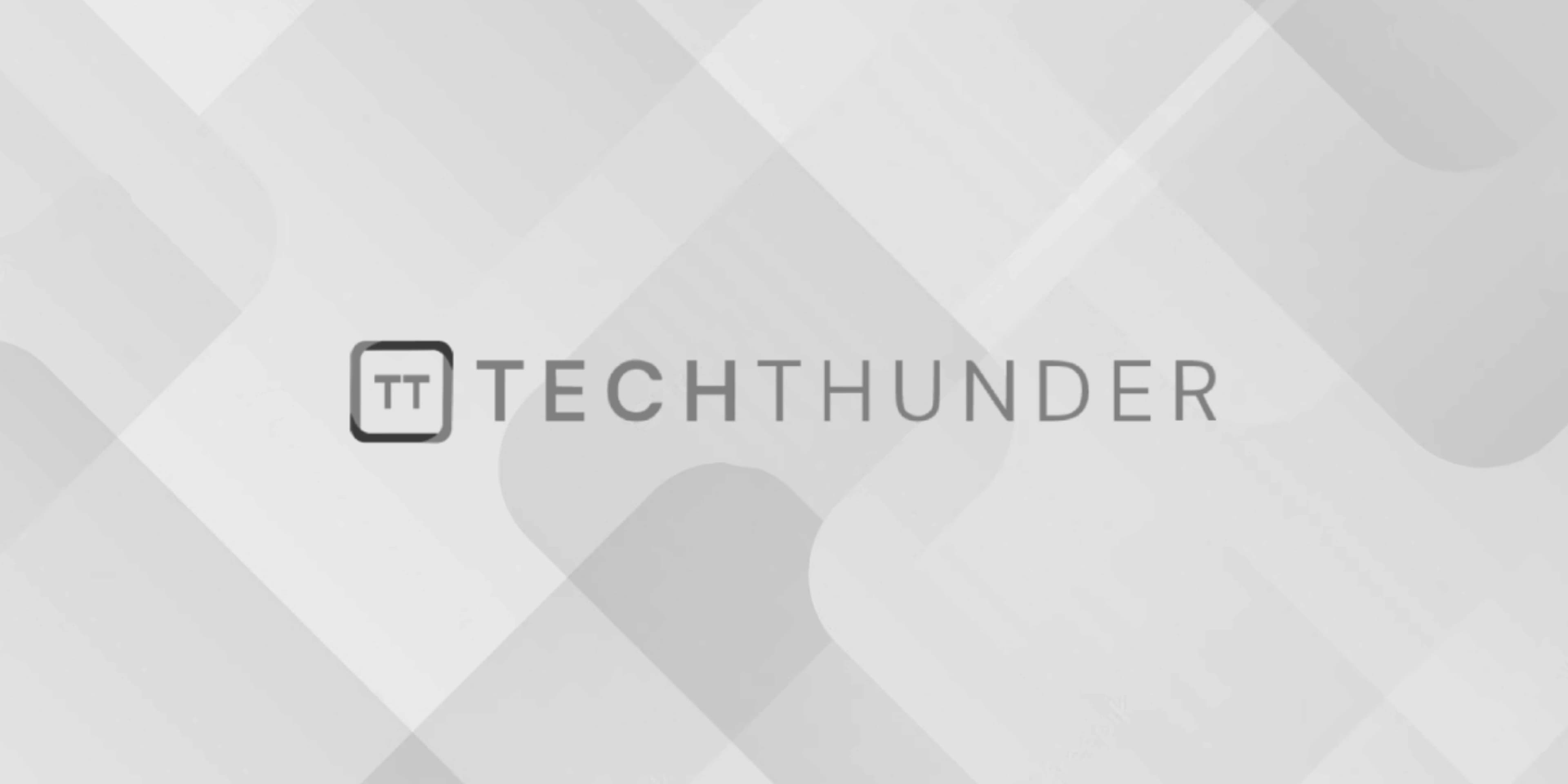
Spring Boot After Returning Advice
The Spring Boot AOP, an “after returning” advice is an aspect that executes after the target method has successfully completed its execution and returned a result. It is used to perform actions based on the method’s return value or to log information about the successful method execution. Here’s how to create an “after returning” advice using Spring Boot AOP:
Step 1: Create a Spring Boot Project
If you haven’t already, create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Add AOP Dependencies
In your project’s pom.xml
(Maven) or build.gradle
(Gradle), add the necessary dependencies for Spring AOP:
For Maven:
<dependencies>
<!-- ... other dependencies ... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
</dependencies>
For Gradle:
dependencies {
// ... other dependencies ...
implementation 'org.springframework.boot:spring-boot-starter-aop'
}
Step 3: Create an “After Returning” Advice
Now, create an “after returning” advice class that will be executed after the target method has successfully completed its execution and returned a result. Here’s an example of an “after returning” advice that logs the method’s return value:
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.AfterReturning;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class AfterReturningLoggingAdvice {
@AfterReturning(pointcut = "execution(* com.example.myapp.service.*.*(..))", returning = "result")
public void logAfterReturning(JoinPoint joinPoint, Object result) {
String methodName = joinPoint.getSignature().getName();
System.out.println("After invoking method: " + methodName);
System.out.println("Method returned: " + result);
}
}
In this example, we’ve created an aspect named AfterReturningLoggingAdvice
, and it defines an “after returning” advice method logAfterReturning
. The @AfterReturning
annotation specifies the pointcut expression that determines when this advice should be executed. In this case, it is executed after any method in the com.example.myapp.service
package has returned a result. The returning
attribute is used to capture the method’s return value.
Step 4: Use AOP Annotations
To apply the “after returning” advice to specific methods or classes, you can use AOP annotations such as @Before
, @After
, @Around
, and others. Here’s an example of how to use @AfterReturning
to apply the “after returning” advice to a specific method:
import org.springframework.stereotype.Service;
@Service
public class MyService {
public int calculateSquare(int number) {
// This is the target method
int result = number * number;
return result;
}
}
In this example, we’ve applied the “after returning” advice to the calculateSquare
method of the MyService
class.
Step 5: Run Your Spring Boot Application
Run your Spring Boot application as you normally would. When the calculateSquare
method is invoked, the “after returning” advice (logAfterReturning
) will execute after the method has successfully completed, and you will see the log messages, including the method’s return value.
That’s it! You’ve created and applied an “after returning” advice using Spring Boot AOP. You can use this approach to perform actions or logging based on the successful return value of specific methods in your application.