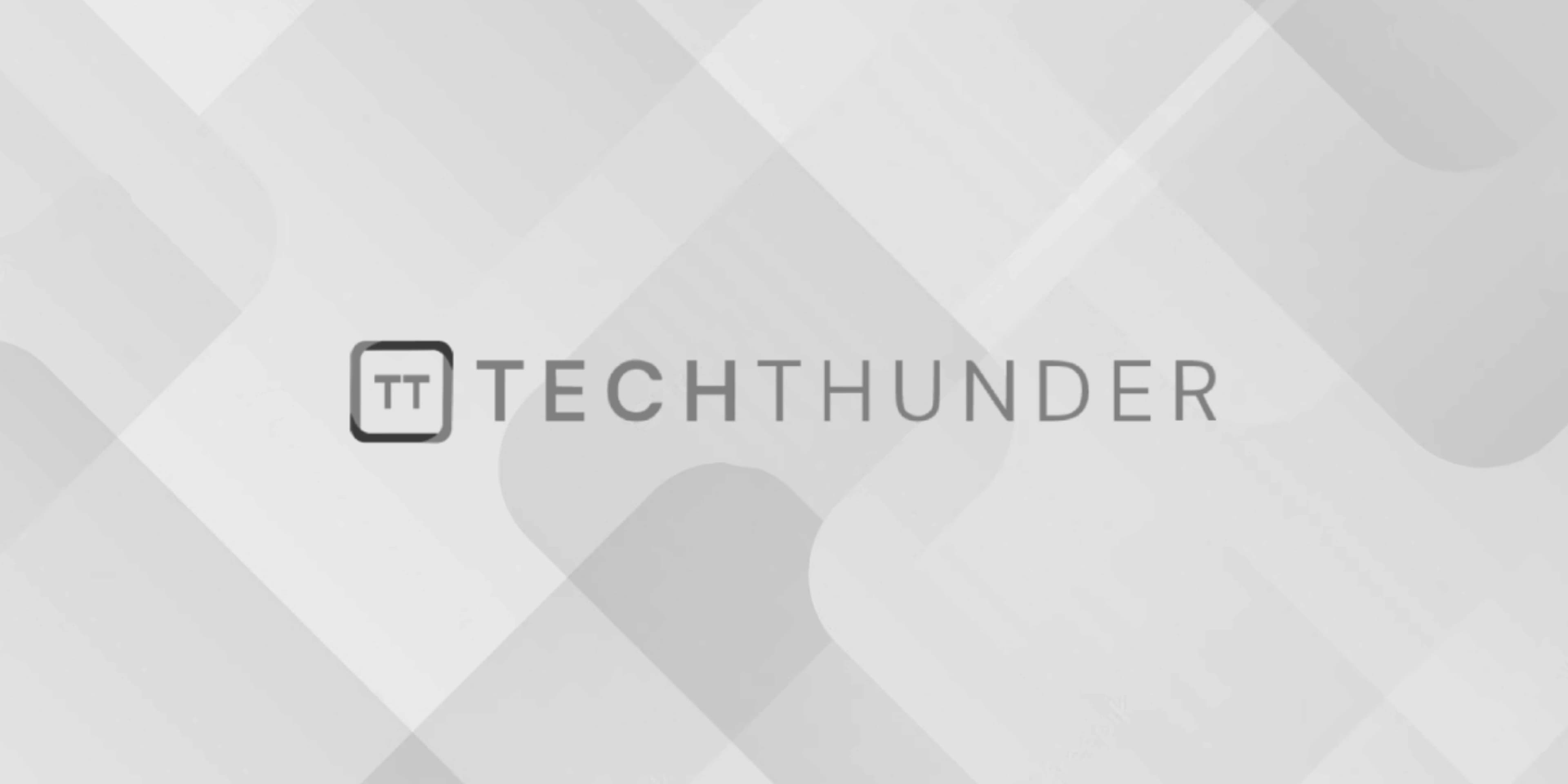
Spring Boot AOP Around Advice
The Spring Boot AOP, an “around” advice is an aspect that wraps around the target method’s execution. It has the ability to control whether the target method should be executed, modify the method’s input or output, or perform custom logic before and after method execution. Here’s how to create an “around” advice using Spring Boot AOP:
Step 1: Create a Spring Boot Project
If you haven’t already, create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Add AOP Dependencies
In your project’s pom.xml
(Maven) or build.gradle
(Gradle), add the necessary dependencies for Spring AOP:
For Maven:
<dependencies>
<!-- ... other dependencies ... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
</dependencies>
For Gradle:
dependencies {
// ... other dependencies ...
implementation 'org.springframework.boot:spring-boot-starter-aop'
}
Step 3: Create an “Around” Advice
Now, create an “around” advice class that will wrap around the target method’s execution. Here’s an example of an “around” advice that logs before and after method execution:
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class AroundLoggingAdvice {
@Around("execution(* com.example.myapp.service.*.*(..))")
public Object logAroundMethod(ProceedingJoinPoint joinPoint) throws Throwable {
String methodName = joinPoint.getSignature().getName();
// Log before method execution
System.out.println("Before invoking method: " + methodName);
// Proceed with the actual method execution
Object result = joinPoint.proceed();
// Log after method execution
System.out.println("After invoking method: " + methodName);
return result;
}
}
In this example, we’ve created an aspect named AroundLoggingAdvice
, and it defines an “around” advice method logAroundMethod
. The @Around
annotation specifies the pointcut expression that determines when this advice should be executed. In this case, it is executed around any method in the com.example.myapp.service
package.
Step 4: Use AOP Annotations
To apply the “around” advice to specific methods or classes, you can use AOP annotations such as @Before
, @After
, @Around
, and others. Here’s an example of how to use @Around
to apply the “around” advice to a specific method:
import org.springframework.stereotype.Service;
@Service
public class MyService {
public void doSomething() {
// This is the target method
System.out.println("Doing something...");
}
}
In this example, we’ve applied the “around” advice to the doSomething
method of the MyService
class.
Step 5: Run Your Spring Boot Application
Run your Spring Boot application as you normally would. When the doSomething
method is invoked, the “around” advice (logAroundMethod
) will execute before and after the method, and you will see the log messages.
You’ve created and applied an “around” advice using Spring Boot AOP. You can use this approach to wrap around the execution of specific methods in your application, perform custom logic, modify method input or output, and control whether the method should be executed.