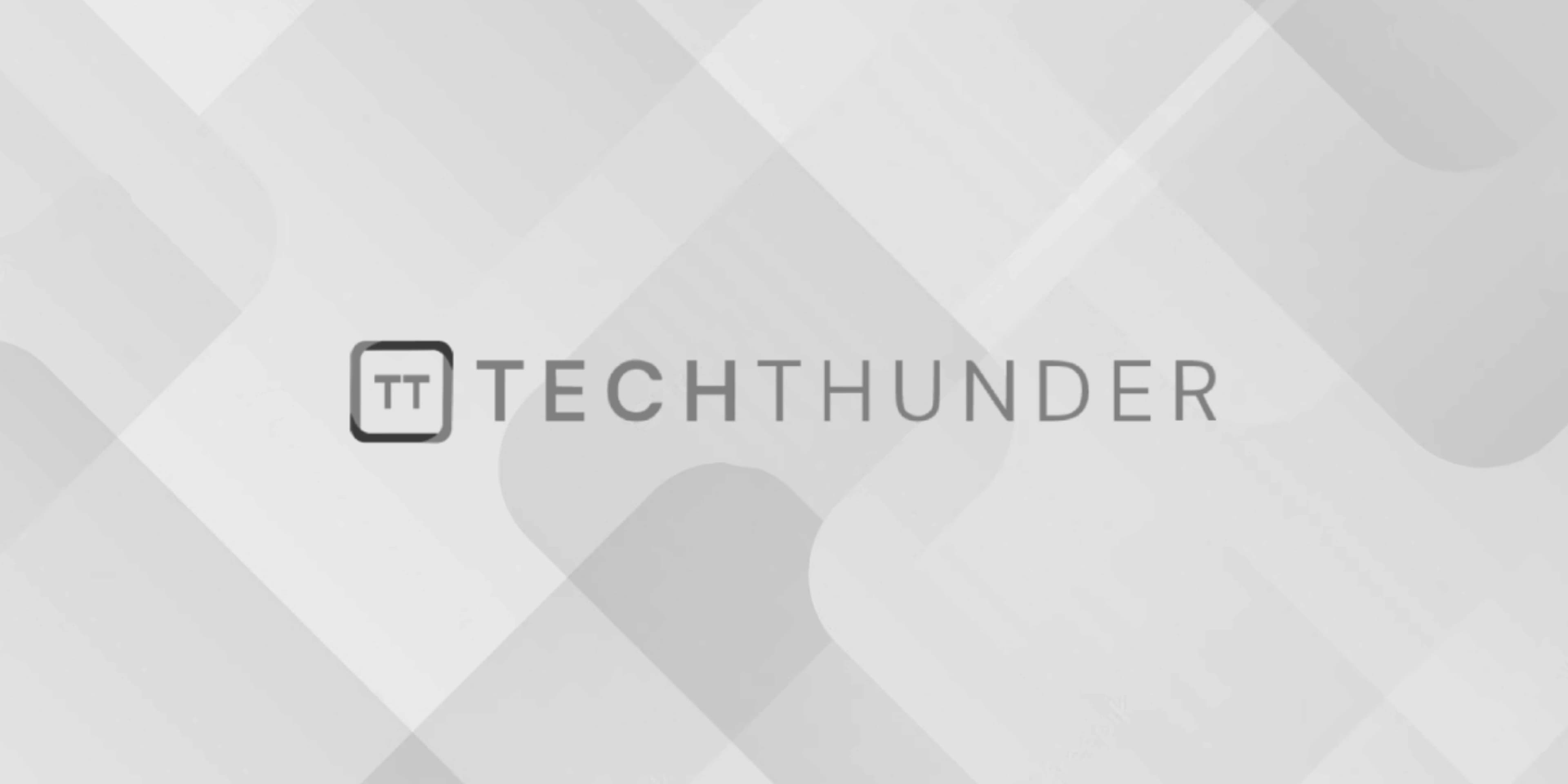
246 views
Spring Boot Annotations
Spring Boot relies heavily on annotations to simplify configuration and development. These annotations are used to define various aspects of your Spring Boot application, such as components, configurations, endpoints, and more. Here are some commonly used Spring Boot annotations and their purposes:
- @SpringBootApplication:
- This annotation marks the main class of a Spring Boot application.
- It combines several annotations into one, including
@Configuration
,@EnableAutoConfiguration
, and@ComponentScan
. - It’s typically placed on the class containing the
main()
method to bootstrap the application.
- @Controller:
- Indicates that a class is a Spring MVC controller.
- Controllers handle incoming HTTP requests and return responses.
- @RestController:
- This is a specialization of
@Controller
. - It indicates that a class is a controller that returns data instead of rendering views.
- Methods in a
@RestController
are typically annotated with@RequestMapping
or its variants to map HTTP endpoints.
- @RequestMapping:
- Maps HTTP requests to controller methods.
- Used to specify the URL path and HTTP method for a particular endpoint.
- Can be used at both class and method levels.
- @GetMapping, @PostMapping, @PutMapping, @DeleteMapping, @PatchMapping:
- These annotations are shortcuts for specifying the HTTP method in
@RequestMapping
. - They are often used for RESTful services to define specific HTTP methods for handling requests.
- @Autowired:
- Used for automatic dependency injection.
- When applied to fields, constructors, or methods, it tells Spring to inject the appropriate bean or component.
- @Component:
- Marks a class as a Spring component.
- Spring Boot will automatically scan for classes with this annotation and register them as beans.
- @Service:
- A specialization of
@Component
. - It is typically used to annotate service classes.
- @Repository:
- A specialization of
@Component
. - It is used to annotate classes that interact with a database or a data source.
- @Configuration:
- Indicates that a class defines Spring bean configurations.
- Methods within the class marked with
@Bean
will return Spring beans that can be managed by the Spring container.
- @EnableAutoConfiguration:
- Enables Spring Boot’s automatic configuration.
- This annotation allows Spring Boot to configure your application based on the classpath and project dependencies.
- @Value:
- Used to inject property values from property files or environment variables into fields, constructor parameters, or methods.
- @Profile:
- Allows you to activate or deactivate a specific bean or configuration class based on the active Spring profiles.
- @ConditionalOnProperty:
- Specifies that a bean should be created only if a certain property is present in the configuration.
- @ConfigurationProperties:
- Binds properties from property files to fields in a Java bean.
- @SpringBootTest:
- Used for integration testing.
- Starts the complete Spring application context for testing.
- @Test:
- A JUnit annotation used to mark a method as a test method.
- When combined with Spring Boot’s test framework, it allows for easy unit and integration testing.
These are just a few of the many annotations provided by Spring Boot. Using these annotations appropriately can significantly simplify the configuration and development of Spring Boot applications. You can also create custom annotations or leverage annotations from other Spring projects, such as Spring Data or Spring Security, to further enhance your Spring Boot application.