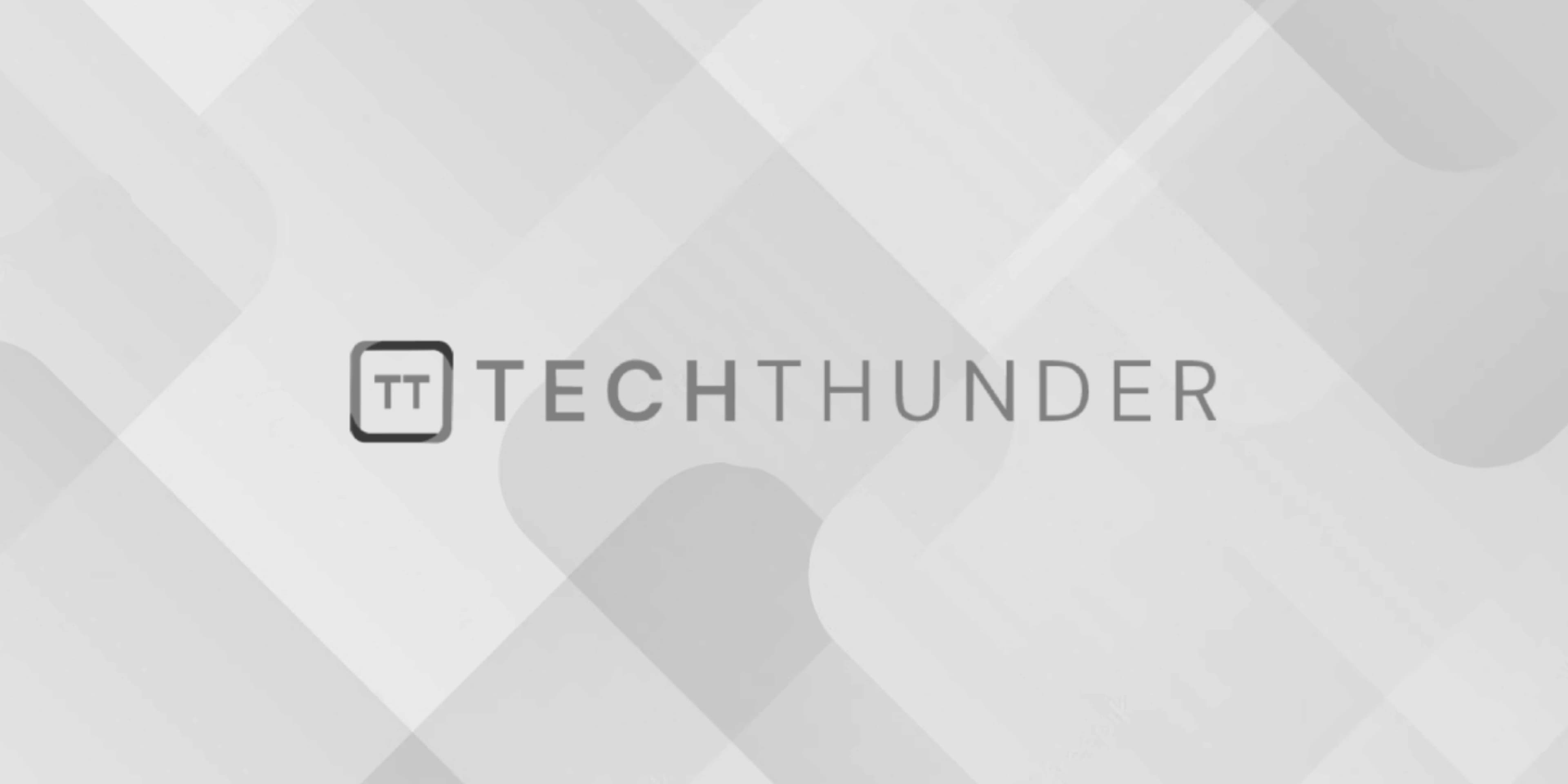
Spring Boot AOP
Spring Boot provides support for Aspect-Oriented Programming (AOP) through the Spring AOP framework. AOP allows you to modularize cross-cutting concerns in your application, such as logging, security, and transactions. In Spring Boot, you can use AOP to apply aspects to your application’s components, enhancing their behavior without modifying their code directly.
Here’s a step-by-step guide on how to use AOP in a Spring Boot application:
Step 1: Create a Spring Boot Project
If you haven’t already, create a Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Add AOP Dependencies
In your project’s pom.xml
(Maven) or build.gradle
(Gradle), add the necessary dependencies for Spring AOP:
For Maven:
<dependencies>
<!-- ... other dependencies ... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
</dependencies>
For Gradle:
dependencies {
// ... other dependencies ...
implementation 'org.springframework.boot:spring-boot-starter-aop'
}
Step 3: Create an Aspect
An aspect is a class that defines cross-cutting concerns and contains advice (methods) that are executed at specific points in your application’s execution. Here’s an example of an aspect that logs method execution:
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@After("execution(* com.example.myapp.service.*.*(..))")
public void logMethodExecution(JoinPoint joinPoint) {
String methodName = joinPoint.getSignature().getName();
System.out.println("Method executed: " + methodName);
}
}
In this example, we’ve created an aspect called LoggingAspect
that logs the execution of methods in the com.example.myapp.service
package.
Step 4: Configure AOP
By default, Spring Boot autoconfigures AOP for you. However, you can customize the AOP configuration if needed. For example, you can configure where to scan for aspects, set the order of aspect execution, etc. Configuration can be done in a @Configuration
class:
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@Configuration
@EnableAspectJAutoProxy
public class AopConfig {
// Additional AOP configuration if needed
}
Step 5: Use AOP Annotations
To apply AOP to specific methods or classes, you can use AOP annotations such as @Before
, @After
, @Around
, and others. Here’s an example of how to use @Before
and @After
annotations:
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Before("execution(* com.example.myapp.service.*.*(..))")
public void beforeMethod() {
// This advice runs before the target method
System.out.println("Before method execution");
}
@After("execution(* com.example.myapp.service.*.*(..))")
public void afterMethod() {
// This advice runs after the target method (regardless of success or failure)
System.out.println("After method execution");
}
}
In this example, we’ve applied @Before
and @After
advice to all methods in the MyService
class.
Step 6: Run Your Spring Boot Application
Run your Spring Boot application as you normally would. The AOP aspects you’ve defined will automatically intercept method calls and execute the advice methods accordingly.
That’s it! You’ve successfully set up and used AOP in a Spring Boot application. You can now apply AOP to various cross-cutting concerns in your application, such as logging, security, and performance monitoring, without cluttering your core business logic.