed for your application.