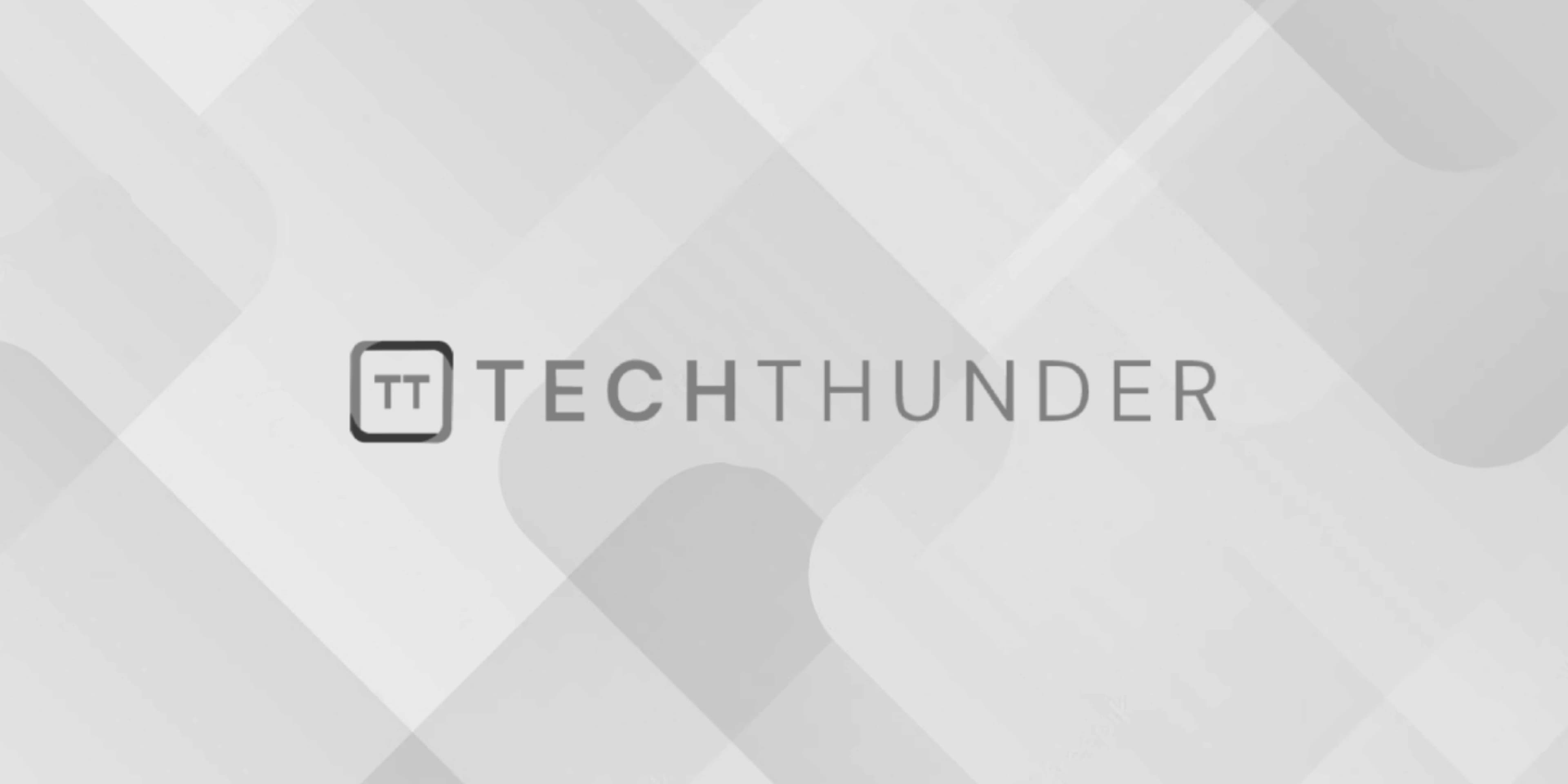
Spring Boot Example
The simple Spring Boot example that demonstrates the creation of a RESTful web service with a single endpoint that returns “Hello, World!” as a response. This example assumes you have Spring Boot and a development environment (e.g., Java and an Integrated Development Environment) set up.
Step 1: Create a Spring Boot Project
You can create a Spring Boot project using the Spring Initializr or your preferred IDE. Here’s how to do it using the Spring Initializr:
- Visit the Spring Initializr web page at https://start.spring.io/.
- Configure your project by selecting “Maven Project” or “Gradle Project,” choosing the language (Java or Kotlin), and setting the Spring Boot version.
- Define your project’s metadata (e.g., Group, Artifact, Package name).
- Add the “Spring Web” dependency from the “Dependencies” section.
- Click the “Generate” button to download the project as a ZIP file.
Step 2: Set Up Your Project in an IDE
If you’re using an IDE like IntelliJ IDEA or Eclipse, import the project by opening the downloaded ZIP file. If you’re using a text editor, extract the ZIP file to your desired directory.
Step 3: Write the Spring Boot Code
Create a new Java class, e.g., HelloController.java
, in your project’s source code directory (usually src/main/java/com/example/demo
) with the following code:
package com.example.demo;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
In this code:
@RestController
annotation marks the class as a Spring MVC controller, indicating that it will handle HTTP requests and return data as JSON.@GetMapping("/hello")
maps the/hello
URL path to thesayHello()
method.sayHello()
is a simple method that returns the “Hello, World!” string.
Step 4: Run the Application
You can run your Spring Boot application using your IDE or from the command line. To run it from the command line, open a terminal, navigate to your project directory, and use the following command (Maven example):
mvn spring-boot:run
Step 5: Access the Hello World Endpoint
Open a web browser or use a tool like curl
to access the “Hello World” endpoint:
- Open a web browser and go to http://localhost:8080/hello (assuming your application is running on the default port).
- You should see the “Hello, World!” message displayed in your browser.
That’s it! You’ve created a simple Spring Boot application that serves a “Hello, World!” message via a RESTful web service. This is a basic example, and Spring Boot can be used to build much more complex and feature-rich applications with ease.