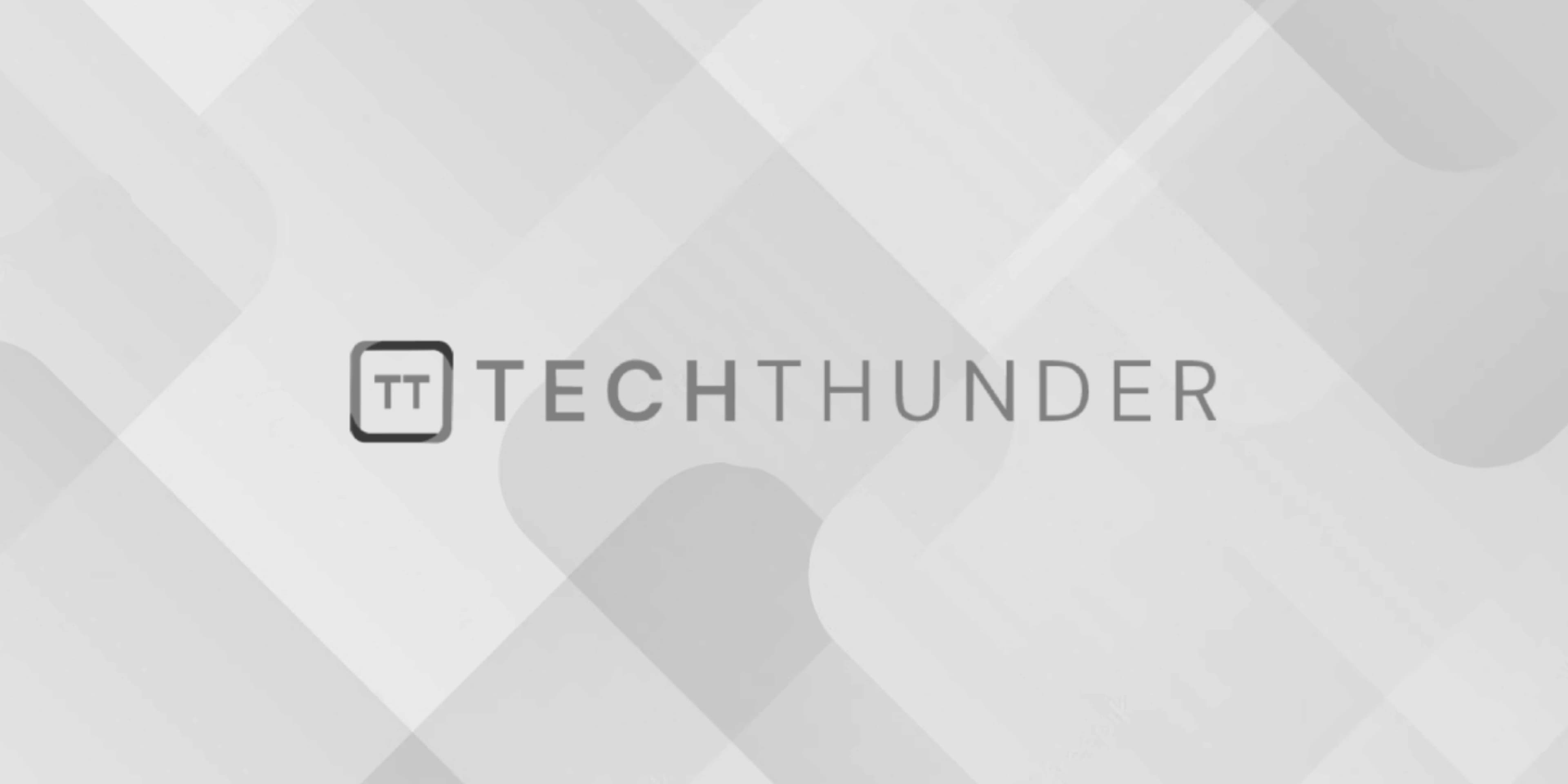
Implementing Exception Handling- 404 Resource Not Found
Implementing exception handling in a Spring Boot application is essential to provide meaningful error responses to clients. When a requested resource is not found (e.g., HTTP 404 – Resource Not Found), you should return a user-friendly error message to the client. Here’s how to implement exception handling for the “404 Resource Not Found” scenario:
Step 1: Create a Custom Exception Class:
First, create a custom exception class that extends the RuntimeException
or another appropriate exception class. This custom exception should capture the specific error message and any additional information you want to include in the error response. For a “Resource Not Found” scenario, you can create a ResourceNotFoundException
:
public class ResourceNotFoundException extends RuntimeException {
public ResourceNotFoundException(String message) {
super(message);
}
}
Step 2: Create a Custom Exception Handler:
Next, create a custom exception handler class that will handle the ResourceNotFoundException
and return a proper error response to the client. You can use the @ControllerAdvice
annotation to define a global exception handler for your application:
@ControllerAdvice
public class CustomExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFoundException(ResourceNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse(HttpStatus.NOT_FOUND.value(), ex.getMessage(), System.currentTimeMillis());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
// Add other exception handlers as needed
}
In the handleResourceNotFoundException
method:
- We use the
@ExceptionHandler
annotation to specify that this method handlesResourceNotFoundException
exceptions. - We create an
ErrorResponse
object to encapsulate the error details, including the HTTP status code, error message, and timestamp. - We return a
ResponseEntity
with the error response and an HTTP status ofNOT_FOUND
(404).
Step 3: Create an ErrorResponse Class:
Define an ErrorResponse
class to structure the error response in a consistent format:
public class ErrorResponse {
private int status;
private String message;
private long timestamp;
// Constructors, getters, and setters
}
Step 4: Throwing the Exception:
In your controller or service layer, when you encounter a “Resource Not Found” scenario, throw the ResourceNotFoundException
with a descriptive message:
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userRepository.findById(id)
.orElseThrow(() -> new ResourceNotFoundException("User not found with id: " + id));
return new ResponseEntity<>(user, HttpStatus.OK);
}
In this example, if the requested user with the specified ID is not found in the database, a ResourceNotFoundException
is thrown with the appropriate message.
Step 5: Test the Exception Handling:
Now, when a client sends a request for a non-existent resource (e.g., GET /users/123
where user with ID 123 does not exist), the custom exception handler will capture the exception and return a JSON error response with a 404 status code:
{
"status": 404,
"message": "User not found with id: 123",
"timestamp": 1634643867580
}
By implementing exception handling in this way, you can ensure that your Spring Boot application provides clear and consistent error responses to clients when resources are not found or when other exceptions occur.