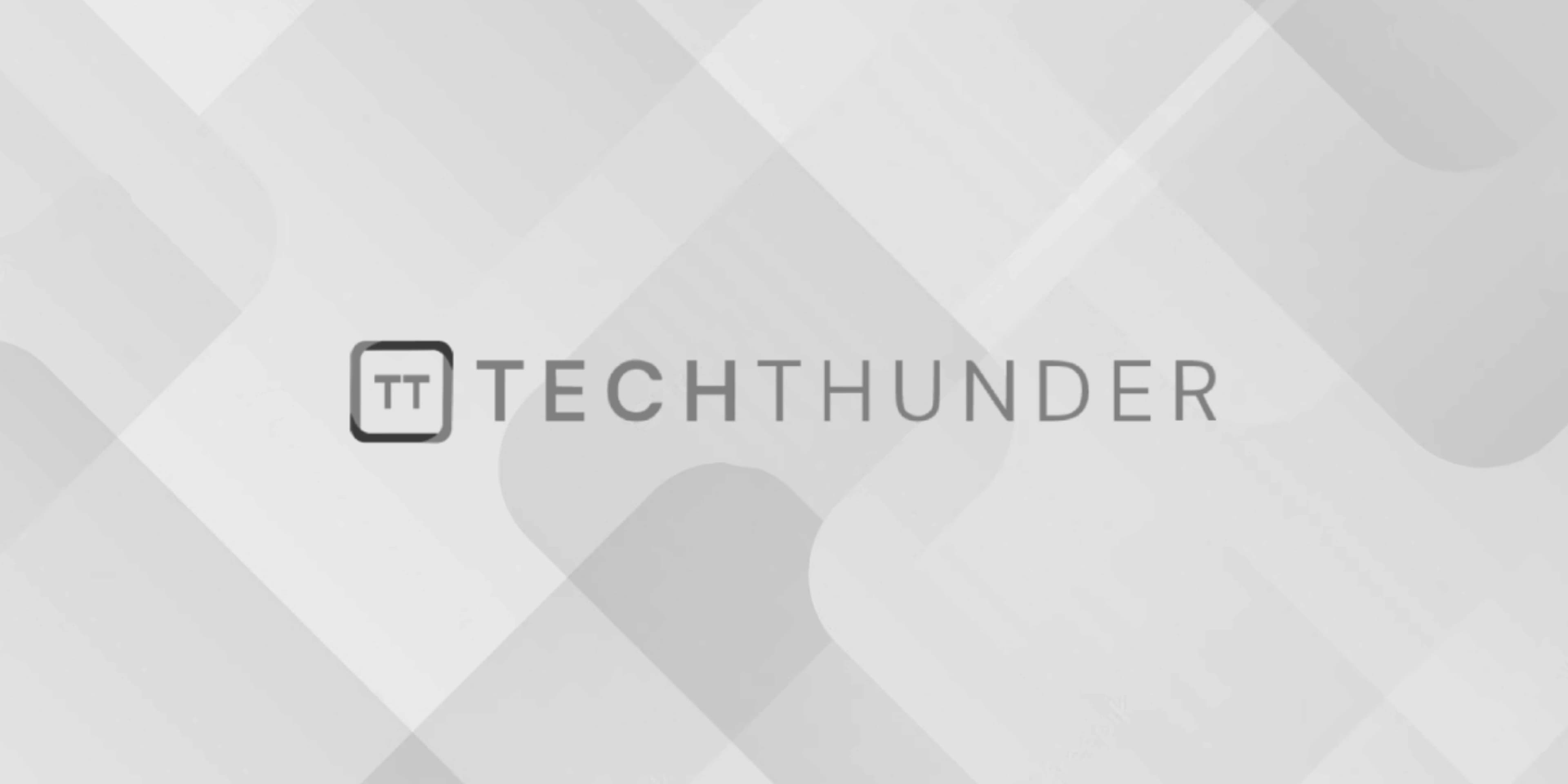
Monitoring APIs with Spring Boot Actuator
Spring Boot Actuator is a set of production-ready features and tools for monitoring and managing Spring Boot applications. It provides a wide range of functionality, including health checks, metrics, application info, environment properties, and more. Monitoring APIs with Spring Boot Actuator allows you to gain insights into your application’s runtime behavior and diagnose issues. Here are the key steps to monitor APIs using Spring Boot Actuator:
1. Add Spring Boot Actuator Dependency:
- Start by adding the Spring Boot Actuator dependency to your project’s
pom.xml
(for Maven) orbuild.gradle
(for Gradle). The dependency typically looks like this:
<!-- For Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
// For Gradle
implementation 'org.springframework.boot:spring-boot-starter-actuator'
2. Configure Actuator Endpoints:
- By default, Spring Boot Actuator provides several built-in endpoints, such as
/actuator/health
,/actuator/metrics
,/actuator/info
, etc. You can configure which endpoints are enabled or disabled in yourapplication.properties
orapplication.yml
file. For example, to enable all endpoints, you can use:
management.endpoints.web.exposure.include=*
3. Access Actuator Endpoints:
- Once configured, you can access the Actuator endpoints by making HTTP GET requests to the appropriate URLs. For example:
/actuator/health
: Provides information about the application’s health./actuator/metrics
: Offers various metrics related to your application./actuator/info
: Provides custom information about your application./actuator/env
: Displays environment properties./actuator/beans
: Lists all Spring beans in the application./actuator/mappings
: Shows a list of all URL mappings.
4. Securing Actuator Endpoints (Optional):
- By default, Actuator endpoints may not be secure, and you might want to restrict access to certain endpoints in a production environment. You can use Spring Security or other authentication mechanisms to secure these endpoints.
5. Customizing Actuator Endpoints (Optional):
- You can customize the behavior and content of Actuator endpoints by providing your own implementations or by configuring them using properties in your
application.properties
orapplication.yml
file. For example, you can add custom health indicators or customize the/actuator/info
endpoint.
6. Use Actuator Data for Monitoring:
- Once your Actuator endpoints are configured and secured, you can use the data provided by these endpoints for monitoring and management purposes. For example, you can set up monitoring tools or dashboards that regularly query the
/actuator/metrics
endpoint to collect and visualize application metrics.
7. Extend Actuator with Custom Endpoints (Optional):
- In addition to the built-in endpoints, you can create custom Actuator endpoints by extending the
AbstractEndpoint
class. This allows you to expose application-specific information or perform custom management tasks through Actuator.
Spring Boot Actuator is a powerful tool for monitoring and managing Spring Boot applications in a production environment. It provides a wealth of information and tools to help you ensure the health and performance of your APIs. Properly configuring and securing Actuator endpoints is essential to make the most of these features.