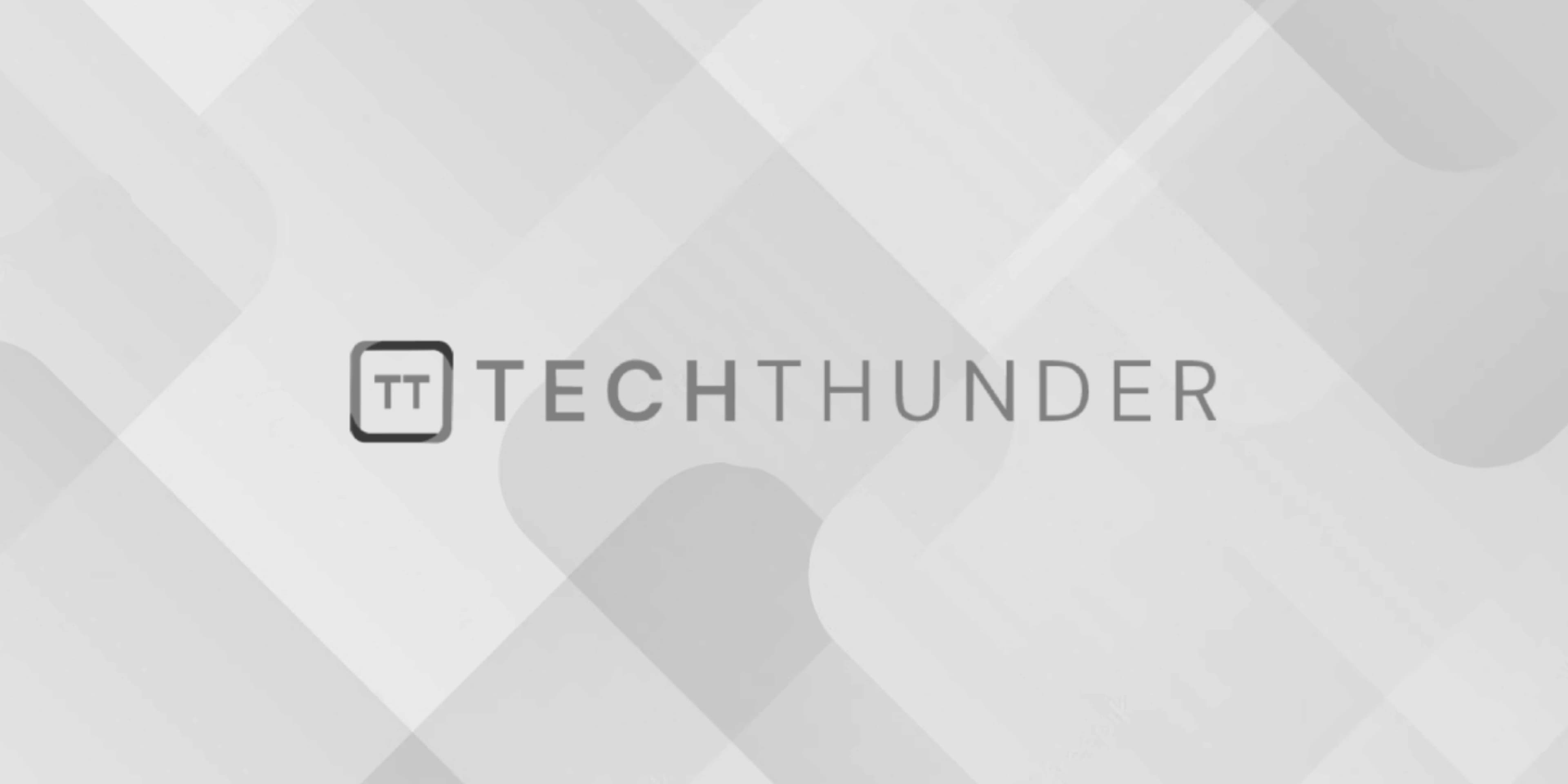
127 views
Spring Boot Tutorial
Creating a comprehensive Spring Boot tutorial here is beyond the scope of this text-based format, but I can provide you with a high-level outline of the typical steps involved in building a Spring Boot application and suggest some resources where you can find detailed tutorials:
Steps to Create a Spring Boot Application:
- Setup Your Development Environment:
- Install Java JDK 8 or later.
- Install a build tool like Maven or Gradle.
- Optionally, install an Integrated Development Environment (IDE) such as IntelliJ IDEA, Eclipse, or Visual Studio Code.
- Create a New Spring Boot Project:
- Use the Spring Initializr web tool or command-line interface to generate a new Spring Boot project with the necessary dependencies and configurations.
- Define Your Application’s Domain:
- Create domain classes (e.g., entities) to represent the data structure of your application.
- Use annotations like
@Entity
,@Table
, and@Column
for JPA-based applications.
- Create Repositories and Services:
- Implement data access logic using Spring Data JPA repositories.
- Create service classes to encapsulate business logic.
- Create Controllers:
- Build RESTful or web controllers to handle incoming HTTP requests.
- Use annotations like
@RestController
,@GetMapping
,@PostMapping
, etc., to define endpoints.
- Configure Application Properties:
- Define application-specific properties in
application.properties
orapplication.yml
files. - Configure database connection details, server ports, logging levels, etc.
- Test Your Application:
- Write unit tests and integration tests for your application’s components.
- Use testing frameworks like JUnit and Spring Test.
- Run Your Application:
- Start your Spring Boot application using the
java -jar
command or your IDE. - Access your application through a web browser or API testing tool.
- Extend Your Application:
- Add more features, services, and controllers as needed.
- Implement security, authentication, and authorization if required.
- Deploy Your Application:
- Deploy your Spring Boot application to a production environment. Options include cloud platforms, containerization (Docker), or traditional server hosting.
- Monitor and Maintain:
- Implement monitoring and management features using Spring Boot Actuator.
- Regularly maintain and update dependencies as needed.
Resources for Spring Boot Tutorials:
- Spring Boot Official Documentation:
- The official documentation is a comprehensive resource for learning about Spring Boot: https://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/
- Spring Guides:
- The Spring website provides a collection of guides that cover various Spring Boot topics: https://spring.io/guides
- Baeldung Spring Boot Tutorials:
- Baeldung offers a wide range of Spring Boot tutorials and articles: https://www.baeldung.com/spring-boot
- Spring Boot and Spring Data JPA Tutorial:
- A step-by-step tutorial on building a Spring Boot application with Spring Data JPA: https://www.callicoder.com/spring-boot-rest-api-tutorial-with-mysql-jpa-hibernate/
- Spring Boot and Spring Security Tutorial:
- Learn how to secure your Spring Boot application with Spring Security: https://www.baeldung.com/spring-security-login
- YouTube Tutorials:
- Search for Spring Boot tutorials on YouTube. Many instructors provide video tutorials covering various aspects of Spring Boot.
- Online Courses:
- Consider enrolling in online courses on platforms like Udemy, Coursera, or Pluralsight, which offer in-depth Spring Boot courses.
Remember that building a Spring Boot application is an iterative process, and you’ll likely encounter challenges along the way. The key is to practice and gradually increase your understanding of Spring Boot’s features and best practices.