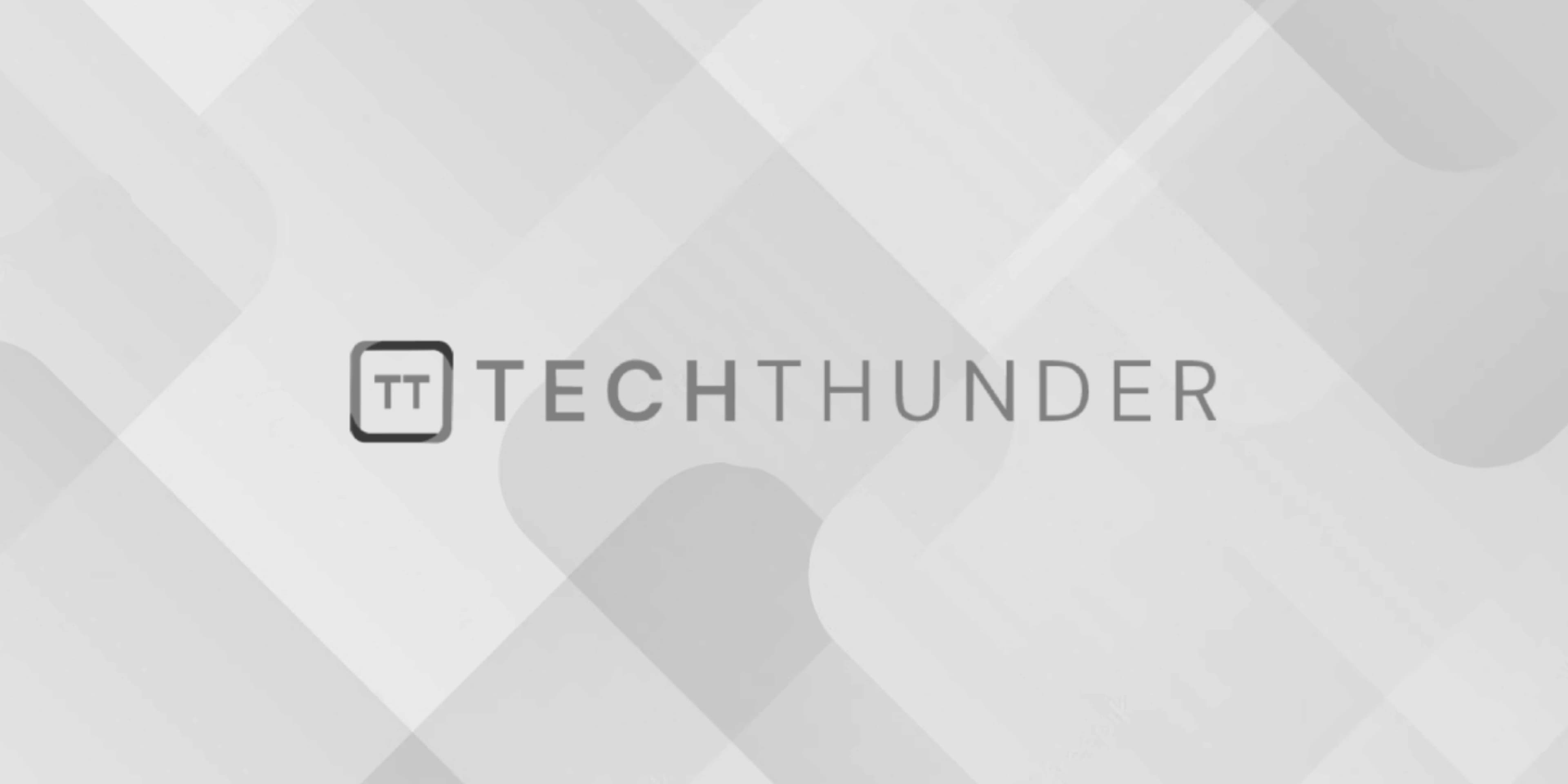
Implementing Generic Exception Handling for all Resources
To implement generic exception handling for all resources in a Spring Boot application, you can create a global exception handler that captures and handles exceptions thrown during the request handling process. This approach ensures that all unhandled exceptions are processed in a consistent manner, and error responses are returned to the client. Here are the steps to implement generic exception handling:
Step 1: Create a Custom Exception Class (Optional):
You can create a custom exception class if you have specific exceptions that you want to handle differently. This step is optional if you only need to capture and handle generic exceptions. For example, you can create a custom exception like CustomException
:
public class CustomException extends RuntimeException {
public CustomException(String message) {
super(message);
}
}
Step 2: Create a Global Exception Handler:
Create a global exception handler class annotated with @ControllerAdvice
. This class will handle exceptions globally for all resources in your application. Inside the handler class, define methods to handle specific exception types and return appropriate error responses:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleGenericException(Exception ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.INTERNAL_SERVER_ERROR.value(), ex.getMessage(), System.currentTimeMillis());
return new ResponseEntity<>(errorResponse, HttpStatus.INTERNAL_SERVER_ERROR);
}
@ExceptionHandler(CustomException.class)
public ResponseEntity<ErrorResponse> handleCustomException(CustomException ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.BAD_REQUEST.value(), ex.getMessage(), System.currentTimeMillis());
return new ResponseEntity<>(errorResponse, HttpStatus.BAD_REQUEST);
}
}
In the example above:
- We use the
@ExceptionHandler
annotation to specify which exception types each method should handle. In this case, we have two methods: one to handle generic exceptions (Exception
) and another to handle custom exceptions (CustomException
). - Inside each handler method, we create an
ErrorResponse
object containing the HTTP status code, error message, and timestamp. - We return a
ResponseEntity
with the error response and an appropriate HTTP status code.
Step 3: Create an ErrorResponse Class:
Define an ErrorResponse
class to structure the error response in a consistent format, as shown in the previous response:
public class ErrorResponse {
private int status;
private String message;
private long timestamp;
// Constructors, getters, and setters
}
Step 4: Throwing Exceptions:
In your controller or service layer, when you encounter exceptions, you can throw them, and the global exception handler will capture and process them. For example:
@GetMapping("/user/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
if (id == null) {
throw new CustomException("User ID cannot be null");
}
User user = userService.getUserById(id);
return new ResponseEntity<>(user, HttpStatus.OK);
}
In this example, if the id
is null
, a CustomException
is thrown, which will be caught by the global exception handler and return an error response.
Step 5: Test the Exception Handling:
Now, when a client sends a request that results in an exception, the global exception handler will capture and process the exception, returning an appropriate JSON error response.
By implementing a global exception handler, you ensure that all unhandled exceptions in your Spring Boot application are processed consistently, and clients receive informative error responses. You can customize the error handling logic further by adding more exception handler methods for specific exception types as needed.